SCSS z-index
How do I make an scss z-index?
Function and mixin to handle z-index values across your project. What is a scss z-index? How do you make a scss z-index? This script and codes were developed by Jakob-e on 04 July 2022, Monday.
SCSS z-index - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>SCSS z-index</title> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <link rel='stylesheet prefetch' href='css/bnnmjd.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <article> <header> <h1>SCSS z-index</h2> <p>Did you ever do a "z-index: 999;"? If so you might need something like this... <p>Rather than typing the z-index value in your classes simply make a reference to a centralized list or map and take control of all your elements in one place.</p> </header> <section> <h2>Value based</h2> <p>If you prefer defining each z-index individually define your z-layers as a map.</p> <div data-ace-theme="tomorrow" data-ace-mode="scss" data-ace-cursor="false">
//
// Value based (map)
//
$z-layers: ( 'wallpaper' : 0 , 'page-content' : 1 , 'page-footer' : 2 , 'page-header' : 2 , 'page-navigation' : 3 , 'modal-window' : 4
);
// Use it
.class { z-index: z('modal-window'); } // => z-index: 4
.class { @include z('modal-window'); } // => z-index: 4 </div> </section> <section> <h2>Index based</h2> <p>If you don't care about specific z-index values – define your z-layers as a list. When requested the index is returned – making the first item the lowest.</p> <div data-ace-theme="tomorrow" data-ace-mode="scss" data-ace-cursor="false">//
// Index based (top is lower)
//
$z-layers: ( 'wallpaper' , 'page-content' , 'page-footer' , 'page-header' , 'page-navigation' , 'modal-window'
);
// Use it
.class { z-index: z('modal-window'); } // => z-index: 6
.class { @include z('modal-window'); } // => z-index: 6 </div> </section> <section> <h2>Function and Mixin</h2> <div data-ace-theme="tomorrow" data-ace-mode="scss" data-ace-cursor="false">//
// Function and mixin to return the z-index
// from $z-layers
//
@function z-index($name){ $z: null; @if type-of($z-layers) == map { $z: map-get($z-layers, $name); } @if type-of($z-layers) == list { @for $i from 1 through length($z-layers) { @if nth($z-layers, $i) == $name { $z: $i; } } } @if $z { @return $z; } @else { @error('Could not find a z-index for `#{$name}`'); }
}
@mixin z-index($name){ z-index: z-index($name); }
// Short hands – if you are lazy ;-)
@function z($arglist...){ @return z-index($arglist...); }
@mixin z($arglist...){ @include z-index($arglist...); } </div> </section> <footer data-related-pens="KpdBzY"></footer>
</article> <script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/ace/1.1.3/ace.js'></script>
<script src='http://codepen.io/jakob-e/pen/MwKXbV.js'></script> <script src="js/index.js"></script>
</body>
</html>
SCSS z-index - Script Codes CSS Codes
// Google Font
// http://codepen.io/jakob-e/pen/LVVXjO
// Source: // codepen.io/jakob-e/pen/BNNMjd
@include google-font(Lato, 300 400);
@function z-index($name){ $z: null; @if type-of($z-layers) == map { $z: map-get($z-layers, $name); } @if type-of($z-layers) == list { @for $i from 1 through length($z-layers) { @if nth($z-layers, $i) == $name { $z: $i; } } } @if $z { @return $z; } @else { @error('Could not find a z-index for `#{$name}`'); }
}
@mixin z-index($name){ z-index: z-index($name); }
// Short hands
@function z($arglist...){ @return z-index($arglist...); }
@mixin z($arglist...){ @include z-index($arglist...); }
//
// Value based
//
$z-layers:( 'wallpaper' : 0 , 'page-content' : 1 , 'page-footer' : 2 , 'page-header' : 2 , 'page-navigation' : 3 , 'modal-window' : 4
);
/* Map */
.class { z-index: z('modal-window'); } // => z-index: 4
.class { @include z('modal-window'); } // => z-index: 4
//
// Index based (top is lower)
//
$z-layers:( 'wallpaper' , 'page-content' , 'page-footer' , 'page-header' , 'page-navigation' , 'modal-window'
);
/* List */
.class { z-index: z('modal-window'); } // => z-index: 6
.class { @include z('modal-window'); } // => z-index: 6
h1 { font: 300 36px 'Lato', sans-serif; color: #508cbc; }
h2 { font: 400 24px 'Lato', sans-serif; color: #508cbc; }
p { font: 300 16px 'Lato', sans-serif; color: #404040; }
strong { font: inherit; font-weight: 400; }
body { background: whitesmoke;}
article { display:block; max-width:800px; margin:0 auto; padding:20px 40px 40px; background: #fff; border-radius: 7px;
box-shadow:0px 0px 10px rgba(0,0,0,0.2); margin-bottom:100px;}
article { margin:-20px auto 0; max-width: 900px; border-radius: 7px; padding: 20px 40px 0; background: white; box-shadow: 0 5px 5px rgba(0, 0, 0, 0.2);
}
[data-related-pens] { background: darken(whitesmoke,20%); }
SCSS z-index - Script Codes JS Codes
//
// Editor: //cdnjs.cloudflare.com/ajax/libs/ace/1.1.3/ace.js
var theme='ace/theme/tomorrow';
var mode='ace/mode/scss';
var editors = document.querySelectorAll('[data-ace-mode]');
for(var i=0,l=editors.length;i<l;++i){ var editor= ace.edit(editors[i]); if(editors[i].getAttribute('data-ace-theme')){ editor.setTheme('ace/theme/'+editors[i].getAttribute('data-ace-theme')); } else { editor.setTheme(theme); } if(editors[i].getAttribute('data-ace-mode')){ editor.getSession().setMode('ace/mode/'+editors[i].getAttribute('data-ace-mode')); } else { editor.getSession().setMode(mode); } editor.renderer.setShowGutter(false); editor.setShowPrintMargin(false); editor.setShowPrintMargin(false); editor.setDisplayIndentGuides(false); editor.setOptions({ maxLines: Infinity, //readOnly: false, highlightActiveLine: false, highlightGutterLine: false });
}
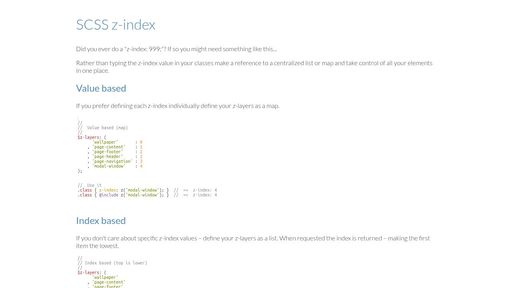
Developer | Jakob-e |
Username | jakob-e |
Uploaded | July 04, 2022 |
Rating | 4.5 |
Size | 7,745 Kb |
Views | 32,384 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Profile Top | 8,420 Kb |
Slide to Navigation | 3,063 Kb |
Angular toggle password directive | 4,356 Kb |
Snap to base-line | 4,943 Kb |
CSS Shapes | 14,064 Kb |
SVG Path Circle | 2,166 Kb |
Placeholder Services | 4,197 Kb |
Encode SVG SCSS | 14,772 Kb |
SVG Encoding | 3,280 Kb |
Baseline, Scale and Element Queries | 8,635 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Long Shadow Button | Uixcrazy | 3,550 Kb |
Light Switch | Bartuc | 4,933 Kb |
CodeCamp Tribute Page | JonathanDeJesus | 6,860 Kb |
Reviews and Ratings Star | Zbnmstry | 1,591 Kb |
Twitch API | Coderpilot | 3,412 Kb |
Angular-HAML | Cwacht | 2,022 Kb |
Html5-canvas-snow-effect | MariamMassadeh | 2,609 Kb |
NgEasyModal | Lorenzodianni | 4,159 Kb |
CSS Parent Selector | Tomhodgins | 2,143 Kb |
JQuery AJAX reddit Exercise | Btholt | 1,777 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!