Cube of cubes
How do I make an cube of cubes?
Inspired by https://twitter.com/beesandbombs/status/887282988718936064. What is a cube of cubes? How do you make a cube of cubes? This script and codes were developed by Eli Fitch on 07 November 2022, Monday.
Cube of cubes - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Cube of cubes</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="container"></div> <script src='https://cdnjs.cloudflare.com/ajax/libs/three.js/r79/three.min.js'></script>
<script src='https://cdn.rawgit.com/mrdoob/three.js/dev/examples/js/controls/OrbitControls.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/gsap/1.20.2/TweenMax.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.4/lodash.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Cube of cubes - Script Codes CSS Codes
* { margin: 0; padding: 0;
}
.container { height: 100vh; width: 100%; position: relative;
}
canvas { position: absolute; top: 0; left: 0; height: 100%; width: 100%;
}
Cube of cubes - Script Codes JS Codes
'use strict';
//https://twitter.com/beesandbombs/status/887282988718936064
// Implementation:
//
var NUM_CUBES = 50;
// const CAMERA_DISTANCE = 18;
var CAMERA_DISTANCE = Math.min(window.innerWidth / 39, 18);
var boxPositions = [];
var boxes = [];
var processedAsOrigin = [];
var colors = { elephant: '#27393F', spray: '#7BE2FC', tangerine: '#FF977A', purple: '#D2A0E9', gold: '#FFD081', red: '#FF607D', blue: '#7EB6F8', green: '#C8EAA0'
};
var redMaterial = new THREE.MeshBasicMaterial({ color: colors.tangerine });
var blueMaterial = new THREE.MeshBasicMaterial({ color: colors.gold });
var greenMaterial = new THREE.MeshBasicMaterial({ color: colors.red });
var cubeMaterials = [redMaterial, redMaterial, blueMaterial, blueMaterial, greenMaterial, greenMaterial];
function composeScene(scene) { makeBoxStar({ x: 0, y: 0, z: 0 }, scene); makeBoxStar({ x: -1, y: 2, z: -1 }, scene); //top makeBoxStar({ x: 1, y: -2, z: 1 }, scene); //bottom makeBoxStar({ x: 1, y: 1, z: -2 }, scene); //top right makeBoxStar({ x: 2, y: -1, z: -1 }, scene); //bottom right makeBoxStar({ x: -2, y: 1, z: 1 }, scene); //top left makeBoxStar({ x: -1, y: -1, z: 2 }, scene); //bottom left makeBoxTweens(boxes);
}
function makeBoxStar(origin, scene) { scene.add(makeBox({ x: origin.x, y: origin.y, z: origin.z })); //middle scene.add(makeBox({ x: origin.x - 0.5, y: origin.y + 1, z: origin.z - 0.5 })); //top scene.add(makeBox({ x: origin.x + 0.5, y: origin.y - 1, z: origin.z + 0.5 })); //bottom scene.add(makeBox({ x: origin.x + 0.5, y: origin.y + 0.5, z: origin.z - 1 })); //top right scene.add(makeBox({ x: origin.x + 1, y: origin.y - 0.5, z: origin.z - 0.5 })); //bottom right scene.add(makeBox({ x: origin.x - 1, y: origin.y + 0.5, z: origin.z + 0.5 })); //top left scene.add(makeBox({ x: origin.x - 0.5, y: origin.y - 0.5, z: origin.z + 1 })); //bottom left
}
function makeBox(position, mat) { var posX = position.x; var posY = position.y; var posZ = position.z; if (!boxAlreadyThere({ x: posX, y: posY, z: posZ })) { var boxGeo = new THREE.BoxGeometry(1, 1, 1); // const boxMat = mat || new THREE.MeshNormalMaterial(); var boxMat = mat || new THREE.MeshFaceMaterial(cubeMaterials); var box = new THREE.Mesh(boxGeo, boxMat); box.position.set(posX, posY, posZ); boxPositions.push(position); boxes.push(box); return box; } return null;
}
function boxAlreadyThere(position) { for (var i = 0; i < boxPositions.length; i++) { if (_.isEqual(position, boxPositions[i])) { return true; } } return false;
}
function getMaxPosition(position) { var x = Math.abs(position.x); var y = Math.abs(position.y); var z = Math.abs(position.z); var result = x; if (result < y) { result = y; } if (result < z) { result = z; } return result;
}
function getDistanceFromOrigin(positionVector) { return positionVector.distanceTo(new THREE.Vector3(0, 0, 0));
}
function makeBoxTweens(boxes) { var dur = 1; var masterTL = new TimelineMax(); masterTL.pause(); for (var _iterator = boxes, _isArray = Array.isArray(_iterator), _i = 0, _iterator = _isArray ? _iterator : _iterator[Symbol.iterator]();;) { var _ref; if (_isArray) { if (_i >= _iterator.length) break; _ref = _iterator[_i++]; } else { _i = _iterator.next(); if (_i.done) break; _ref = _i.value; } var box = _ref; var delay = getDistanceFromOrigin(box.position) * 0.1; var tl = new TimelineMax(); tl.pause(); tl.to(box.scale, dur, { x: 0.75, y: 0.75, z: 0.75, ease: Power3.easeInOut }).to(box.rotation, dur, { x: 0, y: Math.PI / 2, z: 0, ease: Power3.easeInOut }).to(box.scale, dur, { x: 1, y: 1, z: 1, ease: Power3.easeInOut }).to(box.scale, dur, { x: 0.75, y: 0.75, z: 0.75, ease: Power3.easeInOut }).to(box.rotation, dur, { x: Math.PI / 2, y: Math.PI / 2, z: 0, ease: Power3.easeInOut }).to(box.scale, dur, { x: 1, y: 1, z: 1, ease: Power3.easeInOut }).to(box.scale, dur, { x: 0.75, y: 0.75, z: 0.75, ease: Power3.easeInOut }).to(box.rotation, dur, { x: Math.PI / 2, y: 0, z: 0, ease: Power3.easeInOut }).to(box.scale, dur, { x: 1, y: 1, z: 1, ease: Power3.easeInOut }).to(box.scale, dur, { x: 0.75, y: 0.75, z: 0.75, ease: Power3.easeInOut }).to(box.rotation, dur, { x: 0, y: 0, z: 0, ease: Power3.easeInOut }).to(box.scale, dur, { x: 1, y: 1, z: 1, ease: Power3.easeInOut }).repeat(-1).repeatDelay(0).delay(delay); tl.play(); }
}
//////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////
var renderer = undefined;
var camera = undefined;
var controls = undefined;
var scene = undefined;
function makeIsometricCamera() { // stolen from https://stackoverflow.com/questions/23450588/isometric-camera-with-three-js var aspect = window.innerWidth / window.innerHeight; var d = CAMERA_DISTANCE * 10; var w = window.innerWidth; var h = window.innerHeight; var cameraLeft = w / -d; var cameraRight = w / d; var cameraTop = h / d; var cameraBottom = h / -d; camera = new THREE.OrthographicCamera(cameraLeft, cameraRight, cameraTop, cameraBottom, 1, 100); // camera = new THREE.OrthographicCamera( - d * aspect, d * aspect, d, - d, 1, 1000 ); camera.position.set(CAMERA_DISTANCE, CAMERA_DISTANCE, CAMERA_DISTANCE); // all components equal camera.lookAt(scene.position); // or the origin
}
function init() { var container = document.getElementsByClassName('container')[0]; var w = container.offsetWidth; var h = container.offsetHeight; renderer = new THREE.WebGLRenderer({ antialias: true }); renderer.setSize(w, h); renderer.setClearColor(colors.elephant); renderer.setPixelRatio(window.devicePixelRatio); container.appendChild(renderer.domElement); scene = new THREE.Scene(); makeIsometricCamera(); controls = new THREE.OrbitControls(camera, renderer.domElement); controls.enableDamping = true; controls.dampingFactor = 0.2; controls.rotateSpeed = 0.3; controls.enableRotate = false; var hemilight = new THREE.HemisphereLight(0xFFFFFF, 0xFAFAFA, 2); scene.add(hemilight); scene.add(camera); composeScene(scene); render();
}
function render() { window.requestAnimationFrame(render); renderer.render(scene, camera); controls.update();
}
init();
window.addEventListener('resize', function () { // renderer.setSize(window.innerWidth, window.innerHeight); // camera.aspect = window.innerWidth / window.innerHeight; // camera.updateProjectionMatrix(); CAMERA_DISTANCE = Math.min(window.innerWidth / 39, 18); renderer.setSize(window.innerWidth, window.innerHeight); var w = window.innerWidth; var h = window.innerHeight; var d = CAMERA_DISTANCE * 10; camera.left = w / -d; camera.right = w / d; camera.top = h / d; camera.bottom = h / -d; camera.updateProjectionMatrix();
}, false);
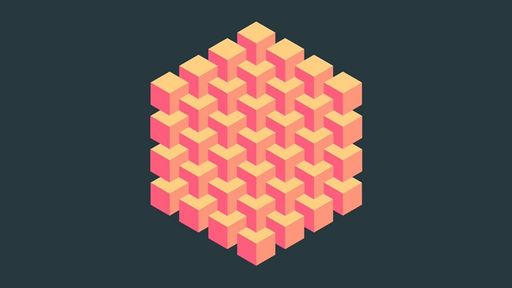
Developer | Eli Fitch |
Username | elifitch |
Uploaded | November 07, 2022 |
Rating | 4 |
Size | 6,112 Kb |
Views | 10,120 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Animated multi-line underline | 3,247 Kb |
Batman Middle Finger | 3,152 Kb |
SVG hamburger menu button | 2,602 Kb |
Animated SVG Hexagon | 3,470 Kb |
Diagonal gradient fill button | 4,860 Kb |
Smiley guy | 3,845 Kb |
3D Retro Wave Spin | 5,825 Kb |
Gradient Borders Mixin | 3,394 Kb |
Flexslider thumbnail modification | 13,431 Kb |
Slanty Button Mixin | 4,109 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Word Wrap Algorithm for Multiline Canvas Text | Peterhry | 2,349 Kb |
Airbnb Homepage | SindhujaD | 2,480 Kb |
Comment Jquery | SquishyAndroid | 2,421 Kb |
Sassy Buttons | Elyseholladay | 2,299 Kb |
Starfield using KineticJS | Asp | 3,512 Kb |
Background-blend-mode Test | 0x04 | 4,744 Kb |
Faux column absolute wrapper | Yurimorini | 1,823 Kb |
A Pen by Bryan Rojas | Bryanrojas | 1,873 Kb |
Funny menu | AxeLVaisper | 4,671 Kb |
Subtle site navigation with description | Necks | 3,206 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!