React Carousel
How do I make an react carousel?
Just trying out the CSSTransitionGroup add-on!. What is a react carousel? How do you make a react carousel? This script and codes were developed by Andy Pagès on 10 November 2022, Thursday.
React Carousel - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>React Carousel</title> <link rel='stylesheet prefetch' href='https://cdnjs.cloudflare.com/ajax/libs/foundicons/3.0.0/foundation-icons.css'>
<link rel='stylesheet prefetch' href='https://cdnjs.cloudflare.com/ajax/libs/foundicons/3.0.0/svgs/fi-list.svg'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="app"></div> <script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react-with-addons.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react-dom.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
React Carousel - Script Codes CSS Codes
@import url("https://fonts.googleapis.com/css?family=Lobster");
body { background-color: #89FAD0; font-family: 'Lobster';
}
#carousel { position: absolute; height: 200px; width: 810px; margin: auto; left: 0; right: 0; top: 0; bottom: 0;
}
.arrow { position: absolute; width: 30px; height: 30px; background-color: white; text-align: center; font-size: 25px; border-radius: 50%; cursor: pointer; font-size: 20px; color: #228291; line-height: 30px; margin-top: 85px; z-index: 1000;
}
.arrow-right { left: 780px;
}
.item { text-align: center; color: white; font-size: 40px; position: absolute; transition: height 1s, width 1s, left 1s, margin-top 1s, line-height 1s, background-color 1s;
}
.level-2 { height: 150px; width: 110px; line-height: 150px; background-color: #228291; left: 650px; margin-top: 25px;
}
.level-1 { height: 180px; width: 130px; line-height: 180px; background-color: #6796E5; left: 500px; margin-top: 10px;
}
.level0 { height: 200px; width: 150px; line-height: 200px; background-color: #4EC9E1; left: 330px;
}
.level1 { height: 180px; width: 130px; line-height: 180px; background-color: #6796E5; margin-top: 10px; left: 180px;
}
.level2 { height: 150px; width: 110px; line-height: 150px; background-color: #228291; margin-top: 25px; left: 50px;
}
.left-enter { opacity: 0; left: -60px; height: 120px; width: 90px; line-height: 120px; margin-top: 40px;
}
.left-enter.left-enter-active { opacity: 1; left: 50px; height: 150px; width: 110px; line-height: 150px; margin-top: 25px; transition: left 1s, opacity 1s, height 1s, width 1s, margin-top 1s, line-height 1s;
}
.left-leave { opacity: 1; left: 650px; height: 150px; width: 110px; line-height: 150px; margin-top: 25px;
}
.left-leave.left-leave-active { left: 780px; opacity: 0; height: 120px; line-height: 120px; margin-top: 40px; width: 90px; transition: left 1s, opacity 1s, height 1s, width 1s, margin-top 1s, line-height 1s;
}
.right-enter { opacity: 0; left: 760px; height: 120px; width: 90px; line-height: 120px; margin-top: 40px;
}
.right-enter.right-enter-active { left: 650px; opacity: 1; height: 150px; margin-top: 25px; line-height: 150px; width: 110px; transition: left 1s, opacity 1s, height 1s, width 1s, margin-top 1s, line-height 1s;
}
.right-leave { left: 50px; height: 150px; opacity: 1; margin-top: 25px; line-height: 150px; width: 110px;
}
.right-leave.right-leave-active { left: -60px; opacity: 0; height: 120px; width: 90px; line-height: 120px; margin-top: 40px; transition: left 1s, opacity 1s, height 1s, width 1s, margin-top 1s, line-height 1s;
}
.noselect { -webkit-user-select: none; -khtml-user-select: none; -moz-user-select: none; -ms-user-select: none; user-select: none;
}
React Carousel - Script Codes JS Codes
'use strict';
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var ReactCSSTransitionGroup = React.addons.CSSTransitionGroup;
var Carousel = function (_React$Component) { _inherits(Carousel, _React$Component); function Carousel(props) { _classCallCheck(this, Carousel); var _this = _possibleConstructorReturn(this, _React$Component.call(this, props)); _this.state = { items: _this.props.items, active: _this.props.active, direction: '' }; _this.rightClick = _this.moveRight.bind(_this); _this.leftClick = _this.moveLeft.bind(_this); return _this; } Carousel.prototype.generateItems = function generateItems() { var items = []; var level; console.log(this.state.active); for (var i = this.state.active - 2; i < this.state.active + 3; i++) { var index = i; if (i < 0) { index = this.state.items.length + i; } else if (i >= this.state.items.length) { index = i % this.state.items.length; } level = this.state.active - i; items.push(React.createElement(Item, { key: index, id: this.state.items[index], level: level })); } return items; }; Carousel.prototype.moveLeft = function moveLeft() { var newActive = this.state.active; newActive--; this.setState({ active: newActive < 0 ? this.state.items.length - 1 : newActive, direction: 'left' }); }; Carousel.prototype.moveRight = function moveRight() { var newActive = this.state.active; this.setState({ active: (newActive + 1) % this.state.items.length, direction: 'right' }); }; Carousel.prototype.render = function render() { return React.createElement( 'div', { id: 'carousel', className: 'noselect' }, React.createElement( 'div', { className: 'arrow arrow-left', onClick: this.leftClick }, React.createElement('i', { className: 'fi-arrow-left' }) ), React.createElement( ReactCSSTransitionGroup, { transitionName: this.state.direction }, this.generateItems() ), React.createElement( 'div', { className: 'arrow arrow-right', onClick: this.rightClick }, React.createElement('i', { className: 'fi-arrow-right' }) ) ); }; return Carousel;
}(React.Component);
var Item = function (_React$Component2) { _inherits(Item, _React$Component2); function Item(props) { _classCallCheck(this, Item); var _this2 = _possibleConstructorReturn(this, _React$Component2.call(this, props)); _this2.state = { level: _this2.props.level }; return _this2; } Item.prototype.render = function render() { var className = 'item level' + this.props.level; return React.createElement( 'div', { className: className }, this.props.id ); }; return Item;
}(React.Component);
var items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
ReactDOM.render(React.createElement(Carousel, { items: items, active: 0 }), document.getElementById('app'));
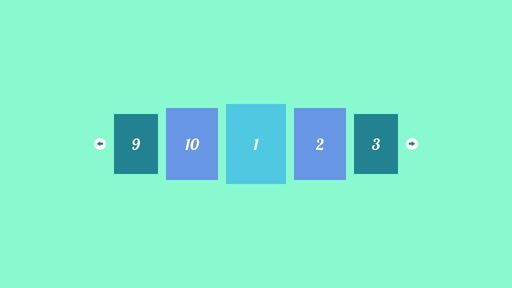
Developer | Andy Pagès |
Username | andyNroses |
Uploaded | November 10, 2022 |
Rating | 4.5 |
Size | 5,188 Kb |
Views | 36,432 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Fancy Rotate Menu - CSS Only | 2,701 Kb |
Fancy Animated Input Field | 7,995 Kb |
CSS Only Homer Simpson | 2,745 Kb |
Mr. Robot Animation | 3,719 Kb |
CSS Old Computer | 9,210 Kb |
Batman Joker Morphing | 6,300 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Replace url via jquery | Serluk | 1,429 Kb |
Simple Flat Menu | Jeplaa | 2,467 Kb |
Scroll using CSS | Casperovic | 2,159 Kb |
SVG email test v2.0 | M_J_Robbins | 2,090 Kb |
Sticky Navbar | Phantomesse | 5,106 Kb |
Custom Checkbox and radio inputs SCSS | Rgfx | 3,367 Kb |
Plotting Points with D3.js | Laurakelly | 31,996 Kb |
HTML5 Video Autoplay | Zivcos | 1,352 Kb |
CSS3 Form Page Design | Rssatnam | 3,613 Kb |
Double box-shadow | Daubac402 | 1,436 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!