Math using Stacks
How do I make an math using stacks?
What is a math using stacks? How do you make a math using stacks? This script and codes were developed by Ajala Comfort on 11 January 2023, Wednesday.
Math using Stacks - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Math using Stacks </title> <link rel='stylesheet prefetch' href='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body>
<body></body> <script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.3.1/react.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.3.1/react-dom.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.0/jquery.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Math using Stacks - Script Codes CSS Codes
body > div > div{width:70%;margin-top:200px;margin-left:15%}
body > div > div .form-control{width:70%;display:inline-block;text-align:center;}
body > div > div button{width:30%;display:inline-block;}
body > div > div p{width:100%;text-align:center;color:gray;}
body > div > div p span{font-weight:bolder;color:black;}
Math using Stacks - Script Codes JS Codes
"use strict";
function processExp(ex) { return ex.split(" ").join("").split("");
}
//console.log(("5*1235 * 2+13436 /2").split(/[*\+\-\/]/g))
function List() { this.data = []; this.add = function (val) { return val === '(' || val === ')' ? null : this.data.push(val); }; this.process = function (exp) { return this.data = processExp(exp); }; this.removeLast = function () { return this.data.shift(); }; this.isEmpty = function () { return this.data.length <= 0; }; this.toString = function () { return this.data.join(""); };
}
function Stack() { this.data = []; this.top = 0; this.pop = function () { this.top--;return this.data.pop(); }; this.push = function (val) { this.data.push(val);return this.top++; }; this.peek = function () { return this.data[this.top - 1]; }; this.isEmpty = function () { return this.data.length <= 0; }; this.length = function () { return this.data.length; }; this.toString = function () { return this.data.join(""); };
}
function precedence(a) { if (a === '+' || a === '-') { return 3; } return 2;
}
function preFix(ex) { var input = new List(), output = new List(), leftBrackPos = new Stack(), operator = new Stack(); input.process(ex);var i = 0; function solve() { var value = input.data[0]; //returns the first element of the input array //check if num or not if (isNaN(value)) { //not number if (value === '(') { //add intothe operator stack and update the left brack stack operator.push(value);leftBrackPos.push(operator.length() - 1); } else if (value === ')') { //pop the first var lastLeftBracket = leftBrackPos.pop(); //returns the last add postion of the closest left bracket while (operator.top - 1 >= lastLeftBracket) { //loops while the top posi is above the pos of he closest bracket//add to the output; pop from operator output.add(operator.pop()); } } else { //check if the leftbrack stack is empty if (leftBrackPos.isEmpty() === false) { //if the leftbrack stack is not empty just update the operator stack operator.push(value); } //check if there is a value in operator to compare with else if (operator.peek() !== undefined) { ///if there is a value compare var pre = precedence(operator.peek()) <= precedence(value); if (pre) { //if the top value in operator stack has lower or similar precendence than the incoming operator --> remove the top operator and push to the output and update the operator stack output.add(operator.pop());operator.push(value); } else { //if greater than just update the operator stack operator.push(value); } } else { //if there are no operators update stack operator.push(value); } } } else { output.add(value); } //remove the value from input input.removeLast(); //check if the input is empty i++; if (input.isEmpty()) { //if empty --> done; check if the leftbrackidempty // console.log("DONE") if (leftBrackPos.isEmpty()) { //if empty push all operators into the outpur while (!operator.isEmpty()) { output.add(operator.pop()); } } else { return "INVALID EXPRESSION"; } return output.data; } else { //if not empty;repeat the solve return solve(); } } return solve();
}
function myMath(a, b, sign) { if (a === undefined || b === undefined) { return undefined; } a = parseInt(a);b = parseInt(b); var ans; switch (sign) { case '+': ans = a + b;break; case '-': ans = a - b;break; case '/': ans = a / b;break; case '*': ans = a * b;break; } return ans;
}
function doMath(input) { if (!Array.isArray(input)) { return { answer: "Invalid Expression", postfix: "Unable to Process Expression" }; } var i = 0, arithmetic = new Stack(), ans = 0; var post = input.join(""); while (ans !== undefined && i < input.length) { if (isNaN(input[i])) { //not a number pop to values var a = arithmetic.data.pop(), b = arithmetic.data.pop(); ans = myMath(b, a, input[i]); if (ans !== undefined) { arithmetic.data.push(ans); } } else { arithmetic.data.push(input[i]); } i++; } ans = ans === undefined ? "INVALID EXPRESSION" : ans; return { answer: ans, postfix: post };
}
var Maths = React.createClass({ displayName: "Maths", getInitialState: function getInitialState() { var exp = "1 + 2 + (3 * 5)"; return { expression: exp, postfix: doMath(preFix(exp)) }; }, updateExpression: function updateExpression(e) { return this.setState({ expression: e.target.value }); }, solve: function solve() { return this.setState({ postfix: doMath(preFix(this.state.expression)) }); }, render: function render() { return React.createElement( "div", null, React.createElement( "div", null, React.createElement( "div", { className: "alert alert-success", role: "alert" }, "Currently works for numbers below 10 Sawwie! :)" ), React.createElement("input", { type: "text", id: "expression", className: "form-control", placeholder: this.state.expression, onChange: this.updateExpression }), React.createElement( "button", { className: "btn btn-success", onClick: this.solve }, "Solve" ), React.createElement( "p", { id: "answer" }, "Answer ", React.createElement( "span", null, this.state.postfix.answer ) ), React.createElement( "p", { id: "postfix" }, "PostFix --> ", React.createElement( "span", null, this.state.postfix.postfix ) ) ) ); }
});
ReactDOM.render(React.createElement(Maths, null), document.body);
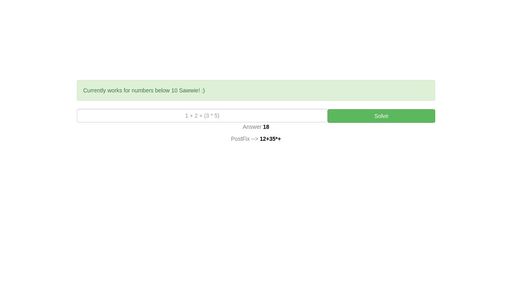
Developer | Ajala Comfort |
Username | AJALACOMFORT |
Uploaded | January 11, 2023 |
Rating | 3 |
Size | 5,686 Kb |
Views | 4,048 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Material Chat app Interface with ReactJS | 5,851 Kb |
Basic ReactJs Form | 5,214 Kb |
Recipe Book | 4,813 Kb |
Palindrome Word | 3,421 Kb |
Hashtables | 1,836 Kb |
Bill Splitting App | 6,825 Kb |
Intermediate Todo List with React.js | 6,191 Kb |
My Porfolio | 5,245 Kb |
Slide Show with ReactJs | 3,672 Kb |
SVG Clock Practice | 2,436 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Another brick in the wall | Fivera | 1,955 Kb |
About Us | Francescaedits | 1,902 Kb |
Layout 11 | Altynai | 1,690 Kb |
Basecamp 3 Document | Lachlanjc | 3,811 Kb |
Simple search using AngularJS | Haykou | 1,802 Kb |
Testing Portfolio Page | Sideshowli | 3,395 Kb |
IE11 Test | Boostnewmedia | 4,998 Kb |
Drag in vanilla js using dotval math instead of translate | Paulq | 2,662 Kb |
Halo 5 REQ Guide Bookmarklet | Cwacht | 3,993 Kb |
The Fly | GianlucaGuarini | 3,405 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!