JSON and Ajax demo part1
How do I make an json and ajax demo part1?
What is a json and ajax demo part1? How do you make a json and ajax demo part1? This script and codes were developed by DAWEI DAI on 29 September 2022, Thursday.
JSON and Ajax demo part1 - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>JSON and Ajax demo part1</title> <link rel='stylesheet prefetch' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="container-fluid"> <div class="row text-center"> <h2>Cat Photo Finder</h2> </div> <div class="row text-center"> <div class="col-xs-12 well message"> The message will go here </div> </div> <div class="row text-center"> <div class="col-xs-12"> <button id="getMessage" class="btn btn-primary"> Get Message </button> <button id="getCatJSON" class="btn btn-primary"> Get Gat JSON String </button> </div> </div> <div class="jumbotron"> <div class="row"> <div class="col-xs-12"> <h3 class="text-primary text-center">Lesson 1</h3> <p>Here the document ready is optional because codepen automatically puts the js right before the closing body tag</p> <h3 class="text-primary text-center">Lesson 2</h3> <pre>$("#getCatJSON").on("click", function() { $.getJSON("https://www.freecodecamp.com/json/cats.json", function(json) { $(".message").html(JSON.stringify(json)); });
});</pre> <pre>Technically should show: [
{
id: 0,
imageLink: "https://s3.amazonaws.com/freecodecamp/funny-cat.jpg",
codeNames: [
"Juggernaut",
"Mrs. Wallace",
"Buttercup"
]
},
{
id: 1,
imageLink: "https://s3.amazonaws.com/freecodecamp/grumpy-cat.jpg",
codeNames: [
"Oscar",
"Scrooge",
"Tyrion"
]
},
{
id: 2,
imageLink: "https://s3.amazonaws.com/freecodecamp/mischievous-cat.jpg",
codeNames: [
"The Doctor",
"Loki",
"Joker"
]
}
]</pre> <p><strong>JSON can be array format or object format</strong></p> <p>notice witrhout stringfy, not working, json variable contains Javscript Object</p> <p>If without jQuery, you use plain Ajax, then you need to do JSON.parse(xhr.reponseText)</p> <p>But here you are using jQuery + Ajax, json is ALREADY json object. JQuery parsed it for you automatically</p> <p>Still not working here because of same domain policy for prevent accessing a different domain from script - XMLHttpRequest cannot load https://www.freecodecamp.com/json/cats.json. No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'https://s.codepen.io' is therefore not allowed access.</p> <h3 class="text-primary text-center">Lesson 3</h3> <p>Now that we're getting data from a JSON API, let's display it in our HTML. We can use the .forEach() method to loop through our data and modify our HTML elements. First, let's declare an html variable with var html = "";. Then, let's loop through our JSON, adding more HTML to that variable. When the loop is finished, we'll render it. </p> <pre>Here's the code that does this:
json.forEach(function(val) { var keys = Object.keys(val); html += "<div class = 'cat'>"; keys.forEach(function(key) { html += "<b>" + key + "</b>: " + val[key] + "<br>"; }); html += "</div><br>";
});
</pre> <pre>[
{
id: 0,
imageLink: "https://s3.amazonaws.com/freecodecamp/funny-cat.jpg",
codeNames: [
"Juggernaut",
"Mrs. Wallace",
"Buttercup"
]
},
{
id: 1,
imageLink: "https://s3.amazonaws.com/freecodecamp/grumpy-cat.jpg",
codeNames: []
},
{
id: 2,
imageLink: "https://s3.amazonaws.com/freecodecamp/mischievous-cat.jpg",
codeNames: [
"The Doctor",
"Loki",
"Joker"
]
}
]</pre> <h3 class="text-primary text-center">Lesson 4</h3> <p>We've seen from the last two lessons that each object in our JSON array contains an imageLink key with a value that is the url of a cat's image. When we're looping through these objects, let's use this imageLink property to display this image in an img element.</p> <pre><script> $(document).ready(function() { $("#getMessage").on("click", function() {
  $.getJSON("/json/cats.json", function(json) { var html = ""; json.forEach(function(val) { html += "<div class = 'cat'>"; // Only change code below this line. html += "<img src = '" + val.imageLink + "'>"; // Only change code above this line. html += "</div>"; });
     $(".message").html(html); }); }); });
</script></pre> <h3 class="text-primary text-center">Lesson 5</h3> <p> If we don't want to render every cat photo we get from our Free Code Camp's Cat Photo JSON API, we can pre-filter the json before we loop through it. Let's filter out the cat whose "id" key has a value of 1. Here's the code to do this:</p> <pre>json = json.filter(function(val) { return (val.id !== 1);
});</pre> <h3 class="text-primary text-center">Lesson 6</h3> <p>Get Geolocation Data Another cool thing we can do is access our user's current location. Every browser has a built in navigator that can give us this information. The navigator will get our user's current longitude and latitude. You will see a prompt to allow or block this site from knowing your current location. The challenge can be completed either way, as long as the code is correct. By selecting allow you will see the text on the output phone change to your latitude and longitude Here's some code that does this:</p> <pre><script> // Only change code below this line. if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function(position) { $("#data").html("latitude: " + position.coords.latitude + "<br>longitude: " + position.coords.longitude); });
} // Only change code above this line.
</script>
<div id = "data"> <h4>You are here:</h4>
</div>
</pre> <div id = "data"> <h4>You are here:</h4>
</div> </div> </div> </div>
</div> <script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.2/jquery.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
JSON and Ajax demo part1 - Script Codes CSS Codes
body{ margin-top: 60px;
}
JSON and Ajax demo part1 - Script Codes JS Codes
$(document).ready(function() { $("#getMessage").on("click", function() { $(".message").html("Here is the message"); }); // end of first onClick assaignment if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function(position) { $("#data").html("latitude: " + position.coords.latitude + "<br>longitude: " + position.coords.longitude); }); } });
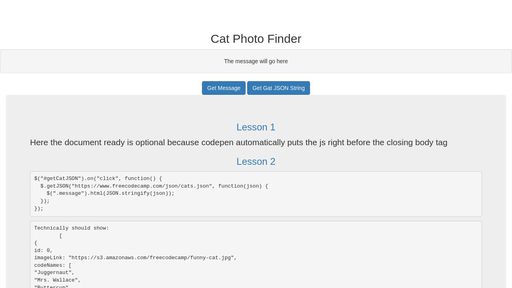
Developer | DAWEI DAI |
Username | shachopin |
Uploaded | September 29, 2022 |
Rating | 3 |
Size | 3,828 Kb |
Views | 28,336 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
RequireJS consolidation part1 | 6,672 Kb |
Oracle JET consolidation part3 | 10,230 Kb |
Java consolidation part1.1 - Collections 1 | 12,731 Kb |
Oracle JET Experiment 1 - ojMenu and delayed Ajax and launch Popup | 5,635 Kb |
Oracle JET Experiment 5 - Toolbar | 3,377 Kb |
Rails consolidation part5 - Bootstrap and Asset Pipeline | 10,926 Kb |
Rails consolidation part14 - Rails app with Oracle JET and knockout js | 1,957 Kb |
ADF consolidation part1 | 5,420 Kb |
Rails consolidation part6 - Sample Code Snippets_A | 10,663 Kb |
Oracle JET Experiment 3 - Offcanvas | 4,424 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Very Simple Slider | Doodlemarks | 2,682 Kb |
Count up | Alanshortis | 2,391 Kb |
After America | Jonathangarner | 2,686 Kb |
A Pen by Anoop | Anoopjohn | 330,760 Kb |
JQuery AJAX reddit Exercise | Btholt | 1,777 Kb |
Realistic Buttons | Stoypenny | 2,248 Kb |
Background-blend-mode Test | 0x04 | 4,744 Kb |
CSS3 keyframe onload animation. | Samueljseay | 1,706 Kb |
Svg sky | Omodev | 7,070 Kb |
Rotate Demo | Agelber | 3,061 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!