Planet z-invert cutoff render visualized
How do I make an planet z-invert cutoff render visualized?
What is a planet z-invert cutoff render visualized? How do you make a planet z-invert cutoff render visualized? This script and codes were developed by Elliot on 22 December 2022, Thursday.
Planet z-invert cutoff render visualized - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Planet z-invert cutoff render visualized</title>
</head>
<body> <canvas id="c" height=560 width=1000 tabindex='1'></canvas>
<img id="source" src="https://upload.wikimedia.org/wikipedia/commons/thumb/8/83/Equirectangular_projection_SW.jpg/1920px-Equirectangular_projection_SW.jpg"> <script src='https://rawgit.com/vezwork/World-points/master/paths.js'></script> <script src="js/index.js"></script>
</body>
</html>
Planet z-invert cutoff render visualized - Script Codes JS Codes
"use strict";
var c = document.getElementById("c");
var ctx = c.getContext("2d");
function drawPlanet(planet) { ctx.beginPath(); ctx.arc(planet.origin.x, //x origin planet.origin.y, //y origin planet.radius, //radius 0, Math.PI * 2, false); ctx.fillStyle = "rgba(200, 200, 200, 1)"; ctx.fill();
}
function projectOnPlanet(planet, coord) { var rad = planet.radius + coord.z; var x = coord.x + planet.rot.x; var y = coord.y; var cx = rad * Math.sin(x) * Math.sin(y); var icy = rad * Math.cos(y); var icz = rad * Math.cos(x) * Math.sin(y); //do a cartesian rotation on the x axis for planet y rot var cy = icy * Math.cos(planet.rot.y) + icz * Math.sin(planet.rot.y); var cz = icz * Math.cos(planet.rot.y) - icy * Math.sin(planet.rot.y); return { x: cx, y: cy, z: cz };
}
function projectOnPlanetZInvert(planet, coord) { var _a = projectOnPlanet(planet, coord), x = _a.x, y = _a.y, z = _a.z; var rad = planet.radius + coord.z; if (z < 0) { var r = Math.sqrt(Math.pow(x, 2) + Math.pow(y, 2)); var r_ = 2 * rad - r; x = (x / r) * r_; y = (y / r) * r_; } return { x: x, y: y, z: z };
}
function drawPathOnPlanet(planet, coords) { if (coords.length > 1) { ctx.beginPath(); var next_1 = projectOnPlanetZInvert(planet, coords[0]); var p1_1 = [], p2_1 = [], p3_1 = [], p4_1 = [], p5_1 = [], p6_1 = []; var resolve_1 = undefined; var resolveAtEnd_1 = undefined; coords.forEach(function (coord, i) { var cur = next_1; if (coords[i + 1] !== undefined) next_1 = projectOnPlanetZInvert(planet, coords[i + 1]); else next_1 = projectOnPlanetZInvert(planet, coords[0]); if (i === 0) p6_1.push(cur); //do stuff here if (cur.z >= 0 && next_1.z >= 0) { ctx.lineTo(planet.origin.x + cur.x, planet.origin.y + cur.y); } else if (cur.z >= 0 && next_1.z <= 0) { ctx.lineTo(planet.origin.x + cur.x, planet.origin.y + cur.y); ctx.lineTo(planet.origin.x + next_1.x, planet.origin.y + next_1.y); resolve_1 = next_1; p3_1.push(next_1); p4_1.push(cur); } else if (cur.z <= 0 && next_1.z >= 0) { if (resolve_1 === undefined) resolveAtEnd_1 = cur; else { //draw point between cur and resolve var avg = { x: (cur.x + resolve_1.x) / 2, y: (cur.y + resolve_1.y) / 2 }; var r = Math.sqrt(Math.pow(avg.x, 2) + Math.pow(avg.y, 2)); var r_ = 2 * planet.radius; avg.x = avg.x / r * r_; avg.y = avg.y / r * r_; ctx.lineTo(planet.origin.x + avg.x, planet.origin.y + avg.y); } ctx.lineTo(planet.origin.x + cur.x, planet.origin.y + cur.y); p1_1.push(next_1); p2_1.push(cur); } else { p5_1.push(cur); } }); if (resolveAtEnd_1 !== undefined) { var avg = { x: (resolveAtEnd_1.x + resolve_1.x) / 2, y: (resolveAtEnd_1.y + resolve_1.y) / 2 }; var r = Math.sqrt(Math.pow(avg.x, 2) + Math.pow(avg.y, 2)); var r_ = 2 * planet.radius; avg.x = avg.x / r * r_; avg.y = avg.y / r * r_; ctx.lineTo(planet.origin.x + avg.x, planet.origin.y + avg.y); } ctx.closePath(); ctx.fillStyle = "white"; ctx.fill(); function drawColor(col, s) { if (s === void 0) { s = 5; } return function (_a) { var x = _a.x, y = _a.y; ctx.fillStyle = col; ctx.fillRect(planet.origin.x + x, planet.origin.y + y, s, s); }; } p6_1.forEach(drawColor("black", 10)); p5_1.forEach(drawColor("white")); p1_1.forEach(drawColor("red")); p2_1.forEach(drawColor("orange")); p3_1.forEach(drawColor("blue"), 4); p4_1.forEach(drawColor("purple")); }
}
function drawCircleOnPlanet(planet, circle) { var _a = projectOnPlanet(planet, circle.coord), x = _a.x, y = _a.y, z = _a.z; if (z > 0) { ctx.beginPath(); ctx.ellipse(planet.origin.x + x, //x origin planet.origin.y + y, //y origin Math.sin(Math.PI / 2 * Math.abs((rad - Math.sqrt(Math.pow(x, 2) + Math.pow(y, 2))) / rad)) * circle.radius, //x radius circle.radius, //y radius Math.atan2(y, x), //rot 0, Math.PI * 2, false); //how to draw a full ellipse. ctx.fill(); ctx.stroke(); }
}
ctx.strokeStyle = "black";
ctx.fillStyle = "white";
var globe_img = document.getElementById("source");
var planet = { radius: 250, origin: { x: 450, y: 280, z: 0 }, rot: { x: 0, y: 0 }
};
var mini = { radius: 10, origin: { x: 450, y: 280, z: 0 }, rot: { x: 0, y: 0 }
};
var mini2 = { radius: 1, origin: { x: 450, y: 280, z: 0 }, rot: { x: 0, y: 0 }
};
var path = [];
var togglePath = true;
var mapWidth = globe_img.width * 3, mapHeight = globe_img.height * 3;
function draw() { ctx.fillStyle = "#42bff4"; ctx.fillRect(0, 0, c.width, c.height); ctx.fillStyle = "white"; //stitching underlay ctx.drawImage(globe_img, 450 + mapWidth / 2 * planet.rot.x / Math.PI, 280 + mapHeight / 2 * planet.rot.y / Math.PI - mapHeight / 4, mapWidth, mapHeight / 2); drawPlanet(planet); drawPlanet(mini); drawPlanet(mini2); ctx.fillText(planet.rot.x + " : " + planet.rot.y, 10, 10); if (togglePath) { drawPathOnPlanet(planet, path); drawPathOnPlanet(planet, americas_path); } //planet.rot.x += 0.01; //planet.rot.y += 0.001; requestAnimationFrame(draw);
}
draw();
var offsetPrev = { x: 0, y: 0 };
c.addEventListener("mousemove", function (e) { if (e.altKey) { planet.rot.x -= (offsetPrev.x - e.offsetX) * 0.002; planet.rot.y -= (offsetPrev.y - e.offsetY) * 0.002; } offsetPrev.x = e.offsetX; offsetPrev.y = e.offsetY;
});
c.addEventListener("keydown", function (e) { if (e.key === "z") togglePath = !togglePath; if (e.key === "p") console.log(JSON.stringify(path)); if (e.key === "q") mapHeight += 200; if (e.key === "a") mapHeight -= 200; if (e.key === "w") mapWidth += 200; if (e.key === "s") mapWidth -= 200;
});
c.addEventListener("mousedown", function (_) { path.push({ x: -planet.rot.x, y: Math.PI / 2 + planet.rot.y, z: 0 });
});
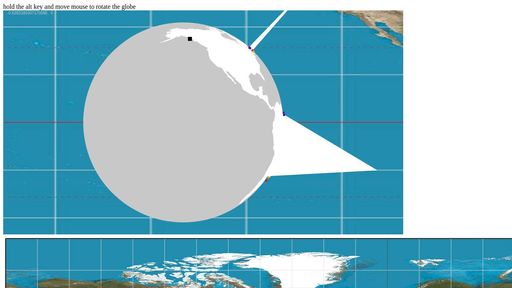
Developer | Elliot |
Username | vez |
Uploaded | December 22, 2022 |
Rating | 3 |
Size | 5,249 Kb |
Views | 12,144 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Es6 style odd one out | 1,502 Kb |
Vid player | 2,513 Kb |
Sierpinski carpet | 1,746 Kb |
Scrolling highlight | 1,664 Kb |
Drag and drop image 1bitifier | 2,087 Kb |
Simple Collatz Generator | 1,706 Kb |
Js heap | 1,724 Kb |
Weird ES6 Shenanigans | 1,885 Kb |
Progress Scroll | 2,037 Kb |
A Pen by Elliot | 1,664 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Bootstrap 3 Price Table | Honglio | 2,655 Kb |
Obligatory CSS3 UI Nav | Romandiaz | 9,017 Kb |
React Template | Isac | 1,241 Kb |
Loader | MikitaLisavets | 3,321 Kb |
Social Profiles | Lachlanjc | 1,939 Kb |
Sign Up Form | Sicontis | 5,272 Kb |
Cartoon Bomb | Tcmulder | 4,929 Kb |
Spin | Elalemanyo | 8,262 Kb |
A Pen by tugce | Ecgutcnkr | 4,197 Kb |
Flat design iframe | Damienpm | 1,819 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!