Github Repo Breakdown
How do I make an github repo breakdown?
This pen breaks down a Github repo by language, and generates a graph based off the data. Just enter a username, and select one of that user's public repos to generate a pie chart based on the percentage (in bytes) represented by each language in the project.. What is a github repo breakdown? How do you make a github repo breakdown? This script and codes were developed by Jacob Poston on 27 December 2022, Tuesday.
Github Repo Breakdown - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Github Repo Breakdown</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="githubRepoViewer"> <link href="https://fonts.googleapis.com/css?family=Open+Sans:300,400,700" rel="stylesheet" type="text/css"> <div id="searchSection"> <div id="instructions"> <h1>Github Repo Analyzer</h1> <p>Enter a username in the top textbox and press "Load Users's Repos"</p> </br> <p>Then, select a repo from the dropdown and press "Generate Graph"</p> </div> <div id="searchMenu"> <input value="torvalds" type="text" id="userInput" /> <button onclick="setRepoSelect()">Load User's Repos</button> <select id="repoSelect"></select> <button onclick="setRepoGraph()">Generate Graph</button> </div> </div> <div id="graphSection"> <canvas id="graph"></canvas> <div id="graphLegend"></div> </div>
</div> <script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script>
<script src='https://rawgit.com/nnnick/Chart.js/master/Chart.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Github Repo Breakdown - Script Codes CSS Codes
@import url(https://fonts.googleapis.com/css?family=Source+Sans+Pro:200,300,400,600,700|Oswald:400,300,700);
* { margin: 0; padding: 0;
}
body{ background: #ddd;
}
#instructions { position: relative; margin-top: 30vh; margin-left: 15%; margin-bottom: 20px;
}
#instructions * { font: "Oswald"; letter-spacing: 0.5px; font-weight: 100;
}
#searchSection { display: inline-block; float: left; width: 40vw; height: 100vh;
}
#searchMenu input { width: 182px;
}
#searchMenu button:hover { background: #234;
}
#searchMenu button:active { background: #123; width: 190px;
}
#searchMenu > * { -webkit-transition: 0.1s all; text-align: center; display: block; margin: 5px auto; width: 200px; padding: 7px; border-radius: 5px; border: 2px solid #000; color: #777;
}
#graphSection { float: left; height: 100vh
}
#graph { margin: 50px; width: 400px; height: 400px;
}
#graphLegend { width: 500px;
}
.doughnut-legend { float: left; width: 200px; height: 100px; list-style: none; font-size: 14px; display: inline-block;
}
.doughnut-legend li * { display: inline-block; vertical-align: middle; margin: 0;
}
.doughnut-legend li { margin: 5px; display: block;
}
.doughnut-legend li div { font-size: 1em; width: 3em;
}
.doughnut-legend li span { width: 20px; height: 20px; border-radius: 5px; margin-right: 10px; margin-left: 10px;
}
Github Repo Breakdown - Script Codes JS Codes
var RATE_LIMIT_EXCEEDED = false;
var graphOptions = { segmentShowStroke: true, segmentStrokeColor: "#fff", segmentStrokeWidth: 0.5, percentageInnerCutout: 70, animationSteps: 100, animationEasing: "easeOutBounce", animateRotate: true, animateScale: false, animation: false, tooltipFillColor: "rgba(0,0,0,0.8)", tooltipTemplate: "<%if (label){%><%=label %>: <%}%><%= value + '%' %>", legendTemplate: "<ul class=\"<%=name.toLowerCase()%>-legend\"><% for (var i=0; i<segments.length; i++){%><li><div class=\"doughnut-value\"><%=segments[i].value%>%</div><span style=\"background-color:<%=segments[i].fillColor%>\"></span><p><%if(segments[i].label){%><%=segments[i].label%><%}%></p></li><%}%></ul>"
};
Object.size = function(obj) { var size = 0, key; for (key in obj) { if (obj.hasOwnProperty(key)) size++; } return size;
};
//If a color is entirely black, edit this function to fix the
//Github color name to match the JSON color map found at:
//https://raw.githubusercontent.com/doda/github-language-colors/master/colors.json
function getColorName(languageName, codeColors) { if (languageName == "C#") // This is what Github calls it languageName = "C Sharp"; // This needs to be what it matches in the JSON file if(languageName == "C++") languageName = "Objective-C++"; return codeColors[languageName] != null ? codeColors[languageName] : "#000";;
}
function getHighlightColor(color, percent) { var num = parseInt(color.slice(1), 16), amt = Math.round(2.55 * percent), R = (num >> 16) + amt, G = (num >> 8 & 0x00FF) + amt, B = (num & 0x0000FF) + amt; return "#" + (0x1000000 + (R < 255 ? R < 1 ? 0 : R : 255) * 0x10000 + (G < 255 ? G < 1 ? 0 : G : 255) * 0x100 + (B < 255 ? B < 1 ? 0 : B : 255)).toString(16).slice(1);
}
function convertDataToPercentage(data) { var sum = 0; data.forEach(function(dataPoint) { sum += dataPoint.value; }); data.forEach(function(dataPoint) { var percent = Math.round(10000 * (dataPoint.value / sum))/100; dataPoint.value = percent; }); return data;
}
function getRateLimit(callback) { var request = new XMLHttpRequest(); request.onload = function() { var response = JSON.parse(this.responseText); var remaining = response.resources["core"].remaining; var limit = response.resources["core"].limit; RATE_LIMIT_EXCEEDED = remaining < 1; console.log(RATE_LIMIT_EXCEEDED); var utcSeconds = response.resources["core"].reset; var d = new Date(0); d.setUTCSeconds(utcSeconds); if(RATE_LIMIT_EXCEEDED) alert('Sorry, but Github restricts unauthenticated api requests to only 60 per hour. \nYou have run out of requests, and will be allowed to request again at: \n' + d.toString()); }; request.open('get', 'https://api.github.com/rate_limit'); request.send()
}
function getRepos(username, callback) { if (!RATE_LIMIT_EXCEEDED) { var request = new XMLHttpRequest(); request.onload = callback; request.open('get', 'https://api.github.com/users/' + username + '/repos'); request.send() }
}
function getRepoStats(username, repoName, infoGet, callback) { if (!RATE_LIMIT_EXCEEDED) { var request = new XMLHttpRequest(); request.onload = callback; request.open('get', 'https://api.github.com/repos/' + username + '/' + repoName + '/' + infoGet, true); request.send() }
}
function createRepoGraph(username, repoName, canvasID) { if (!RATE_LIMIT_EXCEEDED) { repo = {}; $.getJSON("https://raw.githubusercontent.com/doda/github-language-colors/master/colors.json", function(codeColors) { getRepoStats(username, repoName, "languages", function() { repo.languages = JSON.parse(this.responseText); var data = []; for (var lang in repo.languages) { var dataColor = getColorName(lang, codeColors); data.push({ value: repo.languages[lang], color: dataColor, highlight: getHighlightColor(dataColor, 5), label: lang }); } data = convertDataToPercentage(data); var ctx = document.getElementById(canvasID).getContext("2d"); var languageGraph = new Chart(ctx).Doughnut(data, graphOptions); $("#graphLegend").empty(); $("#graphLegend").append(languageGraph.generateLegend()); }); }); } /*getRepoStats(username, repoName, "commits", function() { repo.commits = JSON.parse(this.responseText); });*/
}
function setRepoGraph() { var username = $("#userInput").val(); var repoName = $("#repoSelect").val(); console.log(username + " " + repoName); createRepoGraph(username, repoName, "graph");
}
function setRepoSelect() { if (!RATE_LIMIT_EXCEEDED) { $("#repoSelect").empty(); getRepos($("#userInput").val(), function() { var repos = JSON.parse(this.responseText); console.log(repos); for (var repo in repos) { if (repos[repo].name) { var repoOption = $('<option value="' + repos[repo].name + '">' + repos[repo].name + '</option>'); $("#repoSelect").append(repoOption); } } }); }
}
getRateLimit();
setRepoSelect();
setTimeout(setRepoGraph, 1000);
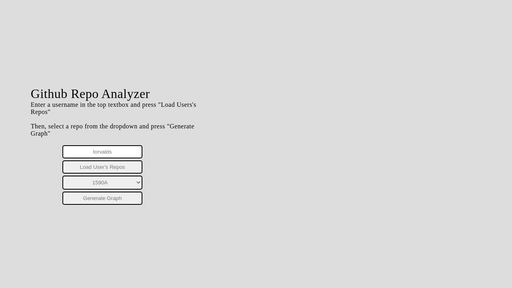
Developer | Jacob Poston |
Username | u1tralord |
Uploaded | December 27, 2022 |
Rating | 3 |
Size | 4,448 Kb |
Views | 8,096 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Simple Dialog | 2,504 Kb |
Custom Input Form | 2,587 Kb |
Database Form | 0 Kb |
A Pen by Jacob Poston | 3,103 Kb |
Timeline | 2,601 Kb |
My First CSS Animation | 1,879 Kb |
Basic Jumbotron with Layers | 1,867 Kb |
Tasker Interfaces | 1,740 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Count checked checkboxes with jQuery | Mestika | 2,343 Kb |
SVG Modified with Query String | Jnowland | 1,663 Kb |
SnappySnippet Test | Elmsoftware | 8,385 Kb |
Base-Style | Daniel_gooss | 2,614 Kb |
Pure CSS albums gallery | Renaudtertrais | 2,978 Kb |
Compare resources on mobile sites | Gyusza | 3,226 Kb |
Wave Lines | Mikehobizal | 4,023 Kb |
Blog Concept 2 | JGallardo | 2,994 Kb |
Pure CSS Read More Arrow | Zephyr | 1,747 Kb |
Learning canvas drawing | Aurer | 2,204 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!