Flight Search Form
How do I make an flight search form?
What is a flight search form? How do you make a flight search form? This script and codes were developed by Sicontis on 12 July 2022, Tuesday.
Flight Search Form - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Flight Search Form</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="search-form"> <div id="header"> <h1>SEARCH FOR CHEAP FLIGHTS</h1> </div> <section> <div class="flight" id="flightbox"> <form id="flight-form"> <!-- TRIP TYPE --> <div id="flight-type"> <div class="info-box"> <input type="radio" name="flight-type" value="Return" id="return" checked /> <label for="return">RETURN</label> </div> <div class="info-box"> <input type="radio" name="flight-type" value="Single" id="one-way" /> <label for="one-way">ONE WAY</label> </div> </div> <!-- FROM/TO --> <div id="flight-depart"> <div class="info-box"> <label for="">LEAVING FROM</label> <input type="text" id="dep-from" /> <div id="depart-res"></div> </div> <div class="info-box" id="arrive-box"> <label for="">ARRIVING AT</label> <input type="text" id="dep-to" /> <div id="arrive-res"></div> </div> </div> <!-- FROM/TO --> <div id="flight-dates"> <div class="info-box"> <label for="">LEAVING ON</label> <input type="text" id="leave-date" readonly /> </div> <div class="info-box" id="return-box"> <label for="">RETURNING ON</label> <input type="text" id="return-date" readonly /> </div> </div> <!-- PASSENGER INFO --> <div id="flight-info"> <div class="info-box"> <label for="adults">ADULTS</label> <select name="adults" id="adults"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> </select> </div> <div class="info-box"> <label for="children">CHILDREN</label> <select name="children" id="children"> <option value="0">0</option> <option value="1">1</option> <option value="0">2</option> <option value="3">3</option> </select> </div> <div class="info-box"> <label for="class-type">CLASS</label> <select name="class-type" id="class-type"> <option value="Economy">ECONOMY</option> <option value="Business">BUSINESS</option> <option value="First">FIRST CLASS</option> </select> </div> </div> <!-- SEARCH BUTTON --> <div id="flight-search"> <div class="info-box"> <input type="submit" id="search-flight" value="SEARCH FLIGHTS"/> </div> </div> </form> </div> </section> <div id="calender"> <div class="nav"> <button id="prev"><</button> <p><span id="month"></span> <span id="year"></span></p> <button id="next">></button> </div> <table id="cal"></table> </div>
</div>
<div id="confirm"></div> <script src="js/index.js"></script>
</body>
</html>
Flight Search Form - Script Codes CSS Codes
/* Search Form */
* { box-sizing: border-box;
}
body { font-family: sans-serif; display: flex; flex-direction: column; justify-content: center; align-items: center;;
}
#search-form { border: 1px solid red; width: 700px; position: relative;
}
#header { height: 80px; background: red; display: flex; justify-content: flex-start; align-items: center;
}
#header h1 { color: #FFF; margin-left: 20px; font-size: 1.3em
}
.flight { padding: 20px;
}
.flight input, select { color: #6B6A6A;
}
.info-box { padding: 10px 0; margin-top: 20px; flex: 1; border-right: 20px solid transparent;
}
.info-box:last-child { border-right: none;
}
.info-box label { display: block; color: #6B6A6A; font-size: .9em;
}
.info-box input[type=text], input[type=submit], select { border: none; outline: none; background: #E2E0E0; margin-top: 5px; width: 100%; padding: 5px; height: 30px; border-radius: 3px;
}
#flight-type { display: flex; justify-content: flex-start; border-bottom: 1px dotted silver;
}
#flight-type .info-box { flex: 0 1 25%; display:flex; align-items: center;
}
#flight-depart { display: flex; justify-content: space-between; border-bottom: 1px dotted silver;
}
#flight-depart .info-box { position: relative;
}
#depart-res, #arrive-res { position: absolute; background: #FFFFFF;
}
#depart-res p, #arrive-res p { padding: 7px 2px; border-bottom: 1px dotted silver;
}
#depart-res p:hover, #arrive-res p:hover { background: #EDEDED; cursor: pointer;
}
#flight-dates { display: flex; border-bottom: 1px dotted silver;
}
#flight-info { display: flex; border-bottom: 1px dotted silver;
}
#flight-search .info-box { display: flex; justify-content: center;
}
#flight-search input[type=submit] { background: #55AF3A; color: white; padding: 0; height: 50px; font-size: 1.2em;
}
/* Calender */
#calender { border: 3px solid red; padding: 5px; opacity: 0; background: #FFFFFF; position: absolute; transition: opacity .3s; pointer-events: none;
}
#calender .nav { padding: 3px; display: flex; justify-content: space-between; align-items: center; margin-bottom: 5px;
}
#calender .nav button { background: #FF3240; border: none; outline: none; color: #FFFFFF; padding: 5px 8px; font-weight: 600; border-radius: 3px;
}
table { border: 1px solid #DBDBDB; background: #F4F4F4;
}
table td { border: 1px solid #DBDBDB; padding: 5px; text-align: center; cursor: pointer;
}
.normal { color: rgba(0,0,0,.8)
}
.today { background: #FF3240; color: #FFFFFF;
}
.closed { color: #DBDBDB; cursor: default;
}
.picked { background: green;
}
#month { display: inline-block;
}
#confirm { width: 500px; padding: 20px; border: 5px solid #55AF3A; margin: 20px auto; opacity: 0;
}
#confirm h3 { font-size: 1.2em; margin-bottom: 20px;
}
#confirm p { padding: 10px;
}
#confirm strong { font-weight: 600;
}
Flight Search Form - Script Codes JS Codes
/*jshint esversion: 6 */
let months = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
let dateObj = new Date();
let month = dateObj.getMonth();
let currMonth = dateObj.getMonth();
let year = dateObj.getFullYear();
let currYear = dateObj.getFullYear();
let tDate = dateObj;
let stayOpen = false;
let pickedDate = '';
let table = _('cal');
let cal = _('calender');
let xhr = new XMLHttpRequest();
let fromTo = 'from';
_('month').innerHTML = months[month];
_('year').innerHTML = year;
_('prev').addEventListener('click', () => trackMonth('prev'));
_('next').addEventListener('click', () => trackMonth('next'));
_('dep-from').addEventListener('keyup', searchCities);
_('dep-to').addEventListener('keyup', searchCities);
_('dep-from').addEventListener('focus', () => whichCityBox('from'));
_('dep-to').addEventListener('focus', () => whichCityBox('to'));
_('one-way').addEventListener('click', () => toggleReturnBox('one-way'));
_('return').addEventListener('click', () => toggleReturnBox('return'));
_('flight-form').addEventListener('submit', onSubmit);
_('leave-date').addEventListener('focus', showCalender);
_('leave-date').addEventListener('blur', hideCalender);
_('return-date').addEventListener('focus', showCalender);
_('return-date').addEventListener('blur', hideCalender);
function _(id) { return document.getElementById(id);
}
function addZero(d) { if (d < 10) return '0' + d; return d;
}
function convertDate(d) { var ts = new Date(d).toString(); ts = ts.substr(0, 15); console.log(ts); return ts;
}
function onSubmit(e) { e.preventDefault(); let form = this; let txt = '<h3>Search Form Results</h3>'; let returnTrip = form.elements[0]; let onewayTrip = form.elements[1]; let departFrom = form.elements[2].value; let arrivingAt = form.elements[3].value; let leavingOn = form.elements[4].value; let returningOn = form.elements[5].value; let adults = form.elements[6].value; let children = form.elements[7].value; let classType = form.elements[8].value; if (returnTrip.checked === true) txt += '<p>This is a <strong>Return</strong> trip.</p>'; if (onewayTrip.checked === true) txt += '<p>This is a <strong>One Way</strong> trip.</p>'; txt += '<p>Leaving on <strong>'+ convertDate(leavingOn) +'</strong> from <strong>'+ departFrom +'</strong>'; if(returningOn.length > 1) { txt += '<p>Returning on <strong>'+ convertDate(returningOn) +'</strong> from <strong>'+ arrivingAt +'</strong></p>'; } else { txt += ' and travelling to <strong>'+ arrivingAt + '</strong></p>'; } txt += '<p>Flying <strong>'+ classType +'</strong> class with <strong>'+ adults +'</strong> adults and <strong>'+ children +'</strong> children.</p>'; _('confirm').style.opacity = 1; _('confirm').innerHTML = txt; this.reset();
}
function toggleReturnBox(btn) { let box1 = _('return-box'); console.log(this.checked); if (btn == 'return') { box1.style.opacity = 1; } if (btn == 'one-way') { box1.style.opacity = 0; }
}
function whichCityBox(ft) { fromTo = ft;
}
function addFromCity(o) { _('dep-from').value = o.innerText; _('depart-res').style.display = 'none';
}
function addToCity(o) { _('dep-to').value = o.innerText; _('arrive-res').style.display = 'none';
}
function searchCities(e) { console.log(fromTo); let query = e.target.value; let url = 'https://maps.googleapis.com/maps/api/place/autocomplete/json?input=' + query + '&types=(cities)&key=AIzaSyA3sUMaNBtaRTxi8KLraNrYCVga4xK55WE'; let country = ''; let resp; xhr.open('GET', url); xhr.send(); xhr.onreadystatechange = function() { var DONE = 4; // readyState 4 means the request is done. var OK = 200; // status 200 is a successful return. if (xhr.readyState === DONE) { if (xhr.status === OK) resp = JSON.parse(xhr.responseText); var data = resp.predictions; for (var i = 0; i < data.length; i++) { if (fromTo == 'from') { country += '<p id="country" onclick="addFromCity(this)">' + data[i].description + '</p>'; } if (fromTo == 'to') { country += '<p id="country" onclick="addToCity(this)">' + data[i].description + '</p>'; } } } else { console.log('Error: ' + xhr.status); // An error occurred during the request. } if (fromTo == 'from') { _('depart-res').innerHTML = country; } if (fromTo == 'to') { _('arrive-res').innerHTML = country; } };
}
function positionCalender(box) { let boxPos = _('search-form').getBoundingClientRect(), leaveBoxPos = _('leave-date').getBoundingClientRect(), returnBoxPos = _('return-date').getBoundingClientRect(), leaveTopPos = leaveBoxPos.top - boxPos.top, leaveLeftPos = leaveBoxPos.left - boxPos.left, returnTopPos = returnBoxPos.top - boxPos.top, returnLeftPos = returnBoxPos.left - boxPos.left; if (box === 'leave-date') { cal.style.top = (leaveTopPos + 32) + 'px'; cal.style.left = (leaveLeftPos) + 'px'; } if (box === 'return-date') { cal.style.top = (returnTopPos + 32) + 'px'; cal.style.left = (returnLeftPos) + 'px'; }
}
function showCalender() { cal.style.opacity = 1; cal.style.pointerEvents = 'auto'; stayOpen = true; pickedDate = this.id; positionCalender(pickedDate);
}
function hideCalender() { if (!stayOpen) { cal.style.opacity = 0; cal.style.pointerEvents = 'none'; }
}
function getCellDate() { var tds = document.querySelectorAll('tbody td'), i; for (i = 0; i < tds.length; i++) { let btn = tds[i]; btn.addEventListener('click', fetchDate); }
}
function fetchDate() { let td = this.getAttribute('data-date'); if (pickedDate == 'leave-date') { _('leave-date').setAttribute('value', td); } if (pickedDate == 'return-date') { _('return-date').value = td; } stayOpen = false; hideCalender();
}
function trackMonth(dir) { if (dir == 'prev') month -= 1; if (dir == 'next') month += 1; if (month > 11) { month = 0; year += 1; } if (month < 0) { month = 11; year -= 1; } _('month').innerHTML = months[month]; _('year').innerHTML = year; calender(month, year);
}
function calender(month, year) { let today = dateObj.getDate(); let firstDay = new Date(year, month, 1); let startDay = firstDay.getDay(); let weekDays = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat']; var monthLength = new Date(year, month + 1, 0).getDate(); let html = ''; let dd; // DAYS OF WEEK HEADER html += '<tr>'; for (let i = 0; i < weekDays.length; i++) { html += '<td>' + weekDays[i] + '</td>'; } html += '</tr>'; // CALENDAR PART var count = 0; // count of table's <td> cells if (startDay !== 0) { // Leave these cells blank html += "<tr><td colspan='" + startDay + "'></td>"; count = startDay; } for (var day = 1; day <= monthLength; day++) { if (count % 7 === 0) { // new table row html += "<tr>"; } dd = addZero(year) + '/' + addZero(month + 1) + '/' + day; if (count < today && month == currMonth || year < currYear) { html += "<td class='closed' data-date=" + dd + ">" + day + "</td>"; } else { html += "<td class='normal' data-date=" + dd + ">" + day + "</td>"; } count++; if (count % 7 === 0) { html += "</tr>"; } } var blankCells = 7 - count % 7; if (blankCells < 7) { html += "<td colspan='" + blankCells + "'></td></tr>"; } table.innerHTML = html; getCellDate();
}
calender(month, year);
positionCalender('leave-date');
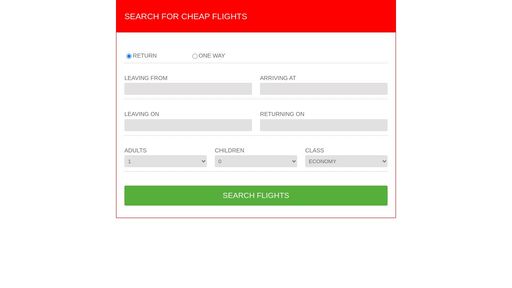
Developer | Sicontis |
Username | Sicontis |
Uploaded | July 12, 2022 |
Rating | 3 |
Size | 5,620 Kb |
Views | 127,512 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Sign Up Form | 5,272 Kb |
VueJS Reddit Component | 2,672 Kb |
Pomodoro Timer | 3,327 Kb |
That Reddit Guy | 2,140 Kb |
3D Flip Subscribe Button | 2,598 Kb |
Form Elements | 2,907 Kb |
Horizontal Bar Chart D3.js | 3,824 Kb |
Simple Day Timer | 2,549 Kb |
Vue JS Typeahead | 2,660 Kb |
Weather API Test | 3,032 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
SVG Text Masking | JMChristensen | 2,141 Kb |
JS Beispiel getElementsByClassName 3 | HSZG-Frontend-Kurs | 1,988 Kb |
Flat buttons for Eliassen.com | Kdooley89 | 1,737 Kb |
Animated Slide Hamburger Mobile Menu | BJack | 2,247 Kb |
Simple, flat contact form | Zeaklous | 2,719 Kb |
Calendar | Miroot | 2,033 Kb |
Magnus 3 | ARocketfish | 7,944 Kb |
A Pen by boilzzz | Boilzzz | 2,761 Kb |
A Pen by Matt Popovich | Mpopv | 3,349 Kb |
C.Rowe Button | Brownerd | 2,473 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!