Progress bar animation that loops perfectly, hopefully
How do I make an progress bar animation that loops perfectly, hopefully?
Promises should make sure the progress bar is reset to 0 at the end of the animation.. What is a progress bar animation that loops perfectly, hopefully? How do you make a progress bar animation that loops perfectly, hopefully? This script and codes were developed by AciD on 15 October 2022, Saturday.
Progress bar animation that loops perfectly, hopefully - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Progress bar animation that loops perfectly, hopefully</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="container"> <div class="progressBar bar1">setTimeout</div> <div class="progressBar bar2">setTimeout with a magic number</div> <div class="progressBar bar3">Promises</div> <div class="progressBar bar4">Overly complicated Xin Method</div> <div class="progressBar bar5">Events</div>
</div> <script src="js/index.js"></script>
</body>
</html>
Progress bar animation that loops perfectly, hopefully - Script Codes CSS Codes
.progressBar { opacity: 0.80; height: 20px; width: 2%; -webkit-transform: translate3d(0px, 0px, 0px); transform: translate3d(0px, 0px, 0px); -webkit-transition: none; transition: none; overflow: hidden; text-align: right; padding-right: 1rem; font-weight: 600; color: #333;
}
.progressBar:not(:last-child) { margin-bottom: 2rem;
}
.progressBar.bar1 { background-color: #69D2E7;
}
.progressBar.bar2 { background-color: #A7DBD8;
}
.progressBar.bar3 { background-color: #E0E4CC;
}
.progressBar.bar4 { background-color: tomato;
}
.progressBar.bar5 { background-color: lime;
}
.progressBar.progressing { -webkit-transition: width 3s linear; transition: width 3s linear;
}
* { padding: 0; margin: 0; box-sizing: border-box;
}
.container { height: 100vh; display: -webkit-box; display: -ms-flexbox; display: flex; -webkit-box-orient: vertical; -webkit-box-direction: normal; -ms-flex-direction: column; flex-direction: column; -webkit-box-pack: center; -ms-flex-pack: center; justify-content: center;
}
body { background: #333; color: white; font-family: "Open sans", sans-serif; font-weight: 100; font-size: 1rem;
}
a.button { color: yellow; text-decoration: none; -webkit-transition: color 0.3s ease; transition: color 0.3s ease;
}
a.button:focus { outline: none;
}
a.button:hover { color: lime;
}
.button2 { background-color: deeppink; color: white; border: 1px solid red; border-radius: 2px; height: 30px; cursor: pointer; outline: none;
}
.button2:focus, .button2:active { outline: none;
}
Progress bar animation that loops perfectly, hopefully - Script Codes JS Codes
'use strict';
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
var ProgressBar = function () { function ProgressBar(progressBar) { var method = arguments.length <= 1 || arguments[1] === undefined ? 'setTimeout' : arguments[1]; var delayBetweenEachAnimation = arguments.length <= 2 || arguments[2] === undefined ? 3000 : arguments[2]; _classCallCheck(this, ProgressBar); this.progressBar = progressBar; this.method = method; this.delayBetweenEachAnimation = delayBetweenEachAnimation; this.isAnimating = false; this.launchAnimation(); } ProgressBar.prototype.launchAnimation = function launchAnimation() { var _this = this; switch (this.method) { case 'setTimeout': console.log("launchAnimation() with setTimeout"); //DEBUG this.intervalID = window.setInterval(function () { return _this.progressToViaSetTimeout(); }, this.delayBetweenEachAnimation); break; case 'setTimeoutMagicNumber': console.log("launchAnimation() with setTimeout and a magic number"); //DEBUG this.intervalID = window.setInterval(function () { return _this.progressToViaSetTimeoutMagicNumber(); }, this.delayBetweenEachAnimation); break; case 'Promises': console.log("launchAnimation() with Promises"); //DEBUG this.intervalID = window.setInterval(function () { return _this.progressToViaPromises(); }, this.delayBetweenEachAnimation); break; case 'SassyUnhelpfulXin': console.log("launchAnimation() with...err..WTF?!"); //DEBUG this.intervalID = window.setInterval(function () { return _this.progressToWtfXin(); }, this.delayBetweenEachAnimation); break; case 'Events': console.log("launchAnimation() with Events"); //DEBUG this._setEventListener(); this.intervalID = window.setInterval(function () { return _this.progressToViaEvents(); }, this.delayBetweenEachAnimation); break; default: console.log('whoops, something went wrong.'); } }; //---------------------------------------------------------------- //Method 1 : Not reliable since the reset sometimes can not occur ProgressBar.prototype.progressToViaSetTimeout = function progressToViaSetTimeout() { var _this2 = this; var value = arguments.length <= 0 || arguments[0] === undefined ? 100 : arguments[0]; this._progressTo(value); //Remove the progressing class once the animation is finished this.progressBarTimeoutID = window.setTimeout(function () { return _this2._resetProgressBar(); }, this.delayBetweenEachAnimation); //FIXME A magic number (-30 for instance) is needed here in order to allow the animation to finish first }; //---------------------------------------------------------------- //Method 2 : Not reliable since the reset sometimes can not occur, event with a magic number ProgressBar.prototype.progressToViaSetTimeoutMagicNumber = function progressToViaSetTimeoutMagicNumber() { var _this3 = this; var value = arguments.length <= 0 || arguments[0] === undefined ? 100 : arguments[0]; this._progressTo(value); //Remove the progressing class once the animation is finished this.progressBarTimeoutID = window.setTimeout(function () { return _this3._resetProgressBar(); }, this.delayBetweenEachAnimation - 50); //FIXME A magic number (-30 for instance) is needed here in order to allow the animation to finish first. Well...how big sholud be that number? }; //---------------------------------------------------------------- //Method 3 : Use Promises to make sur the 'progressing' CSS class is _always_ removed after the animation is finished ProgressBar.prototype.progressToViaPromises = function progressToViaPromises() { var _this4 = this; var value = arguments.length <= 0 || arguments[0] === undefined ? 100 : arguments[0]; //FIXME Only the first Promise is changing the .progressing class, why is that? var addProgressingClass = new Promise(function (resolve, reject) { console.log("addProgressingClass() launched.", value); //DEBUG _this4.progressBar.classList.add('progressing'); _this4.progressBar.style.width = value + '%'; window.setTimeout(function () { resolve('ok, promise fulfilled!'); //We fulfill the promise ! }, _this4.delayBetweenEachAnimation); }); addProgressingClass.then(function (val) { //Log the fulfillment value console.log("Then() : ", val); //DEBUG _this4._resetProgressBar(); }).catch(function (reason) { //Log the rejection reason console.log('The promise could no be fulfilled [' + reason + ']'); }); }; //---------------------------------------------------------------- //Method 4 : (If you get that in the first read, good for you!) : // "<Xin> Honestly, use an event emitter on your carousel to trigger a restart of a single setTimeout that triggers a css animation to RESTART every time its triggered, or after x seconds to trigger the carousels next event" // // This is translated like this : // 1) Every X seconds, emit an event "carouselSlideShown" (those are emitted by the the carousel normaly) // 2) Catch that event and setTimeout the 'addProgressingCSSClass' function // 3) WTF!? // // // What I want to achieve : // 1) Every X seconds, the carousel emit an event "carouselSlideShown" (X is usually the same value between each loop, but that can vary, since the user can pause the animation for as long as he wants) // 2) Catch that "carouselSlideShown" event and : // a) Immediatly reset the progress bar width to 0%, without animation, // b) Then immediatly start the progress bar animation to width 100% // 3) When the progress bar animation is finished, reset the progress bar width to 0%, without animation. // 4) Repeat. // // Notes : // I) at any moment the user can pause the animation by hovering either the carousel or the controls // II) at any moment the user can switch to any other carousel pane, which means quickly animating the progressbar to 0% while the carousel show an animation to select said pane. Then once the pane is shown, rinse and repeat the usual loop. ProgressBar.prototype.progressToWtfXin = function progressToWtfXin() { var value = arguments.length <= 0 || arguments[0] === undefined ? 100 : arguments[0]; } //WTF. /* _simulateCarouselEventEmit(slideNumber = 1) { let carouselEvent = new CustomEvent('carouselSlideShown', { detail: { 'slideNumber': slideNumber } }); document.dispatchEvent(carouselEvent); } */ //---------------------------------------------------------------- //Method 5 : Use the 'transitionend' event to make sur the 'progressing' CSS class is _always_ removed after the animation is finished ; ProgressBar.prototype.progressToViaEvents = function progressToViaEvents() { var value = arguments.length <= 0 || arguments[0] === undefined ? 100 : arguments[0]; this._resetProgressBar(); this.isAnimating = true; this.progressBar.classList.add('progressing'); this.progressBar.style.width = value + '%'; }; ProgressBar.prototype._setEventListener = function _setEventListener() { var _this5 = this; // this.progressBar.addEventListener("transitionend", () => this._resetProgressBar(), false); //FIXME Uncomment this.progressBar.addEventListener("transitionend", function () { //FIXME delete that console.log('got a "transitionend" event!'); //DEBUG if (!_this5.isAnimating) { //If this is currently animating, it means a new animation has been launched, and I should not cancel it prematurely. _this5._resetProgressBar(); } }, false); }; //-------------------- Private methods -------------------- ProgressBar.prototype._resetProgressBar = function _resetProgressBar() { console.log('_resetProgressBar()'); //DEBUG this.isAnimating = false; this.progressBar.classList.remove('progressing'); this.progressBar.style.width = '0%'; }; ProgressBar.prototype._progressTo = function _progressTo(value) { this._cancelProgressBarProgressingClass(); this._resetProgressBar(); //I make sure the progress bar is set to 0% BEFORE the animation is done this.progressBar.classList.add('progressing'); this.progressBar.style.width = value + '%'; }; ProgressBar.prototype._cancelProgressBarProgressingClass = function _cancelProgressBarProgressingClass() { window.clearTimeout(this.progressBarTimeoutID); }; return ProgressBar;
}();
// let pbTimeout1 = new ProgressBar(document.querySelector('.bar1'), 'setTimeout');
// let pbTimeout2 = new ProgressBar(document.querySelector('.bar2'), 'setTimeoutMagicNumber');
// let pbPromise = new ProgressBar(document.querySelector('.bar3'), 'Promises');
// let pbWTF = new ProgressBar(document.querySelector('.bar4'), 'SassyUnhelpfulXin');
var pbEvents = new ProgressBar(document.querySelector('.bar5'), 'Events');
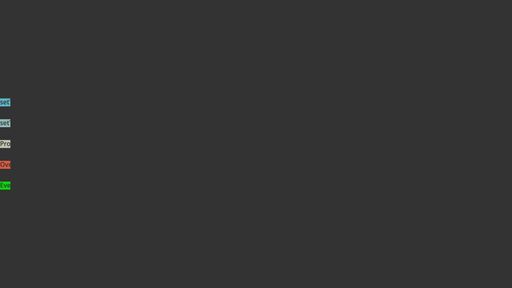
Developer | AciD |
Username | AnotherLinuxUser |
Uploaded | October 15, 2022 |
Rating | 3 |
Size | 7,573 Kb |
Views | 20,240 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Email Marketing Mock | Kristenzirkler | 8,224 Kb |
RWD Conversion Practice | Jxqr97 | 2,743 Kb |
Animate a paper plane along an SVG path, looking ahead | PotatoDie | 3,734 Kb |
Vue.js Starter | Andymerskin | 1,268 Kb |
3d css cube | Semenchenko | 4,578 Kb |
Rain Landing in a Pond | Edball | 3,009 Kb |
Search field | Jamesbarnett | 2,100 Kb |
CSS Grid Overlay | Cliffpyles | 3,090 Kb |
Price table | Serluk | 5,928 Kb |
Animated rainbow wave on canvas | Icodeforlove | 2,777 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!