MiniMarkdown.JS
How do I make an minimarkdown.js?
What is a minimarkdown.js? How do you make a minimarkdown.js? This script and codes were developed by Nicolas Udy on 10 August 2022, Wednesday.
MiniMarkdown.JS - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>MiniMarkdown.JS</title> <link rel='stylesheet prefetch' href='css/wxxzbj.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <main class="page"> <aside class="section section--fixed"> <section class="editor"> <fieldset class="editor__field"> <label for="title">Title</label> <input class="editor__input js-editor-title" id="title" type="text" value="miniMarkdown.JS"/> </fieldset> <div class="editor__content"> <textarea class="editor__textarea js-editor-content">#Basic markdown features with live update
[Here's a link to this pen](http://codepen.io/udyux/pen/qqXLrL?editors=0010) as an example.
The JavaScript that takes care of the live update is written in ES2015 and converted using [Babel](https://babeljs.io/), although it will work natively in the most recent versions of all major browsers without conversion.
You can set the font to be _italic_ or *bold*. You can even set it it to have *_both_*, regardless of which style queue comes _*first*_ or which *_order*_ they're in (check the markup).
#Put subtitles anywhere
Check out the comments at the *top* of the JavaScript pane see how you can style text input.
#Clean content
Multiple newlines are converted to a single newline and then wrapped in P tags.
To keep things safe in this pen, *all html tags are removed* from the input. You _could_ remove the replace found at *_19_*.
#Next up
I'm planning on adding
- ~lists~
- more headings
- tabulation
- ~quotes~
- ~strikethrough~
- code excerpts
in the near future.
Hope this comes in handy, enjoy!
*_UdyUX_*</textarea> </div> </section> </aside> <aside class="section"> <article class="article"> <h1 class="article__title js-article-title"></h1> <div class="article__content js-article-content"></div> </article> </aside>
</main> <script src="js/index.js"></script>
</body>
</html>
MiniMarkdown.JS - Script Codes CSS Codes
.page { position: relative; color: #4c4c4c; font-weight: 200;
}
.section { position: absolute; top: 0; left: 50%; width: 50%; padding: 2.5vw 5vw;
}
.section--fixed { position: fixed; left: 0; height: 100vh; background-color: #fafafa;
}
.section + .section { border-left: 1px solid #c4c4c4;
}
.editor { display: -webkit-box; display: -ms-flexbox; display: flex; -webkit-box-orient: vertical; -webkit-box-direction: normal; -ms-flex-direction: column; flex-direction: column; height: 100%;
}
.editor__input { border: 1px solid #e0e0e0; width: 350px; padding: .5rem 1rem; margin-left: 1rem; font-size: 1.1rem; font-weight: 600; background-color: white; color: inherit;
}
.editor__content { position: relative; -webkit-box-flex: 1; -ms-flex-positive: 1; flex-grow: 1; margin-top: 1.5rem;
}
.editor__textarea { position: absolute; top: 0; left: 0; width: 100%; height: 100%; border: 1px solid #e0e0e0; background-color: white; font-size: .95rem; padding: 1rem; font-weight: 200; font-family: inherit;
}
.article { border: .5rem solid #8c8c8c; padding: 2rem;
}
.article__title { position: relative; margin-bottom: 2rem; padding-bottom: .5rem; font-size: 2.75rem; font-weight: 600;
}
.article__title::after { content: ''; position: absolute; bottom: 0; left: 0; width: 100%; height: .25rem; background-color: #8c8c8c;
}
.article__content { font-size: 1rem; font-weight: 200; line-height: 1.5;
}
.mmd--p + .mmd--p { margin-top: 1rem;
}
.mmd--h2 { margin: 2rem 0 1rem; font-size: 1.5rem; font-weight: 900;
}
.mmd--b { font-weight: 600;
}
.mmd--i { font-style: italic;
}
.mmd--a { color: #456990; font-weight: 300; text-decoration: underline;
}
.mmd--s { text-decoration: line-through;
}
.mmd--code { white-space: pre; padding: 1rem; background-color: #e0e0e0; font-family: monospace;
}
.mmd--li { position: relative; margin-left: 3rem; margin-top: 1.5rem;
}
.mmd--li::before { content: ''; position: absolute; top: 0; right: 100%; -webkit-transform: translate(-0.75rem, 0.5rem); transform: translate(-0.75rem, 0.5rem); width: .4rem; height: .4rem; border-radius: 100%; background-color: #8c8c8c;
}
.mmd--q { position: relative; margin-left: 3rem; margin-top: 1.5rem;
}
.mmd--q::before { content: ''; position: absolute; top: 0; right: 100%; -webkit-transform: translateX(-0.75rem); transform: translateX(-0.75rem); width: .4rem; height: 100%; border-radius: .4rem; background-color: #8c8c8c;
}
.mmd--q + .mmd--q, .mmd--li + .mmd--q, .mmd--q + .mmd--li, .mmd--li + .mmd--li { margin-top: .75rem;
}
.mmd--q + .mmd--p, .mmd--li + .mmd--p { margin-top: 1.5rem;
}
.mmd--q .mmd--br, .mmd--li .mmd--br { display: block; height: .5rem;
}
MiniMarkdown.JS - Script Codes JS Codes
'use strict';
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
/*----------------- #subtitle [label](link) _italic_ *bold* *_bold italic_* - list item > quoted
------------------*/
var Format = function () { function Format() { _classCallCheck(this, Format); } Format.init = function init() { if (!String.replaceMultiple) { String.prototype.replaceMultiple = function () { var _string = this; for (var _len = arguments.length, regexArray = Array(_len), _key = 0; _key < _len; _key++) { regexArray[_key] = arguments[_key]; } regexArray.forEach(function (_ref) { var regex = _ref[0]; var repl = _ref[1]; _string = _string.replace(regex, repl); }); return _string; }; String.prototype.escapeMarkdown = function () { return this.replace(/\\\*_/g, '*_').replace(/\\_\*/g, '_*').replace(/\\\*/g, '*').replace(/\\_/g, '_').replace(/\\`/g, '`').replace(/\\-/g, '‐').replace(/\\#/g, '#').replace(/\\~/g, '∼').replace(/\\>/g, '>'); }; String.prototype.sanitize = function () { return this.replace(/<[^>]+>/g, ''); }; } Format.regex = { trailingWhitespace: [/\s+\n/g, '\n'], repeatNewlines: [/\n{2,}/g, ''], lists: [/^- +(.+)((\n(?=\s{2,}).+)*)/gm, '<p class="mmd--li">$1$2</p>'], quotes: [/^> +(.+)((\n(?=\s{2,}).+)*)/gm, '<p class="mmd--q">$1$2</p>'], lineBreaks: [/(^ {2,}[^\n]+|^<p.+-li[^\n]+|^<p.+-q[^\n]+)*\n\s{2,}/g, '<br><span class="mmd--br"></span>'], code: [/^`{3}\n([^`]+)^`{3}/gm, '<p class="mmd--code">$1</p>'], links: [/\[(.+)\]\((\S+)\)/g, '<a class="mmd--a" href="$2" target="_blank">$1</a>'], boldItalic: [/(?:\*_|_\*)(?!\s)([^*_]+\S)(?:\*_|_\*)/g, '<span class="mmd--b mmd--i">$1</span>'], bold: [/\*(\S[^*]+\S)\*/g, '<span class="mmd--b">$1</span>'], italic: [/\_(\S[^_]+\S)\_/g, '<span class="mmd--i">$1</span>'], strikethrough: [/~(\S[^~]+\S)~/g, '<span class="mmd--s">$1</span>'] }; }; Format.markdown = function markdown(str) { return str.sanitize().escapeMarkdown().replaceMultiple(Format.regex.trailingWhitespace, Format.regex.repeatNewlines, Format.regex.code, Format.regex.lists, Format.regex.quotes, Format.regex.lineBreaks).split('\n').map(function (line) { return !line.length ? null : Format.line(line); }).join(''); }; Format.line = function line(str) { var _line = str.replaceMultiple(Format.regex.links, Format.regex.boldItalic, Format.regex.bold, Format.regex.italic, Format.regex.strikethrough); switch (_line[0]) { case '#': // output subtitle return _line.replace(/#/, '<h2 class="mmd--h2">') + '</h2>'; case '<': // keep tags return _line[1] !== 'p' ? '<p class="mmd--p">' + _line + '</p>' : _line; case ' ': return _line.substring(0, 3) !== ' ' ? '<p class="mmd--p">' + _line + '</p>' : _line; default: // ouput paragraph return '<p class="mmd--p">' + _line + '</p>'; } }; Format.field = function field(str) { return str.sanitize(); }; return Format;
}();
var Editor = function () { function Editor() { var _this = this; _classCallCheck(this, Editor); Format.init(); for (var _len2 = arguments.length, nodes = Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { nodes[_key2] = arguments[_key2]; } nodes.forEach(function (_ref2) { var input = _ref2[0]; var output = _ref2[1]; _this.bindNodes(input, output, ~input.tagName.toLowerCase().indexOf('textarea')); }); } Editor.prototype.bindNodes = function bindNodes(input, output, bindMarkdown) { var formatType = bindMarkdown ? Format.markdown : Format.field; var update = function update() { output.innerHTML = formatType(input.value); }; input.addEventListener('keyup', update); update(); }; return Editor;
}();
new Editor([document.querySelector('.js-editor-title'), document.querySelector('.js-article-title')], [document.querySelector('.js-editor-content'), document.querySelector('.js-article-content')]);
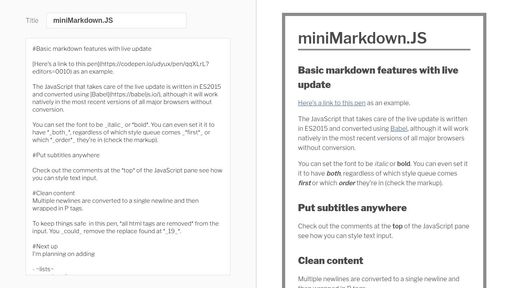
Developer | Nicolas Udy |
Username | udyux |
Uploaded | August 10, 2022 |
Rating | 4 |
Size | 7,647 Kb |
Views | 38,456 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Hue rotate | 5,617 Kb |
Transforming hover buttons | 4,614 Kb |
Cursor based dynamic perspective logo | 4,228 Kb |
CSS Kiwi | 4,883 Kb |
SVG Analog Clock | 5,821 Kb |
Reset | 4,318 Kb |
Simple Stopwatch | 4,799 Kb |
Automatic SVG Pie-Charts | 4,466 Kb |
Snipcart CSS Animations | 18,476 Kb |
Catching panels on scroll | 5,530 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
ECharts Version 3.0 - Bar Marker Chart | WebCodePro | 2,726 Kb |
Slim Grid SASS SCSS v3.2 | Thesturs | 4,709 Kb |
Siema - add pagination to prototype | Pawelgrzybek | 2,575 Kb |
Background Images | Jooonebug | 2,100 Kb |
My Starter Kit For Codepen | Dkdesign | 2,012 Kb |
Stylize Stories | Jvhti | 2,465 Kb |
Expert Help | SinceSidSlid | 4,064 Kb |
Animated bar chart | CreativePunch | 3,124 Kb |
Loading animation with css | Icebob | 2,947 Kb |
About Us | Francescaedits | 1,902 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!