React konva
How do I make an react konva?
What is a react konva? How do you make a react konva? This script and codes were developed by Victoria on 01 December 2022, Thursday.
React konva - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>React konva</title>
</head>
<body> <div id='container'></div> <script src='https://rawgit.com/konvajs/konva/master/konva.js'></script>
<script src='https://rawgit.com/lavrton/react-konva/master/dist/react-konva.bundle.js'></script> <script src="js/index.js"></script>
</body>
</html>
React konva - Script Codes JS Codes
'use strict';
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var _ReactKonva = ReactKonva;
var Layer = _ReactKonva.Layer;
var Rect = _ReactKonva.Rect;
var Stage = _ReactKonva.Stage;
var Group = _ReactKonva.Group;
var ConvaImg = _ReactKonva.Image;
var imageSrc = 'http://coolwildlife.com/wp-content/uploads/galleries/post-3004/Fox%20Picture%20003.jpg';
var Resizer = function (_React$Component) { _inherits(Resizer, _React$Component); function Resizer() { _classCallCheck(this, Resizer); var _this = _possibleConstructorReturn(this, _React$Component.call(this)); _this.state = { x: 10, y: 10, width: 300, height: 200, loading: true }; return _this; } Resizer.prototype.componentWillReceiveProps = function componentWillReceiveProps(_ref) { var url = _ref.url; var prevUrl = this.props.prevUrl; if (url !== prevUrl) { this.updateImg(url); } }; Resizer.prototype.componentDidMount = function componentDidMount() { this.updateImg(this.props.url); }; Resizer.prototype.updateImg = function updateImg(url) { var _this2 = this; this.img = new Image(); this.img.src = url; this.setState({ loading: true }); this.img.onload = function () { var _img = _this2.img; var width = _img.width; var height = _img.height; _this2.setState({ loading: false, imageWidth: width, imageHeight: height }); }; }; Resizer.prototype.handles = function handles() { var handleSize = 10; var handleOffset = -handleSize / 2; var _state = this.state; var x = _state.x; var y = _state.y; var width = _state.width; var height = _state.height; var xs = [x, x + width / 2, x + width]; var ys = [y, y + height / 2, y + height]; return [[0, 0], [1, 0], [2, 0], [2, 1], [2, 2], [1, 2], [0, 2], [0, 1]].map(function (_ref2) { var ix = _ref2[0]; var iy = _ref2[1]; return { x: xs[ix] + handleOffset, y: ys[iy] + handleOffset, width: handleSize, height: handleSize, ix: ix, iy: iy }; }); }; Resizer.prototype.imageWithRatio = function imageWithRatio() { var _state2 = this.state; var imageHeight = _state2.imageHeight; var imageWidth = _state2.imageWidth; var frameRatio = this.state.height / this.state.width; var ratio = imageHeight / imageWidth; var fromWidth = this.state.width * ratio > this.state.height; var width = fromWidth ? imageWidth : imageHeight / frameRatio; var height = fromWidth ? imageWidth * frameRatio : imageHeight; var offsetY = (imageHeight - height) / 2; var offsetX = (imageWidth - width) / 2; return [offsetX, offsetY, width, height, this.state.x, this.state.y, this.state.width, this.state.height]; }; Resizer.prototype.resize = function resize(pos, rect, handle) { var x = handle.x; var y = handle.y; var ix = handle.ix; var iy = handle.iy; var _state3 = this.state; var width = _state3.width; var height = _state3.height; var scaleX = ix - 1; var scaleY = iy - 1; var dx = pos.x - rect.getAbsolutePosition().x; var dy = pos.y - rect.getAbsolutePosition().y; this.setState({ width: Math.max(scaleX * dx + this.state.width, 0), height: Math.max(scaleY * dy + this.state.height, 0), x: -Math.min(scaleX, 0) * dx + this.state.x, y: -Math.min(scaleY, 0) * dy + this.state.y }); }; Resizer.prototype.render = function render() { var _this3 = this; return React.createElement( Group, { draggable: 'true' }, React.createElement(ConvaImg, { ref: function ref(r) { // rewrite konvajs sceneFunc function to change canvas drawImage arguments if (r) { r.sceneFunc(function (context) { context.drawImage.apply(context, [_this3.img].concat(_this3.imageWithRatio())); }); } }, x: 0, y: 0, image: this.img //width={this.state.width} //height={this.state.height} }), this.handles().map(function (handle) { var _ref3 = undefined; return React.createElement(Rect, { ref: function ref(r) { return _ref3 = r; }, x: handle.x, y: handle.y, width: handle.width, height: handle.height, fill: 'blue', draggable: 'true', dragBoundFunc: function dragBoundFunc(pos) { _this3.resize(pos, _ref3, handle); // additionally prevent changing coords for some axis return { x: handle.ix === 1 ? _ref3.getAbsolutePosition().x : pos.x, y: handle.iy === 1 ? _ref3.getAbsolutePosition().y : pos.y }; } }); }) ); }; return Resizer;
}(React.Component);
function App() { return React.createElement( Stage, { width: 800, height: 600 }, React.createElement( Layer, null, React.createElement(Resizer, { url: imageSrc }) ) );
}
ReactDOM.render(React.createElement(App, null), document.getElementById('container'));
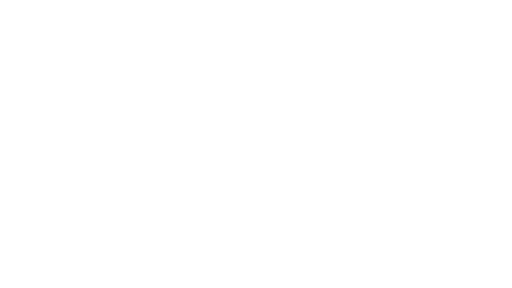
Developer | Victoria |
Username | Enieste |
Uploaded | December 01, 2022 |
Rating | 3 |
Size | 4,721 Kb |
Views | 76,912 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
FCC Tribute page | 3,327 Kb |
FCC Recipe Box | 7,585 Kb |
Profile | 3,970 Kb |
FCC Game of Life | 4,303 Kb |
D3 paths | 9,943 Kb |
FCC Random quote machine | 2,085 Kb |
React TODO | 3,320 Kb |
FCC Leaderboard | 3,665 Kb |
FCC Tic Tac Toe | 5,602 Kb |
FCC Local Weather | 2,076 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Tab Menus | Zephyr | 3,180 Kb |
Scroll to view if element partially out of view port height | ChrisMaki | 2,104 Kb |
Responsive Minimal Blog Layout | Hackthevoid | 5,261 Kb |
Nav Test -- cats 1 | Payls | 4,735 Kb |
Bubble animation | Ftabor | 6,565 Kb |
Vue.js Starter | Andymerskin | 1,268 Kb |
Buttons with style | Chbymnky | 2,082 Kb |
Vertically rotating text with CSS | Nopr | 2,141 Kb |
DevCamp 2014 - Denver Public Library | See8ch | 5,033 Kb |
RPG Style Text Dialogue | Odylic | 2,635 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!