Textbox Memory
How do I make an textbox memory?
This pen showcases memorizing the last couple of text entries typed, and allows you to view them again similar to Terminal or Command Prompt by hitting the UP arrow while having the textbox focused. (probably could optimize for speed a bit more and cleanup code atm). What is a textbox memory? How do you make a textbox memory? This script and codes were developed by Event Horizon on 30 July 2022, Saturday.
Textbox Memory - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Textbox Memory</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="container"> <h3>When you have the input focused, and have already entered text (hit enter), press the up arrow to cycle through the last 20 typed items!</h3>
</div>
<div class="container"> <input id="inp" type="text"/>
</div> <script src="js/index.js"></script>
</body>
</html>
Textbox Memory - Script Codes CSS Codes
*{ box-sizing:border-box;
}
html,body{ height:100%; /* FF3.6+ */
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,rgba(0,0,0,0)), color-stop(50%,rgba(0,0,0,1)), color-stop(100%,rgba(0,0,0,1))); /* Chrome,Safari4+ */
background: -webkit-linear-gradient(top, rgba(0,0,0,0) 0%,rgba(0,0,0,1) 50%,rgba(0,0,0,1) 100%); /* Chrome10+,Safari5.1+ */ /* Opera 11.10+ */ /* IE10+ */
background: linear-gradient(to bottom, rgba(0,0,0,0) 0%,rgba(0,0,0,1) 50%,rgba(0,0,0,1) 100%); /* W3C */
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#00000000', endColorstr='#000000',GradientType=0 ); /* IE6-9 */
}
h3{ display:block; font-size:1.25; font-weight:bold; padding:8px;
}
.container{ padding:8px; text-align:center; color:#000; text-shadow:1px 1px 0px #fff, -1px -1px 0px #fff, 1px 0px 0px #fff, 0px 1px 0px #fff, -1px 0px 0px #fff, 0px -1px 0px #fff;
}
.container input{ width:100%; color:black;
}
@media (max-height: 320px){ .container{ color:white; text-shadow:1px 1px 0px #000, -1px -1px 0px #000, 1px 0px 0px #000, 0px 1px 0px #000, -1px 0px 0px #000, 0px -1px 0px #000; }
}
Textbox Memory - Script Codes JS Codes
/* * * Event-Horizon * * * To Do: convert textMemory.shift() into 2 array system for performance *tempStack = [] *stack = [] * *tempstack.push(1) *tempstack.push(2) *tempstack.push(3) * *tempstack = 3, 2, 1 * *stack.push(tempstack.pop()) //add 3 *stack.push(tempstack.pop()) //add 2 *stack.push(tempstack.pop()) //add 1 * *stack.pop() // pop 1 *stack.pop() // pop 2 *stack.pop() // pop 3 * * *addToQueue(text) * tempstack.push(text) * for each num in tempstack * stack.push(tempstack.pop() * *stack.pop() //use to be stack.shift() optimized for speed * * */
document.addEventListener("DOMContentLoaded", function() { /** * addEntryMemory allows you to add short text memory to input/textarea boxes ala * Terminal / Command Prompt * * @method addEntryMemory * @param {Object} options An options object * @param {Number} options.maxMemory A number indicating maximum text to remember * @param {Number} options.memorySetTime A number in milliseconds for setting items, may want at 10 if using enter key * @param {Number} options.memoryGetTime A number in milliseconds for getting items, default is 600ms * @optional {Boolean} options.useEnter A boolean telling to check for enter key press before continuing, required if no callback function being used * @optional {Function} options.enterCallback A function that is called after setting the memory item but before clearing input */ Element.prototype.addEntryMemory = function(options) { var self = this, textMemory = [], UPARROWKEY = 38, ENTERKEY = 13, curPointer = textMemory.length - 1, setEntryTimeout = undefined, useEnter = options.useEnter || false, enterCallback = options.enterCallback || undefined, maxMemory = options.maxMemory || 10, memorySetTime = (options.memorySetTime&&(useEnter||enterCallback))? 10 : (options.memorySetTime)? options.memorySetTime : 600, memoryGetTime = options.memoryGetTime || 600; function setEntryMemory(text) { if(textMemory.length < maxMemory) { textMemory.push(text); curPointer = textMemory.length - 1; } else { textMemory.shift(); textMemory.push(text); curPointer = textMemory.length - 1; } } function getEntryMemory(elem) { //get last, cycle through elem.disabled = true; elem.value = "Previous entry was: "; window.setTimeout(function() { if(curPointer < 0) { //if pointer goes negative, set it back to end of array curPointer = textMemory.length - 1; } elem.disabled = false; elem.focus(); elem.value = textMemory[curPointer]; curPointer = curPointer - 1; }, memoryGetTime); } self.addEventListener("keyup", function(e) { e = e || window.event; if(e.keyCode == UPARROWKEY) { //if up arrow pressed if(document.activeElement === self) { //if input is focused getEntryMemory(self); } } else { if(useEnter || enterCallback && e.keyCode === ENTERKEY) { window.clearTimeout(setEntryTimeout); setEntryTimeout = window.setTimeout(function() { setEntryMemory(self.value); if(enterCallback && typeof enterCallback === "function") { enterCallback.call(self); } self.value = ""; }, memorySetTime); } else if(!useEnter && !enterCallback) { window.clearTimeout(setEntryTimeout); setEntryTimeout = window.setTimeout(function() { setEntryMemory(self.value); }, memorySetTime); } } }); }; //example usage below var input = document.getElementById("inp"); //change maxMemory to change amount of text saved, memorySetTime to 10 for enterKey interaction, enterCallback to log input value //if you do not provide an enterCallback function or useEnter set to true, it will save everything you type on keyup, and its based on the memorySetTime speed in milliseconds input.addEntryMemory({ maxMemory: 20, memorySetTime: 10, enterCallback: function() { console.log(this.value); } });
});
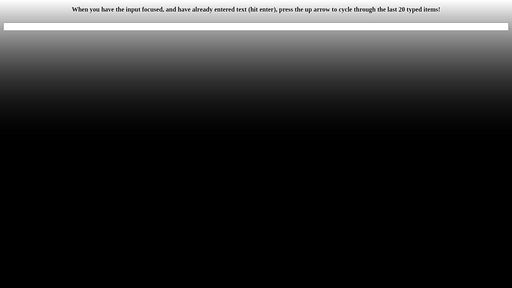
Developer | Event Horizon |
Username | Event_Horizon |
Uploaded | July 30, 2022 |
Rating | 3 |
Size | 3,912 Kb |
Views | 44,528 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Error Status | 2,922 Kb |
Gaming UI | 3,024 Kb |
Slider Colorpicker | 6,130 Kb |
Confetti | 3,734 Kb |
Character Sheet | 3,126 Kb |
Very Animated Loading Bar | 2,246 Kb |
List Sections | 2,721 Kb |
KSP altimeter | 3,150 Kb |
Content Panels | 2,189 Kb |
Music Player | 4,413 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Awesome | Samarthpd | 2,901 Kb |
FCC - Tribute Page | Cmwebby | 0 Kb |
Playing with transition timing | Mattgrosswork | 1,993 Kb |
Learning canvas drawing | Aurer | 2,204 Kb |
Fixed Scrolling Nav Bar | Philsinatra | 0 Kb |
Button Option Group | Honchoman | 1,859 Kb |
JQuery AJAX reddit Exercise | Btholt | 1,777 Kb |
Octopus Bar iPad App Interactions | Davidkpiano | 6,735 Kb |
Flat design iframe | Damienpm | 1,819 Kb |
A Pen by Mike Otis | Mikeotis | 3,185 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!