React dropdown
How do I make an react dropdown?
What is a react dropdown? How do you make a react dropdown? This script and codes were developed by Dave on 25 November 2022, Friday.
React dropdown - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>react dropdown</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="root"></div> <script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.6.1/react.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.6.1/react-dom.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
React dropdown - Script Codes CSS Codes
/* * Settings */
body { color: #777; font-family: sans-serif; padding: 2rem;
}
/* * 1. So dropdown child is correct width. * 2. To allow dropdown to be positioned absolutely */
.c-dropdown-select { display: inline-block; font-size: 0.875rem; position: relative;
}
/* * 1. To position caret icon * 2. Button default is to center text * 3. Truncate For long labels */
.c-dropdown-select__trigger { cursor: pointer; display: block; font-size: inherit; line-height: 1; overflow: hidden; padding-bottom: 0.5rem; padding-left: 0.75rem; padding-right: 2rem; padding-top: 0.5rem; position: relative; text-align: left; text-overflow: ellipsis; white-space: nowrap; /* * The caret icon */
}
.c-dropdown-select__trigger:focus { border-color: #0288D1; outline: 0;
}
.c-dropdown-select__trigger::after { border: 0.5rem solid transparent; border-top-color: #777; content: ''; margin-top: -0.125rem; position: absolute; right: 0.5rem; top: 50%;
}
.c-dropdown-select__trigger-inner { -webkit-box-align: center; -ms-flex-align: center; align-items: center; display: -webkit-box; display: -ms-flexbox; display: flex;
}
.c-dropdown-select__trigger,
.c-dropdown-select__dropdown { background-color: #fff; border: 1px solid #ccc; border-radius: 3px; min-width: 13rem;
}
.c-dropdown-select__dropdown { left: 0; margin-top: 0.125rem; max-width: 15rem; position: absolute; top: 100%;
}
/* * 1. To align bullet and text */
.c-dropdown-select__option { -webkit-box-align: baseline; -ms-flex-align: baseline; align-items: baseline; cursor: pointer; display: -webkit-box; display: -ms-flexbox; display: flex; line-height: 1.4; padding: 0.5rem 0.75rem;
}
.c-dropdown-select__option.is-active, .c-dropdown-select__option:hover { background-color: #f1f1f1;
}
/* * 1. Takes up remaining space * 2. Allow pointer events to pass through */
.c-dropdown-select__option-text { -webkit-box-flex: 1; -ms-flex: 1; flex: 1; margin-left: 0.5rem; pointer-events: none;
}
/* * The circle icon */
/* * 1. Makes a perfect circle when height and width are set. */
.c-status-icon { background-color: #f1f1f1; border-radius: 50%; height: 0.75rem; width: 0.75rem;
}
.c-status-icon--red { background-color: #c62828;
}
.c-status-icon--orange { background-color: #F4511E;
}
.c-status-icon--green { background-color: #388E3C;
}
.c-status-icon--blue { background-color: #0288D1;
}
React dropdown - Script Codes JS Codes
'use strict';
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
// Constants
var KEYCODE = Object.freeze({ up: 38, down: 40, enter: 13, tab: 9
});
// Seed data
var data = [{ id: '1', value: 1, displayText: 'Option 1', color: 'green'
}, { id: '2', value: 2, displayText: 'Option 2 - I am some super long option text though', color: 'blue'
}, { id: '3', value: 3, displayText: 'Option 3', color: 'orange'
}, { id: '4', value: 4, displayText: 'Option 4', color: 'red'
}];
/** * Borrowed `ClickOutside` from my old CM code */
var ClickOutside = function (_React$Component) { _inherits(ClickOutside, _React$Component); function ClickOutside(props) { _classCallCheck(this, ClickOutside); var _this = _possibleConstructorReturn(this, _React$Component.call(this, props)); _this.invokeCallback = _this.invokeCallback.bind(_this); _this.preventClose = _this.preventClose.bind(_this); return _this; } ClickOutside.prototype.componentDidMount = function componentDidMount() { this.bindEvents(); }; ClickOutside.prototype.componentWillUnmount = function componentWillUnmount() { this.unbindEvents(); }; ClickOutside.prototype.bindEvents = function bindEvents() { window.addEventListener('mousedown', this.invokeCallback, false); window.addEventListener('touchstart', this.invokeCallback, false); }; ClickOutside.prototype.unbindEvents = function unbindEvents() { window.removeEventListener('mousedown', this.invokeCallback); window.removeEventListener('touchstart', this.invokeCallback); }; ClickOutside.prototype.invokeCallback = function invokeCallback(e) { var _props = this.props; var callback = _props.callback; var excludeId = _props.excludeId; if (e.target && e.target.id !== excludeId) { callback(); } }; ClickOutside.prototype.preventClose = function preventClose(e) { e.stopPropagation(); }; ClickOutside.prototype.render = function render() { var children = this.props.children; return React.createElement( 'div', { className: 'c-click-outside', onMouseDown: this.preventClose, onTouchStart: this.preventClose, role: 'presentation' }, children ); }; return ClickOutside;
}(React.Component);
// Dumb / presentational components
var StatusIcon = function StatusIcon(_ref) { var color = _ref.color; return React.createElement('span', { 'aria-hidden': 'true', className: 'c-status-icon ' + (color ? 'c-status-icon--' + color : '') });
};
var Trigger = function Trigger(_ref2) { var _ref2$displayText = _ref2.displayText; var displayText = _ref2$displayText === undefined ? 'Please select' : _ref2$displayText; var color = _ref2.color; var value = _ref2.value; var controls = _ref2.controls; var isDropdownOpen = _ref2.isDropdownOpen; var isFocused = _ref2.isFocused; var props = _objectWithoutProperties(_ref2, ['displayText', 'color', 'value', 'controls', 'isDropdownOpen', 'isFocused']); // Adds a ref to expose the native DOM `.focus()` method when required var ref = { ref: isFocused ? function (button) { if (isFocused && button) button.focus(); } : null }; return React.createElement( 'button', _extends({}, ref, props, { 'aria-controls': controls, 'aria-expanded': isDropdownOpen, className: 'c-dropdown-select__trigger' }), React.createElement( 'span', { className: 'c-dropdown-select__trigger-inner' }, React.createElement(StatusIcon, { color: color }), React.createElement( 'span', { className: 'c-dropdown-select__option-text' }, displayText ) ) );
};
var Option = function Option(_ref3) { var isHighlighted = _ref3.isHighlighted; var color = _ref3.color; var displayText = _ref3.displayText; var props = _objectWithoutProperties(_ref3, ['isHighlighted', 'color', 'displayText']); return React.createElement( 'li', _extends({}, props, { 'aria-selected': isHighlighted ? true : null, className: 'c-dropdown-select__option ' + (isHighlighted ? 'is-active' : ''), role: 'option' }), React.createElement(StatusIcon, { color: color }), React.createElement( 'span', { className: 'c-dropdown-select__option-text' }, displayText ) );
};
var Dropdown = function Dropdown(_ref4) { var activeId = _ref4.activeId; var handleClick = _ref4.handleClick; var highlightedIndex = _ref4.highlightedIndex; var id = _ref4.id; var options = _ref4.options; return React.createElement( 'ul', { 'aria-activedescendant': activeId, className: 'c-dropdown-select__dropdown', id: id, role: 'listbox', tabindex: '0' }, options.map(function (item, i) { return React.createElement(Option, _extends({}, item, { isHighlighted: highlightedIndex === i, onClick: handleClick })); }) );
};
// Smart components
var App = function (_React$Component2) { _inherits(App, _React$Component2); function App(props) { _classCallCheck(this, App); var _this2 = _possibleConstructorReturn(this, _React$Component2.call(this)); _this2.state = { isDropdownOpen: false, selectedOption: {}, highlightedIndex: 0, returnFocus: false }; _this2.handleUpKey = _this2.handleUpKey.bind(_this2); _this2.handleTrigger = _this2.handleTrigger.bind(_this2); _this2.handleSelect = _this2.handleSelect.bind(_this2); _this2.handleDownKey = _this2.handleDownKey.bind(_this2); _this2.handleEnterKey = _this2.handleEnterKey.bind(_this2); _this2.handleKeyDown = _this2.handleKeyDown.bind(_this2); _this2.closeDropdown = _this2.closeDropdown.bind(_this2); return _this2; } App.prototype.handleUpKey = function handleUpKey() { var idx = this.state.highlightedIndex; this.setState({ highlightedIndex: idx === 0 ? 0 : idx - 1 }); }; App.prototype.handleTrigger = function handleTrigger() { this.setState({ isDropdownOpen: !this.state.isDropdownOpen, highlightedIndex: 0 }); }; App.prototype.handleSelect = function handleSelect(e) { var appData = this.props.appData; var id = e.target.id; var selectedOption = appData.filter(function (item) { return item.id === id; })[0]; this.setState({ selectedOption: selectedOption, isDropdownOpen: false, returnFocus: true }); }; App.prototype.closeDropdown = function closeDropdown() { this.setState({ isDropdownOpen: false, highlightedIndex: 0 }); }; App.prototype.handleDownKey = function handleDownKey() { var appData = this.props.appData; var idx = this.state.highlightedIndex; var max = appData.length - 1; this.setState({ highlightedIndex: idx === max ? max : idx + 1 }); }; App.prototype.handleEnterKey = function handleEnterKey(e) { if (this.state.isDropdownOpen) { e.preventDefault(); var appData = this.props.appData; this.setState({ selectedOption: appData[this.state.highlightedIndex], isDropdownOpen: false, highlightedIndex: 0 }); } }; App.prototype.handleKeyDown = function handleKeyDown(e) { var _this3 = this, _keyMap; var keyMap = (_keyMap = {}, _keyMap[KEYCODE.down] = this.handleDownKey, _keyMap[KEYCODE.enter] = function (evt) { return _this3.handleEnterKey(evt); }, _keyMap[KEYCODE.up] = this.handleUpKey, _keyMap[KEYCODE.tab] = this.closeDropdown, _keyMap); if (keyMap[e.keyCode]) { keyMap[e.keyCode](e); } }; App.prototype.render = function render() { var _state = this.state; var highlightedIndex = _state.highlightedIndex; var isDropdownOpen = _state.isDropdownOpen; var selectedOption = _state.selectedOption; var returnFocus = _state.returnFocus; var _props2 = this.props; var appData = _props2.appData; var id = _props2.id; return React.createElement( ClickOutside, { callback: this.closeDropdown }, React.createElement( 'div', { className: 'c-dropdown-select' }, React.createElement(Trigger, _extends({}, selectedOption, { controls: id, isDropdownOpen: isDropdownOpen, isFocused: returnFocus, onClick: this.handleTrigger, onKeyDown: this.handleKeyDown })), isDropdownOpen ? React.createElement(Dropdown, { activeId: appData[highlightedIndex].id, handleClick: this.handleSelect, highlightedIndex: highlightedIndex, id: id, options: appData }) : null ) ); }; return App;
}(React.Component);
;
ReactDOM.render(React.createElement(App, { appData: data, id: 'id_super_dropdown' }), document.getElementById('root'));
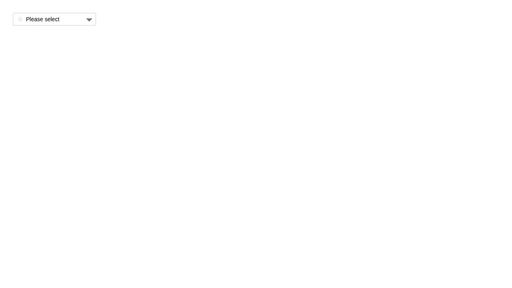
Developer | Dave |
Username | DaveOrDead |
Uploaded | November 25, 2022 |
Rating | 3 |
Size | 8,538 Kb |
Views | 22,264 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Toggle component | 29,331 Kb |
Scrollable tables | 1,926 Kb |
Shell CSS lib | 43,289 Kb |
React Tic Tac Toe | 28,062 Kb |
Flexbox grid mark 2 | 2,131 Kb |
Password validation | 3,866 Kb |
Callbacks | 1,471 Kb |
Webpack config | 1,978 Kb |
Flexbox Grid - equal height | 2,855 Kb |
Callbacks 2 - Passing arguments | 1,496 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Magnus 3 | ARocketfish | 7,944 Kb |
Rainbow Drops | Csbarnes | 2,365 Kb |
Display properties | Hamzaerbay | 1,886 Kb |
Faces SVG animation | ScavengerFrontend | 2,957 Kb |
Process Accordion | Devilskitchen | 31,432 Kb |
Fixed Scrolling Nav Bar | Philsinatra | 0 Kb |
SVG Basics | HipsterBrown | 1,852 Kb |
Mobile first social buttons with no iframe | Alistairtweedie | 3,158 Kb |
CSS Grid Test | Ajaykarwal | 2,377 Kb |
BenU Maintenance Site | Ksherman | 4,893 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!