RangeInputFilter
How do I make an rangeinputfilter?
What is a rangeinputfilter? How do you make a rangeinputfilter? This script and codes were developed by Gregory Potdevin on 01 October 2022, Saturday.
RangeInputFilter - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>RangeInputFilter</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel='stylesheet prefetch' href='http://cdn.jsdelivr.net/searchkit/0.4.0/styles.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="app"></div> <script src='http://cdnjs.cloudflare.com/ajax/libs/react/0.14.3/react.min.js'></script>
<script src='http://cdn.jsdelivr.net/searchkit/0.4.0/bundle.js'></script>
<script src='http://cdnjs.cloudflare.com/ajax/libs/lodash.js/3.5.0/lodash.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
RangeInputFilter - Script Codes CSS Codes
.search__query { position: absolute; top: 0; left: 0; right: 0; height: 41px; display: flex; border-bottom: 1px solid #ddd;
}
.search .search-box { height: 40px; flex: 1;
}
.search .search-box__loader { display: none;
}
.search__results { position: absolute; top: 41px; left: 0; right: 0;
}
.search .hits-list-item { display: block; padding: 16px; width: 100%;
}
.search .hits-hit { text-align: center;
}
.search .hits-hit a { text-decoration: none;
}
.search .hits-hit__poster { max-width: 100px; max-height: 140px; display: inline-block;
}
.search .hits-hit__title { font-size: 12px; text-align: center; margin-top: 5px;
}
RangeInputFilter - Script Codes JS Codes
"use strict";
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var host = "https://kili-eu-west-1.searchly.com/movies/";
var sk = new Searchkit.SearchkitManager(host, { multipleSearchers: false, basicAuth: "read:teetndhjnrspbzxxyfxmf5fb24suqxuj"
});
var FastClick = Searchkit.FastClick;
var SearchkitProvider = Searchkit.SearchkitProvider;
var Searchbox = Searchkit.SearchBox;
var Hits = Searchkit.Hits;
var HitsStats = Searchkit.HitsStats;
var RangeFilter = Searchkit.RangeFilter;
var RangeInput = function (_React$Component) { _inherits(RangeInput, _React$Component); function RangeInput(props) { _classCallCheck(this, RangeInput); var _this = _possibleConstructorReturn(this, _React$Component.call(this, props)); _this.state = { min: "" + props.min, max: "" + props.max }; return _this; } return RangeInput;
}(React.Component);
var RangeInputFilter = function (_RangeFilter) { _inherits(RangeInputFilter, _RangeFilter); function RangeInputFilter(props) { _classCallCheck(this, RangeInputFilter); var _this2 = _possibleConstructorReturn(this, _RangeFilter.call(this, props)); _this2.state = { minStr: "" + props.min, maxStr: "" + props.max }; _this2.handleInputChange = _this2.handleInputChange.bind(_this2); _this2.handleSubmit = _this2.handleSubmit.bind(_this2); _this2.sliderUpdate = _this2.sliderUpdate.bind(_this2); _this2.debounceSearch = _.debounce(function () { console.log('time to search'); _this2.searchkit.performSearch(); }, 500); return _this2; } RangeInputFilter.prototype.sliderUpdate = function sliderUpdate(newValues) { if (newValues[0] == this.props.min && newValues[1] == this.props.max) { this.accessor.state = this.accessor.state.clear(); } else { this.accessor.state = this.accessor.state.setValue({ min: newValues[0], max: newValues[1] }); } this.setState({ min: "" + newValues[0], max: "" + newValues[1] }); }; RangeInputFilter.prototype.handleSubmit = function handleSubmit(e) { e.preventDefault(); this.sliderUpdateAndSearch([parseInt(this.state.min, 10), parseInt(this.state.max, 10)]); }; RangeInputFilter.prototype.handleInputChange = function handleInputChange(type, e) { var state = { min: this.state.min, max: this.state.max }; state[type] = e.target.value; this.setState(state); this.sliderUpdate([parseInt(state.min, 10), parseInt(state.max, 10)]); this.debounceSearch(); }; RangeInputFilter.prototype.render = function render() { var _this3 = this; return React.createElement( "div", null, _RangeFilter.prototype.render.call(this), React.createElement( "form", { onSubmit: this.handleSubmit }, React.createElement("input", { type: "number", style: { display: "inline-block", maxWidth: 70, margin: 4 }, value: this.state.min, onChange: function onChange(e) { return _this3.handleInputChange("min", e); } }), React.createElement("input", { type: "number", style: { display: "inline-block", maxWidth: 70, margin: 4 }, value: this.state.max, onChange: function onChange(e) { return _this3.handleInputChange("max", e); } }), React.createElement("input", { type: "submit", style: { display: "inline-block", maxWidth: 70, margin: 4 } }) ) ); }; return RangeInputFilter;
}(RangeFilter);
var Application = function (_React$Component2) { _inherits(Application, _React$Component2); function Application() { _classCallCheck(this, Application); var _this4 = _possibleConstructorReturn(this, _React$Component2.call(this)); _this4.state = { displayMode: "thumbnail" }; return _this4; } Application.prototype.onDisplayModeChange = function onDisplayModeChange(e) { this.setState({ displayMode: e.target.value }); }; Application.prototype.render = function render() { var displayMode = this.state.displayMode; return React.createElement( "div", null, React.createElement( SearchkitProvider, { searchkit: sk }, React.createElement( "div", { className: "search" }, React.createElement( "div", { className: "search__query" }, React.createElement(Searchbox, { searchOnChange: true, prefixQueryFields: ["actors^1", "type^2", "languages", "title^10"] }) ), React.createElement( "div", { className: "search__results", style: { maxWidth: 250 } }, React.createElement(HitsStats, null), React.createElement(RangeInputFilter, { min: 0, max: 100, field: "metaScore", id: "metascore", title: "Metascore", showHistogram: true }) ) ) ) ); }; return Application;
}(React.Component);
React.render(React.createElement(Application, null), document.getElementById('app'));
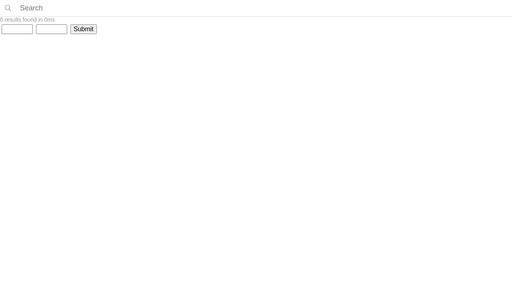
Developer | Gregory Potdevin |
Username | GregoryPotdevin |
Uploaded | October 01, 2022 |
Rating | 3 |
Size | 5,221 Kb |
Views | 16,192 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Page Numbers generator | 3,330 Kb |
A Pen by Gregory Potdevin | 1,713 Kb |
A Pen by Gregory Potdevin | 2,432 Kb |
Control | 2,855 Kb |
CheckboxFilter | 5,749 Kb |
RefinementListFilter sort | 5,022 Kb |
RangeFilter | 3,831 Kb |
HTML Select | 1,362 Kb |
Line | 1,781 Kb |
Shape Maker | 2,056 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Display properties | Hamzaerbay | 1,886 Kb |
CSS Grid Overlay | Cliffpyles | 3,090 Kb |
SVG hamburger menu button | Elifitch | 2,602 Kb |
Wikipedia viewer | Chpecson | 2,865 Kb |
CSS Social Media Icon | TychoBlender | 3,871 Kb |
Infractions - Attitude | Kylie_Joseph | 7,672 Kb |
Siema - add pagination to prototype | Pawelgrzybek | 2,575 Kb |
3d css cube | Semenchenko | 4,578 Kb |
Toolbar | Onsen | 5,414 Kb |
Practice using Wixel | Emnk | 3,057 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!