Audiolib.js
How do I make an audiolib.js?
What is a audiolib.js? How do you make a audiolib.js? This script and codes were developed by Томаш Хамлай on 11 November 2022, Friday.
Audiolib.js - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>audiolib.js</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <style> html, body { height: 100%; } canvas { height: 100%; position: absolute; top: 0; right: 0; bottom: 0; left: 0; margin: auto; } .transculent { opacity: 0; } button { position: absolute; font-size: 3em; top: 50%; left: 50%; height: 25%; transform: translate(-50%, -50%); width: 50%; }
</style>
<script>
// Start off by initializing a new context.
context = new (window.AudioContext || window.webkitAudioContext)();
if (!context.createGain) context.createGain = context.createGainNode;
if (!context.createDelay) context.createDelay = context.createDelayNode;
if (!context.createScriptProcessor) context.createScriptProcessor = context.createJavaScriptNode;
// shim layer with setTimeout fallback
window.requestAnimFrame = (function(){
return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || window.oRequestAnimationFrame || window.msRequestAnimationFrame || function( callback ){ window.setTimeout(callback, 1000 / 60);
};
})();
function playSound(buffer, time) { var source = context.createBufferSource(); source.buffer = buffer; source.connect(context.destination); source[source.start ? 'start' : 'noteOn'](time);
}
function loadSounds(obj, soundMap, callback) { // Array-ify var names = []; var paths = []; for (var name in soundMap) { var path = soundMap[name]; names.push(name); paths.push(path); } bufferLoader = new BufferLoader(context, paths, function(bufferList) { for (var i = 0; i < bufferList.length; i++) { var buffer = bufferList[i]; var name = names[i]; obj[name] = buffer; } if (callback) { callback(); } }); bufferLoader.load();
}
function BufferLoader(context, urlList, callback) { this.context = context; this.urlList = urlList; this.onload = callback; this.bufferList = new Array(); this.loadCount = 0;
}
BufferLoader.prototype.loadBuffer = function(url, index) { // Load buffer asynchronously var request = new XMLHttpRequest(); request.open("GET", 'https://s3-us-west-2.amazonaws.com/s.cdpn.io/1715/the_xx_-_intro.mp3', true); request.responseType = "arraybuffer"; var loader = this; request.onload = function() { // Asynchronously decode the audio file data in request.response loader.context.decodeAudioData( request.response, function(buffer) { if (!buffer) { alert('error decoding file data: ' + url); return; } loader.bufferList[index] = buffer; if (++loader.loadCount == loader.urlList.length) loader.onload(loader.bufferList); }, function(error) { console.error('decodeAudioData error', error); } ); } request.onerror = function() { alert('BufferLoader: XHR error'); } request.send();
};
BufferLoader.prototype.load = function() { for (var i = 0; i < this.urlList.length; ++i) this.loadBuffer(this.urlList[i], i);
};
//Create an AudioContext instance for this sound
var audioContext = new (window.AudioContext || window.webkitAudioContext)();
// Create a buffer for the incoming sound content
var source = audioContext.createBufferSource();
// Create the XHR which will grab the audio contents
var request = new XMLHttpRequest();
// Set the audio file src here
request.open('GET', 'https://s3-us-west-2.amazonaws.com/s.cdpn.io/1715/the_xx_-_intro.mp3', true);
// Setting the responseType to arraybuffer sets up the audio decoding
request.responseType = 'arraybuffer';
request.onload = function() { // Decode the audio once the require is complete audioContext.decodeAudioData(request.response, function(buffer) { source.buffer = buffer; // Connect the audio to source (multiple audio buffers can be connected!) // source.connect(audioContext.destination); // Simple setting for the buffer source.loop = true; // Play the sound! source.start(0); }, function(e) { console.log('Audio error! ', e); });
}
// Send the request which kicks off
request.send();
var WIDTH = 640;
var HEIGHT = 360;
// Interesting parameters to tweak!
var SMOOTHING = 0.8;
var FFT_SIZE = 2048;
function VisualizerSample() { this.analyser = context.createAnalyser(); this.analyser.connect(context.destination); this.analyser.minDecibels = -140; this.analyser.maxDecibels = 0; loadSounds(this, { buffer: source.buffer }, onLoaded); this.freqs = new Uint8Array(this.analyser.frequencyBinCount); this.times = new Uint8Array(this.analyser.frequencyBinCount); function onLoaded() { var button = document.querySelector('button'); button.removeAttribute('disabled'); button.innerHTML = 'Play/pause'; }; this.isPlaying = false; this.startTime = 0; this.startOffset = 0;
}
// Toggle playback
VisualizerSample.prototype.togglePlayback = function() { if (this.isPlaying) { // Stop playback this.source[this.source.stop ? 'stop': 'noteOff'](0); this.startOffset += context.currentTime - this.startTime; console.log('paused at', this.startOffset); // Save the position of the play head. } else { this.startTime = context.currentTime; console.log('started at', this.startOffset); this.source = context.createBufferSource(); // Connect graph this.source.connect(this.analyser); this.source.buffer = this.buffer; this.source.loop = true; // Start playback, but make sure we stay in bound of the buffer. this.source[this.source.start ? 'start' : 'noteOn'](0, this.startOffset % this.buffer.duration); // Start visualizer. requestAnimFrame(this.draw.bind(this)); } this.isPlaying = !this.isPlaying;
}
VisualizerSample.prototype.draw = function() { this.analyser.smoothingTimeConstant = SMOOTHING; this.analyser.fftSize = FFT_SIZE; // Get the frequency data from the currently playing music this.analyser.getByteFrequencyData(this.freqs); this.analyser.getByteTimeDomainData(this.times); var width = Math.floor(1/this.freqs.length, 10); var canvas = document.querySelector('canvas'); var drawContext = canvas.getContext('2d'); canvas.width = WIDTH; canvas.height = HEIGHT; // // Draw the frequency domain chart. for (var i = 0; i < this.analyser.frequencyBinCount; i++) { var value = this.freqs[i]; var percent = value / 256; var height = HEIGHT * percent; var offset = HEIGHT - height - 1; var barWidth = WIDTH/this.analyser.frequencyBinCount; var hue = i/this.analyser.frequencyBinCount * 360; drawContext.fillStyle = 'hsl(' + hue + ', 100%, 50%)'; drawContext.fillRect(i * barWidth, offset, barWidth, height); } // Draw the time domain chart. for (var i = 0; i < this.analyser.frequencyBinCount; i++) { var value = this.times[i]; var percent = value / 256; var height = HEIGHT * percent * 2; var offset = HEIGHT - height/2 - 1; var barWidth = WIDTH/this.analyser.frequencyBinCount; drawContext.fillStyle = 'white'; drawContext.fillRect(i * barWidth, offset, 1, 2); } if (this.isPlaying) { requestAnimFrame(this.draw.bind(this)); }
}
</script>
<canvas style="width: 100%;"></canvas>
<button id="toggle" style="display: block" disabled="true">Please wait, loading...</button>
<script>
var sample = new VisualizerSample();
document.querySelector('button').addEventListener('click', function() { sample.togglePlayback(); console.log(this.className); if(this.className === '') { this.className += 'transculent'; } else { this.className = ''; }
});
</script>
</body>
</html>
Audiolib.js - Script Codes CSS Codes
canvas { background-color: #000;
}
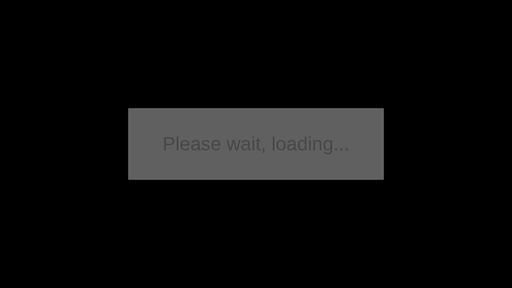
Developer | Томаш Хамлай |
Username | TomashKhamlai |
Uploaded | November 11, 2022 |
Rating | 3 |
Size | 4,063 Kb |
Views | 14,168 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Penalty Calculator | 5,771 Kb |
SVG Mascing Stretching AR rounded | 2,442 Kb |
Jplayer | 1,837 Kb |
Firefox SVG Example | 1,935 Kb |
Calc.js | 3,338 Kb |
SVG Clipping Stretching | 2,684 Kb |
Nesting countersEdit | 1,825 Kb |
Calc2 | 3,704 Kb |
SVG Clipping | 2,784 Kb |
SVG-button Responsive | 2,481 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Mosaic transition effect between two photos using jQuery | Stathisg | 2,518 Kb |
Multicolumns 2 | Raphaelgoetter | 1,857 Kb |
SVG Animation | Pollardld | 3,133 Kb |
A Pen by tugce | Ecgutcnkr | 4,197 Kb |
Ripples in water | Nobitagit | 2,704 Kb |
Mandelbrot Fractal | _Billy_Brown | 2,706 Kb |
A Pen by Mohomed Anees | Mohomedanees | 12,597 Kb |
About Mazano | Kiti | 2,585 Kb |
Day 1 - Portfolio | Chpecson | 3,532 Kb |
Pure CSS Read More Arrow | Zephyr | 1,747 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!