React Weather App
How do I make an react weather app?
Weather forecast for Portland made with React and OpenWeatherMap.org as part of an interview project.. What is a react weather app? How do you make a react weather app? This script and codes were developed by Sarah Dunlap on 18 January 2023, Wednesday.
React Weather App - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>React Weather App</title> <link rel='stylesheet prefetch' href='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-beta/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="container"> <div class="appTitle"> <h2>Weather Forecast</h2> </div>
<div id="weatherApp">
</div>
</div> <script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.6.1/react.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.6.1/react-dom.min.js'></script>
<script src='https://npmcdn.com/[email protected]/dist/ReactNative.js'></script> <script src="js/index.js"></script>
</body>
</html>
React Weather App - Script Codes CSS Codes
body { background-color: #90A4AE;
}
.container { text-align: center;
}
.appTitle { background-color: #fff; margin-top: 20px; padding: 15px; max-width: 400px; min-width: 200px; margin-left: auto; margin-right: auto; border-radius: 5px;
}
#weatherApp { padding: 40px;
}
.weatherColumn { padding: 20px; margin-left: auto; margin-right: auto; width: 200px;
}
.card { min-width: 200px;
}
React Weather App - Script Codes JS Codes
"use strict";
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var WeatherInfo = function (_React$Component) { _inherits(WeatherInfo, _React$Component); function WeatherInfo() { _classCallCheck(this, WeatherInfo); var _this = _possibleConstructorReturn(this, _React$Component.call(this)); _this.state = { weatherData: [], result: [] }; return _this; } WeatherInfo.prototype.componentDidMount = function componentDidMount() { var _this2 = this; fetch('https://api.openweathermap.org/data/2.5/forecast?q=Portland,Oregon&units=imperial&apikey=5282c7acc7cee8e3debc6f2733c2bb95&mode=json').then(function (results) { return results.json(); }).then(function (data) { _this2.setState({ weatherData: data.list }); _this2.setState({ result: _this2.processData(data.list) }); }); }; WeatherInfo.prototype.processData = function processData(weatherData) { var weatherArray = weatherData, weatherArrayLen = weatherArray.length, result = [], weekDaysArray = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"], processWeatherObj = {}, processWeatherKeys = [], today = new Date().getDay(); var date = '', dayOfWeek = '', processWeatherKeysLen = 0, currentDayData = undefined, weatherForTime = {}, descriptionText = undefined, descriptionArrayToCompare = [], averageTemp = undefined, icon = undefined, averageTempLen = undefined; for (var i = 0; i < weatherArrayLen; i++) { weatherForTime = weatherArray[i]; //get day and month to keep track of all values for day and month date = weatherArray[i].dt_txt.slice(0, 10); if (!processWeatherObj[date]) { //keep track of keys for later since I'm already looping through processWeatherKeys.push(date); dayOfWeek = new Date(weatherArray[i].dt_txt).getDay(); if (today === dayOfWeek) { dayOfWeek = "Today"; } else { dayOfWeek = weekDaysArray[dayOfWeek]; } processWeatherObj[date] = { temp: [], minTemp: weatherForTime.main.temp_min, maxTemp: weatherForTime.main.temp_max, description: [], day: dayOfWeek, icon: [] }; } //create arrays to get average temp for the day and average description processWeatherObj[date].temp.push(weatherForTime.main.temp); processWeatherObj[date].description.push(weatherForTime.weather[0].description); processWeatherObj[date].icon.push(weatherForTime.weather[0].icon); //Check if the min Temp and max Temp are true for the day processWeatherObj[date].minTemp = processWeatherObj[date].minTemp < weatherForTime.main.temp_min ? processWeatherObj[date].minTemp : weatherForTime.main.temp_min; processWeatherObj[date].maxTemp = processWeatherObj[date].maxTemp > weatherForTime.main.temp_max ? processWeatherObj[date].maxTemp : weatherForTime.main.temp_max; } //process results processWeatherKeysLen = processWeatherKeys.length; for (var k = 0; k < processWeatherKeysLen; k++) { currentDayData = processWeatherObj[processWeatherKeys[k]]; //get average temp & description info averageTempLen = currentDayData.temp.length; averageTemp = 0; descriptionArrayToCompare = []; for (var j = 0; j < averageTempLen; j++) { averageTemp += currentDayData.temp[j]; var indexOfDescriptionArrayToCompare = descriptionArrayToCompare.indexOf(currentDayData.description[j]); if (indexOfDescriptionArrayToCompare === -1) { descriptionArrayToCompare.push(currentDayData.description[j]); descriptionArrayToCompare.push(1); descriptionArrayToCompare.push(currentDayData.icon[j]); } else { descriptionArrayToCompare[indexOfDescriptionArrayToCompare + 1] += 1; } } if (descriptionArrayToCompare.length > 3) { var mostDesc = descriptionArrayToCompare[1] < descriptionArrayToCompare[4] ? descriptionArrayToCompare[3] : descriptionArrayToCompare[0]; var leastDesc = descriptionArrayToCompare[1] < descriptionArrayToCompare[4] ? descriptionArrayToCompare[0] : descriptionArrayToCompare[3]; icon = descriptionArrayToCompare[1] < descriptionArrayToCompare[4] ? descriptionArrayToCompare[5] : descriptionArrayToCompare[2]; icon = "https://openweathermap.org/img/w/" + icon + ".png"; descriptionText = "Mostly " + mostDesc + " with " + leastDesc; } else { descriptionText = descriptionArrayToCompare[0]; icon = "https://openweathermap.org/img/w/" + descriptionArrayToCompare[2] + ".png"; } result.push(React.createElement( "div", { className: "card card-outline-secondary mb-3 text-center" }, React.createElement( "div", { className: "card-block" }, React.createElement( "div", { className: "row" }, React.createElement( "div", { className: "col-4-md weatherColumn" }, React.createElement( "h3", null, currentDayData.day ), React.createElement( "p", null, descriptionText ) ), React.createElement( "div", { className: "col-3-md offset-1 weatherColumn" }, React.createElement( "h4", null, React.createElement( "strong", null, "Average: ", Math.floor(averageTemp / averageTempLen) ) ), React.createElement("img", { src: icon }) ), React.createElement( "div", { className: "col-4-md offset-1 weatherColumn" }, React.createElement( "p", null, "Max: ", currentDayData.maxTemp ), React.createElement( "p", null, "Min: ", currentDayData.minTemp ) ) ) ) )); } return result; }; WeatherInfo.prototype.render = function render() { return React.createElement( "p", null, this.state.result ); }; return WeatherInfo;
}(React.Component);
var App = function (_React$Component2) { _inherits(App, _React$Component2); function App() { _classCallCheck(this, App); return _possibleConstructorReturn(this, _React$Component2.apply(this, arguments)); } App.prototype.render = function render() { return React.createElement( "div", null, React.createElement(WeatherInfo, null) ); }; return App;
}(React.Component);
;
ReactDOM.render(React.createElement(App, null), weatherApp);
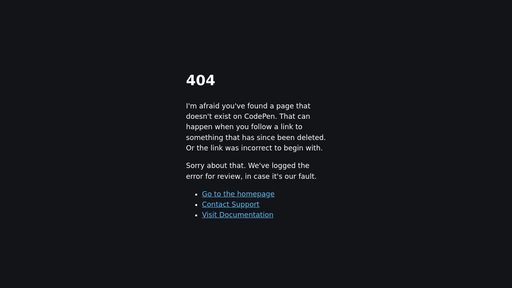
Developer | Sarah Dunlap |
Username | SarahDunlap |
Uploaded | January 18, 2023 |
Rating | 3 |
Size | 5,656 Kb |
Views | 8,096 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Handy Tool For Work | 4,308 Kb |
Wikipedia Search | 2,382 Kb |
Work in Progress | 3,337 Kb |
My CodePen Projects | 3,111 Kb |
Card Game | 2,804 Kb |
JavaScript Calculator | 2,739 Kb |
Cat Clicker | 3,050 Kb |
Rotate Circle | 1,963 Kb |
Tribute | 2,531 Kb |
Pomodoro Clock | 2,695 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Profile box | Daniesy | 2,766 Kb |
TheCalendar.js | The-teacher | 6,330 Kb |
SVG Text Masking | JMChristensen | 2,141 Kb |
CSS 3D Radio buttons | Andreasnylin | 1,650 Kb |
Barber Shop | Bhlaird | 6,270 Kb |
Animate elements with fixed gradient | Badabam | 4,406 Kb |
FreeCodeCamp - Simon Game | Ivhed | 8,481 Kb |
Two joint circles - One element | Berdejitendra | 1,704 Kb |
Alter bg opacity on hover... | Chrisboon27 | 2,054 Kb |
Two tables and header with jspdf-autotable | Someatoms | 2,245 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!