React Calc
How do I make an react calc?
What is a react calc? How do you make a react calc? This script and codes were developed by Alexius M Wronka I I on 19 September 2022, Monday.
React Calc - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>React Calc</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="app"></app> <script src='http://cdnjs.cloudflare.com/ajax/libs/react/0.13.0/react.min.js'></script>
<script src='http://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.8.2/underscore-min.js'></script> <script src="js/index.js"></script>
</body>
</html>
React Calc - Script Codes CSS Codes
.calcBox { margin-top: 60px; position: relative; margin-right: auto; margin-left: auto; width: 400px; border-radius: 25px; height: 400px; border: 3px solid darkgrey; padding: 10px; background-color: lightgrey; box-shadow: -4px 4px 3px black;
}
.calcBox .title { /* display:inline-block;*/ overflow-x: scroll; margin-top: 2%; margin-left: 5%; margin-bottom: -30px; font-family: Verdana; color: grey; font-weight: bold;
}
.calcBox .number-line { margin-top: 20px; position: relative; margin-right: auto; border-top: 2px solid gray; border-bottom: 2px solid #E0E0E0; margin-left: auto; border-radius: 5px; width: 90%; height: 40px; background-color: white;
}
.calcBox .operations-box { display: flex; /* flex-direction:row;*/ margin-top: 10%; margin-bottom: 10%; margin-right: auto; margin-left: auto; float: left; align-content: center; flex-direction: column; width: 100%; /* flex-wrap: wrap;*/
}
.calcBox .operations-box .calc-row { display: flex; flex-direction: row; align-content: center;
}
.calcBox .operations-box .calc-row .calc-button { border-radius: 10px; text-align: center; margin: 2% 2%; padding: 10px; width: 18%; height: 20%; background-color: #B0C4DE; border-bottom: 2px solid skyblue; box-shadow: -.5px .5px 1px black;
}
.calcBox .operations-box .calc-row .calc-button text { font-family: Verdana; color: darkblue; font-weight: bold;
}
.calcBox .operations-box .calc-row .calc-button:active { background-color: lightblue; border-bottom: 2px solid #B0C4DE;
}
.calcBox .operations-box .calc-row .calc-button:active text { color: white;
}
.calcBox .operations-box .calc-row .clear { margin-left: auto; margin-right: auto; width: 60%;
}
.left { text-align: right; margin-right: 5%; font-size: 25px;
}
React Calc - Script Codes JS Codes
"use strict";
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
/* * A simple React component */
var title = "9";
var currOperand = null;
var temp = null;
var total = null;
var storVal = 0;
var equalFunc = null;
var eqCheck = false;
var eqPush = false;
//add numerical values to the title
function addValue(num) { console.log(num); title = num; console.log(title); if (title.indexOf('.') > 0 && num === "." && eqCheck === false) { title += ""; } else if (eqCheck === true) { title = num + ""; eqCheck = false; } else if (title === "0" && eqCheck === false && num !== ".") { if (total === null) { total = num + ""; } title = num + ""; } else if (eqCheck === false) { if (total === null) { total = num + ""; } title += num; }
}
//store title value on operation click and assign operation to equals button
var makeOperation = { "+": function _(x) { if (eqPush) { title = Number(total) + temp + ""; } eqCheck = true; temp = Number(x); currOperand = null; eqPush = true; equalFunc = function equalFunc(y) { eqPush = false; if (currOperand === null) { currOperand = Number(y); title = Number(x) + currOperand; } else { title = Number(title) + currOperand + ""; } }; }, "-": function _(x) { eqCheck = true; currOperand = null; equalFunc = function equalFunc(y) { eqPush = false; if (currOperand === null) { currOperand = Number(y); title = Number(x) - currOperand; } else { title = Number(title) - currOperand + ""; } }; }, "/": function _(x) { eqCheck = true; currOperand = null; equalFunc = function equalFunc(y) { eqPush = false; if (currOperand === null) { currOperand = Number(y); title = Number(x) / currOperand; } else { title = Number(title) / currOperand + ""; } }; }, "*": function _(x) { eqCheck = true; currOperand = null; equalFunc = function equalFunc(y) { eqPush = false; if (currOperand === null) { currOperand = Number(y); title = Number(x) * currOperand; } else { title = Number(title) * currOperand + ""; } }; }
};
//clear the calculator and memory
function clear() { console.log("works"); title = "0"; currVal = 0; storVal = 0; equalFunc = null; currOperand = null; total = null;
}
var objButton = { button: [[{ value: "7" }, { value: "8" }, { value: "9" }, { value: "+" }], [{ value: "4" }, { value: "5" }, { value: "6" }, { value: "-" }], [{ value: "1" }, { value: "2" }, { value: "3" }, { value: "*" }], [{ value: "." }, { value: "0" }, { value: "/" }, { value: "=" }], [{ value: "clear" }]], buttonFuncs: addValue, operandFuncs: makeOperation, clearFunc: clear };
var Button = function (_React$Component) { _inherits(Button, _React$Component); function Button() { _classCallCheck(this, Button); return _possibleConstructorReturn(this, _React$Component.apply(this, arguments)); } Button.prototype.render = function render() { return React.createElement( "div", { onClick: this.props.func, className: "calc-button" }, this.props.value ); }; return Button;
}(React.Component);
var Application = function (_React$Component2) { _inherits(Application, _React$Component2); function Application(props) { _classCallCheck(this, Application); var _this2 = _possibleConstructorReturn(this, _React$Component2.call(this, props)); _this2.state = { title: title }; return _this2; } Application.prototype.render = function render() { return React.createElement( "div", { className: "calcBox" }, React.createElement( "div", { className: "number-line" }, React.createElement( "div", { id: "fitin", className: "title left" }, React.createElement( "span", null, this.state.title ) ) ), React.createElement( "div", { className: "title" }, React.createElement( "span", null, "Easy Calc" ) ), React.createElement(OperationsBox, null) ); }; return Application;
}(React.Component);
var OperationsBox = function (_React$Component3) { _inherits(OperationsBox, _React$Component3); function OperationsBox(props) { _classCallCheck(this, OperationsBox); var _this3 = _possibleConstructorReturn(this, _React$Component3.call(this, props)); _this3.state = objButton; return _this3; } OperationsBox.prototype.numClick = function numClick() { console.log("5"); }; OperationsBox.prototype.render = function render() { var rows = []; this.state.button.forEach(function (button, index) { var row = button.map(function (obj, index2) { if (index === 4) { return React.createElement( "div", { onClick: clear.bind(this), className: "calc-button clear" }, obj.value ); } else { return React.createElement(Button, { func: addValue.bind(this, obj.value), value: obj.value }); } }.bind(this)); rows.push(row); }); return React.createElement( "div", { className: "operations-box" }, React.createElement( "div", { className: "calc-row" }, rows[0] ), React.createElement( "div", { className: "calc-row" }, rows[1] ), React.createElement( "div", { className: "calc-row" }, rows[2] ), React.createElement( "div", { className: "calc-row" }, rows[3] ), React.createElement( "div", { className: "calc-row" }, rows[4] ) ); }; return OperationsBox;
}(React.Component);
var CalcRow = function (_React$Component4) { _inherits(CalcRow, _React$Component4); function CalcRow() { _classCallCheck(this, CalcRow); return _possibleConstructorReturn(this, _React$Component4.apply(this, arguments)); } CalcRow.prototype.render = function render() { /* var buttons = {button: <Button/>}*/ /* var buttons = this.state.button.map(function(button, index){ return (<Button value={this.state.button[index].value}/>) }.bind(this))*/ return React.createElement("div", { className: "calc-row" }); }; return CalcRow;
}(React.Component);
/* * Render the above component into the div#app */
React.render(React.createElement(Application, null), document.getElementById('app'));
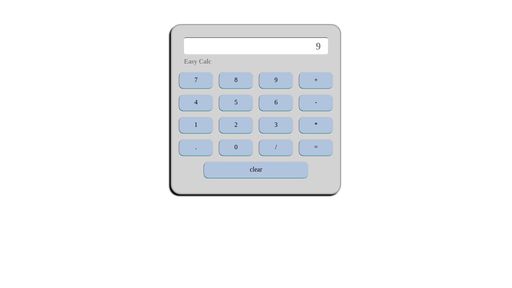
Developer | Alexius M Wronka I I |
Username | awronka |
Uploaded | September 19, 2022 |
Rating | 3 |
Size | 6,046 Kb |
Views | 24,288 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
A Pen by Alexius M Wronka I I | 2,375 Kb |
Angular 2 forms | 1,244 Kb |
Guage | 3,480 Kb |
Angular Calc | 3,707 Kb |
React Test | 1,232 Kb |
Animated Drop Down | 3,066 Kb |
D3 Dot Graph | 4,139 Kb |
Transition button | 4,112 Kb |
List React | 3,155 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Print element on a page | Mrs_snow | 2,081 Kb |
Portfolio Landing Page | FDfranklin | 3,585 Kb |
Count up | Alanshortis | 2,391 Kb |
Multiple jCarousel | Pafnuty | 2,461 Kb |
Javascript Welcome | Peterlewicki | 1,573 Kb |
CSS Bot Confusion | Jpod | 3,456 Kb |
Template | Indra_z85 | 2,323 Kb |
Video mute | Leon9208 | 2,131 Kb |
Buttons for autumn | Nikazawila | 1,795 Kb |
SVG Text Masking | JMChristensen | 2,141 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!