Password strength meter
How do I make an password strength meter?
What is a password strength meter? How do you make a password strength meter? This script and codes were developed by Rico Sta. Cruz on 19 January 2023, Thursday.
Password strength meter - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Password strength meter</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel='stylesheet prefetch' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class='form'> <input type='text' placeholder='password' data-js-password class='form-control'> <div class='password-meter-result' data-js-password-meter> </div>
</div> <script src='https://code.jquery.com/jquery-3.2.1.min.js'></script>
<script src='https://unpkg.com/[email protected]/dist/loadjs.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Password strength meter - Script Codes CSS Codes
* { box-sizing: border-box;
}
html, body { height: 100%;
}
body { display: -webkit-box; display: -ms-flexbox; display: flex; -webkit-box-pack: center; -ms-flex-pack: center; justify-content: center; -webkit-box-align: center; -ms-flex-align: center; align-items: center; background: #eee;
}
.form { width: 320px; min-height: 300px; background: white; padding: 16px;
}
.password-meter { margin: 16px 0;
}
/* Bar */
.password-meter-bar { display: -webkit-box; display: -ms-flexbox; display: flex
}
.password-meter-bar > span { -webkit-box-flex: 1; -ms-flex: 1 1 20%; flex: 1 1 20%; height: 4px; background-color: #eceff1; border-left: solid 1px rgba(255, 255, 255, .6);
}
.password-meter-bar > span:first-child { border-top-left-radius: 3px; border-bottom-left-radius: 3px; border-left: 0;
}
.password-meter-bar > span:last-child { border-top-right-radius: 3px; border-bottom-right-radius: 3px;
}
.password-meter-bar.-score-0 > span:nth-child(1) { background-color: #f44336;
}
.password-meter-bar.-score-1 > span:nth-child(1), .password-meter-bar.-score-1 > span:nth-child(2) { background-color: #f44336;
}
.password-meter-bar.-score-2 > span:nth-child(1), .password-meter-bar.-score-2 > span:nth-child(2), .password-meter-bar.-score-2 > span:nth-child(3) { background-color: #cddc39;
}
.password-meter-bar.-score-3 > span:nth-child(1), .password-meter-bar.-score-3 > span:nth-child(2), .password-meter-bar.-score-3 > span:nth-child(3), .password-meter-bar.-score-3 > span:nth-child(4) { background-color: #8bc34a;
}
.password-meter-bar.-score-4 > span { background-color: #8bc34a;
}
/* Strength: weak */
.password-meter-details { margin-top: 8px
}
.password-meter-details > .strength, .password-meter-details > .hint { margin: 0; padding: 0;
}
.password-meter-details > .strength > .lbl {
}
.password-meter-details > .strength > .score { font-weight: bold;
}
.password-meter-details > .hint { color: #888;
}
.password-meter-suggestions {
}
.password-meter-suggestions, .password-meter-suggestions > li { list-style-type: none; margin: 0; padding: 0;
}
.password-meter-suggestions { margin-top: 8px; display: none;
}
Password strength meter - Script Codes JS Codes
const ZXCVBN = 'https://unpkg.com/[email protected]/dist/zxcvbn.js'
const SCORES = { 0: 'Very weak', 1: 'Weak', 2: 'Fair', 3: 'Good', 4: 'Secure'
}
/* * Checks a password via zxcvbn. */
function scorePassword (...input) { return new Promise((resolve, reject) => { if (window.zxcvbn) { return resolve(window.zxcvbn(...input)) } window.loadjs(ZXCVBN, { success: () => { resolve(window.zxcvbn(...input)) } }) })
}
/* * Preloads zxcvbn */
function prefetch () { return scorePassword()
}
/* * Turns a string into a sentence. */
function sentence (str) { return str && str.replace(/\.?$/, '.')
}
/* * Returns HTML to be rendered based on zxcvbn's result. */
function template (result) { const scoreName = SCORES[result.score] const hint = sentence(result.feedback && result.feedback.warning) const suggestions = result.feedback && result.feedback.suggestions return ` <div class='password-meter -score-${result.score}'> <div class='password-meter-bar -score-${result.score}'> <span></span><span></span><span></span><span></span><span></span> </div> <div class='password-meter-details -score-${result.score}'> <p class='strength'> <span class='lbl'>Password strength:</span> <strong class='score'>${scoreName}</strong> <span class='icon'></span> </p> ${hint ? `<p class='hint'>${hint}</p>` : ''} </div> ${suggestions ? `<ul class='password-meter-suggestions'> ${result.feedback.suggestions.map(s => ` <li class='suggestion'>${s}</li> `)} </ul>` : ''} </div> `
}
/* * Runs the password meter. * Checks `password` and updates HTML of DOM element `$meter`. */
function runMeter (password, options = {}) { const score = options.scorePassword || scorePassword if (password) { return Promise.resolve(score(password)) .then(result => template(result)) } else { return Promise.resolve(null) }
}
/* * Integration */
var $password = document.querySelector('[data-js-password]')
var $meter = document.querySelector('[data-js-password-meter]')
// Preload zxcvbn
$($password).on('focus', () => { prefetch()
}
$($password).on('input', (e) => { runMeter(e.target.value, { scorePassword: customScorePassword }) .then(html => { $meter.innerHTML = html || 'Enter a password' })
})
/* Optional: score a password with your own criteria */
function customScorePassword (input) { if (!input.match(/[A-Z]/)) { return { score: 0, feedback: { warning: 'Please add an uppercase letter' } } } else if (input.length < 8) { return { score: 0, feedback: { warning: 'Please use at least 8 characters' } } } else { return scorePassword(input) }
}
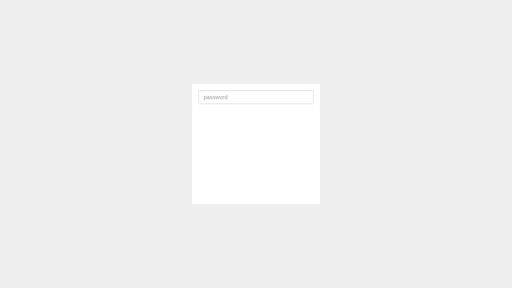
Developer | Rico Sta. Cruz |
Username | rstacruz |
Uploaded | January 19, 2023 |
Rating | 3 |
Size | 5,269 Kb |
Views | 4,048 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Diff-based typing test | 4,262 Kb |
Decca example | 2,444 Kb |
Cookie notice snippet | 2,651 Kb |
Daily UI 6 | 3,115 Kb |
React template | 1,886 Kb |
Coffee image card | 4,570 Kb |
Text in a border | 2,482 Kb |
Fidget spinner in CSS | 2,074 Kb |
Swipeshow demo | 2,133 Kb |
Gradient washing | 1,714 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Personal Website Redesign v2.0 | DevItWithDavid | 5,168 Kb |
CSS Gem Badge | Orchard | 3,335 Kb |
Nested table email layout | Massimo-cassandro | 2,355 Kb |
Responsive Advert | James_zedd | 2,354 Kb |
Google Fonts Sass Mixin | HugoGiraudel | 4,237 Kb |
Shape Outside - Polygon | Stacy | 3,954 Kb |
Resizable SASS Icons | Marianarlt | 7,611 Kb |
Drawing a Terminal with CSS | Lachlanjc | 3,185 Kb |
Search Box in Content Moves to Fixed Header | Chriscoyier | 2,768 Kb |
Classy Blockquote Styling | Andrewwright | 3,212 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!