Particle Text
How do I make an particle text?
In my Pen Laser Writer I did not care about reading the pre-rendered text's color value of each pixel - it was just a matter of pixel or no pixel. I stored the x and y coordinates in a list.. What is a particle text? How do you make a particle text? This script and codes were developed by Johan Karlsson on 26 July 2022, Tuesday.
Particle Text - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Particle Text</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="box"> <canvas id="canvas"></canvas>
</div>
<a href="http://codepen.io/collection/nWQJKO" class="lowerRight" target="_blank">Show me more!</a> <script src='https://ajax.googleapis.com/ajax/libs/webfont/1.6.16/webfont.js'></script> <script src="js/index.js"></script>
</body>
</html>
Particle Text - Script Codes CSS Codes
body { text-align: center; background-color: #dddde0;
}
.lowerRight { margin: 2px; font-family: Ubuntu; font-size: large; color: #00002F; position: fixed; right: 0; bottom: 0;
}
/* Paper like CSS shadows from: http://www.sitepoint.com/pure-css3-paper-curls/ By Craig Buckler
*/
.box { display: inline-block; position: relative; margin: 30px; background-color: #fafaff; box-shadow: 0 0 5px rgba(0, 0, 0, 0.2), inset 0 0 50px rgba(0, 0, 0, 0.1);
}
.box:before, .box:after { position: absolute; width: 30%; height: 10px; content: ' '; left: 12px; bottom: 12px; background: transparent; -webkit-transform: skew(-5deg) rotate(-5deg); transform: skew(-5deg) rotate(-5deg); box-shadow: 0 6px 12px rgba(0, 0, 0, 0.3); z-index: -1;
}
.box:after { left: auto; right: 12px; -webkit-transform: skew(5deg) rotate(5deg); transform: skew(5deg) rotate(5deg);
}
Particle Text - Script Codes JS Codes
"use strict";
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
/* Particle Text Johan Karlsson 2016-04-03
*/
var settings = { startDelay: 60, duration: 300, text: "Particles!", textSize: 180, //easeInOutCubic, easeOutCubic, easeOutBack or easeOutBounce easing: "easeInOutCubic"
};
var Particle = function () { function Particle(destX, destY, x, y, color) { _classCallCheck(this, Particle); this.destX = destX; this.destY = destY; this.x = x; this.y = y; this.startX = x; this.startY = y; this.color = color; } // Based on Robert Penner's easing functions Particle.prototype.easeInOutCubic = function easeInOutCubic(t, b, c, d) { if ((t /= d / 2) < 1) return c / 2 * t * t * t + b; return c / 2 * ((t -= 2) * t * t + 2) + b; }; Particle.prototype.easeOutCubic = function easeOutCubic(t, b, c, d) { return c * ((t = t / d - 1) * t * t + 1) + b; }; Particle.prototype.easeOutBack = function easeOutBack(t, b, c, d) { var s = 1.70158; return c * ((t = t / d - 1) * t * ((s + 1) * t + s) + 1) + b; }; Particle.prototype.easeOutBounce = function easeOutBounce(t, b, c, d) { if ((t /= d) < 1 / 2.75) { return c * (7.5625 * t * t) + b; } else if (t < 2 / 2.75) { return c * (7.5625 * (t -= 1.5 / 2.75) * t + .75) + b; } else if (t < 2.5 / 2.75) { return c * (7.5625 * (t -= 2.25 / 2.75) * t + .9375) + b; } else { return c * (7.5625 * (t -= 2.625 / 2.75) * t + .984375) + b; } }; Particle.prototype.move = function move(tick) { if (this.x !== this.destX) { this.x = this[settings.easing](tick, this.startX, this.destX - this.startX, settings.duration); } if (this.y !== this.destY) { this.y = this[settings.easing](tick, this.startY, this.destY - this.startY, settings.duration); } }; return Particle;
}();
var Writer = function () { function Writer(canvasId) { _classCallCheck(this, Writer); var canvas = document.getElementById(canvasId); this.ctx = canvas.getContext("2d"); this.w = canvas.width = 900; this.h = canvas.height = 300; // A list of all the particles that forms the text this.particles = []; this.tick = 0; } Writer.prototype.init = function init(text, size) { // My hope is that this will force the font to // be loaded before we try to draw text. // Should be synchronous fetch but sometimes // the default font is used instead! W00t?! WebFont.load({ google: { families: ["Ubuntu"] } }); this.ctx.fillStyle = "#00002F"; this.ctx.shadowColor = "#445"; this.ctx.shadowOffsetX = 3; this.ctx.shadowOffsetY = 5; this.ctx.shadowBlur = 5; this.ctx.font = size + "px Ubuntu"; // Draw text on the canvas temporarily var textWidth = this.ctx.measureText(text).width; var startX = (this.w - textWidth) * 0.5; var startY = (this.h - size) * 0.25; this.ctx.fillText(text, 0, size); var image = this.ctx.getImageData(0, 0, this.w, this.h); var buffer32 = new Uint32Array(image.data.buffer); for (var x = 0; x < this.w; x++) { for (var y = 0; y < this.h; y++) { // The buffer is linear, y*w+x is a trick // to calculate the linear index. var color = buffer32[y * this.w + x]; if (color) { // There is a pixel here, add a particle this.particles.push(new Particle(startX + x, startY + y, Math.round(Math.random() * this.w), Math.round(Math.random() * this.h), color)); } } } this.ctx.clearRect(0, 0, this.w, this.h); }; Writer.prototype.draw = function draw() { var _this = this; // Start every frame with an empty image var imageData = this.ctx.createImageData(this.w, this.h); var pixels = new Uint32Array(imageData.data.buffer); this.particles.forEach(function (p) { var x = Math.round(p.x); var y = Math.round(p.y); if (x >= 0 && x < _this.w && y >= 0 && y < _this.h) { pixels[x + _this.w * y] = p.color; } if (_this.tick > settings.startDelay) { p.move(_this.tick - settings.startDelay); } }); this.ctx.putImageData(imageData, 0, 0); this.tick++; requestAnimationFrame(function () { return _this.draw(); }); }; return Writer;
}();
window.onload = function () { var writer = new Writer("canvas"); writer.init(settings.text, settings.textSize); writer.draw();
};
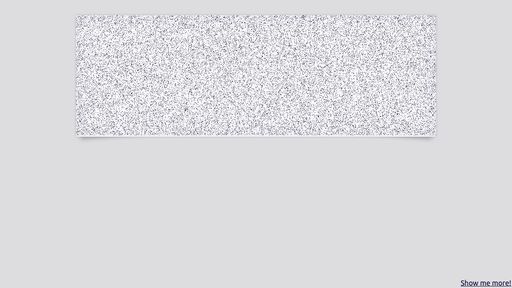
Developer | Johan Karlsson |
Username | DonKarlssonSan |
Uploaded | July 26, 2022 |
Rating | 4.5 |
Size | 5,685 Kb |
Views | 85,008 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Brownian Gnats | 2,822 Kb |
Wayward Walker Worms | 3,123 Kb |
Random Fractal | 2,751 Kb |
The Birth of a Sine Wave | 2,607 Kb |
SoundCloud Music Visualizer | 4,211 Kb |
Antenna Generator | 2,624 Kb |
Apply Filter Effects to Music | 4,027 Kb |
Text by Brownian Motion | 3,182 Kb |
Lissajous x Lissajous | 3,369 Kb |
Rainbow 6-fold Pentille | 4,311 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Flat buttons for Eliassen.com | Kdooley89 | 1,737 Kb |
Canvas stripes | Adrianparr | 1,948 Kb |
Canvas snow | Win7killer | 2,572 Kb |
A Pen by Eka Risyana | Risyana | 3,705 Kb |
Form Labels | Bartuc | 2,717 Kb |
Mini Profile | Frytyler | 3,828 Kb |
Ocean | Gordonnl | 2,817 Kb |
Hard-Stop Gradients | Mackdoyle | 2,288 Kb |
Responsive Minimal Blog Layout | Hackthevoid | 5,261 Kb |
Iron Man SVG Loading Animation | Andythayer | 3,069 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!