Tic Tack Toe
How do I make an tic tack toe?
What is a tic tack toe? How do you make a tic tack toe? This script and codes were developed by Donald on 10 September 2022, Saturday.
Tic Tack Toe - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Tic Tack Toe</title> <link rel='stylesheet prefetch' href='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <html>
<body> <div class='container-fluid'> <h2>Let's Play Tic Tac Toe !</h2> <h4>You vs. me, - Easy Level</h4> <div id='symbolSelect'><h3>Select your marker</h3><button onclick ='setSymbol("X")' class='symbolBtn' id='xbtn'>X</button> <button onclick ='setSymbol("O")' class='symbolBtn' id='obtn'>O</button> </div> <button onclick ='reset()' class='btn btn-block' id='reset'>Reset</button> <div id='turnDisplay'></div> <div id='gameBoard'> <table> <tbody id = 'table'></tbody> </table> </div> </div>
</body>
</html> <script src="js/index.js"></script>
</body>
</html>
Tic Tack Toe - Script Codes CSS Codes
body { text-align: center; background-color: aquamarine;
}
h2 { color: red; margin-top: 30px; font-family: comic sans ms;
}
h4 { font-size: 20px; color: blue;
}
#playerSelect { margin-top: 30px;
}
.playerBtn { border-radius: 50%; height: 50px; width: 140px; margin-bottom: 40px; margin-left: auto; margin-right: auto; font-size: 20px; background-color: blue; color: white;
}
h3 { color: purple; font-family: comic sans ms;
}
.symbolBtn { font-size: 30px; margin: 30px; width: 50px; background-color: white;
}
#reset { width: 100px; background-color: red; margin-left: auto; margin-right: auto; margin-bottom: 20px;
}
#turnDisplay { font-size: 30px; color: blue; font-weight: bold; margin-bottom: 20px;
}
#gameBoard { border-style: solid; height: 305px; width: 305px; margin: auto; margin-bottom: 20px;
}
.squareBtn { height: 100px; width: 100px; border-width: 3px;
}
#table { font-size: 45px; color: blue; font-weight: bold;
}
Tic Tack Toe - Script Codes JS Codes
var symbol = '';
var opponentSymbol = ''
var table = document.getElementById('table');
var yourTurn = true;
var boxAvailable = []; // boolean availability of each box
var remainingBoxes = 9; // available boxes remainint
var xArr = [];
var oArr = [];
var winLine = [];
var disp = document.getElementById('turnDisplay');
document.getElementByClassName('squareBtn').addEventListener('click', displaySymbol);
function reset() { for (var r = 1; r < 10; r++) { xArr[r] = 0; oArr[r] = 0; boxAvailable[r] = true; } yourTurn = true; remainingBoxes = 9 disp.innerHTML = ''; winLine = []; drawBoard();
}
function changeTurn() { yourTurn = !yourTurn; var turn = ''; var color = ''; if (yourTurn) { turn = 'Your Turn'; color = 'blue'; } else { turn = "My Turn ..."; color = 'red'; } disp.innerHTML = turn; disp.style.color = color;
}
function setSymbol(s) { reset(); symbol = s; opponentSymbol = s == 'X' ? 'O' : 'X'; var xbtn = document.getElementById('xbtn'); var obtn = document.getElementById('obtn'); if (s == 'X') { xbtn.style.background = 'yellow'; obtn.style.background = 'white'; } else { xbtn.style.background = 'white'; obtn.style.background = 'yellow'; } drawBoard();
}
function drawBoard() { var index = 1; var html = ''; for (var i = 0; i < 3; i++) { html += '<tr> '; for (var j = 0; j < 3; j++) { html += "<td> <button onclick='displaySymbol(" + index + ")' class='squareBtn' id='square" + index + "'> </button> </td> "; boxAvailable[index] = true; index++; } html += '</tr>'; } table.innerHTML = html; disp.innerHTML = 'Your Turn'; disp.style.color = 'blue';
}
function updateXOArr(n, sym) { if (sym == 'X') { xArr[n] = 1; } else { oArr[n] = 1; }
}
function displaySymbol(n) { if (yourTurn && boxAvailable[n]) { document.getElementById('square' + n).innerHTML = symbol; boxAvailable[n] = false; updateXOArr(n, symbol); remainingBoxes--; if(winCondition(symbol)) { disp.innerHTML = 'Yay, you win !'; disp.style.color = 'blue'; drawWinLine(); setTimeout(reset, 3000); return; } if (remainingBoxes === 0) { disp.innerHTML = "It's a tie ..."; disp.style.color = 'green'; setTimeout(reset, 3000); return; } changeTurn(); setTimeout(computerTurn, 1500); }
}
function randomize() { //returns a random number between 1 and 9; var num = Math.floor(Math.random() * 9) + 1; return num;
}
function computerTurn() { if (remainingBoxes > 0) { var num = 0; while (!boxAvailable[num]) { num = randomize(); } var elem = document.getElementById('square' + num); elem.innerHTML = opponentSymbol; elem.style.color = 'red'; boxAvailable[num] = false; updateXOArr(num, opponentSymbol); remainingBoxes--; if(winCondition(opponentSymbol)) { disp.innerHTML = 'I win ...'; disp.style.color = 'red'; drawWinLine(); setTimeout(reset, 3000); return; } changeTurn(); }
}
function winCondition(sym) { if (remainingBoxes > 4) return false; var checkArr = sym == 'X' ? xArr : oArr; for (var m = 1; m < 4; m++) { if(checkArr[3*(m-1)+1] + checkArr[3*(m-1)+2] + checkArr[3*(m-1)+3] == 3) { winLine = [3*(m-1)+1, 3*(m-1)+2, 3*(m-1)+3]; return true; // check for horizontal lines } else if(checkArr[3*0+m] + checkArr[3*1+m] + checkArr[3*2+m] == 3) { // check for vertial lines winLine = [3*0+m, 3*1+m, 3*2+m]; return true; } } if(checkArr[1] + checkArr[5] + checkArr[9] == 3) { winLine = [1, 5, 9]; return true; } else if (checkArr[3] + checkArr[5] + checkArr[7] == 3) { winLine = [3, 5, 7]; return true; // check for diagonal lines } else return false;
}
function drawWinLine() { for (var w = 0; w < 3; w++) { document.getElementById('square' + winLine[w]).style.background = 'yellow'; }
}
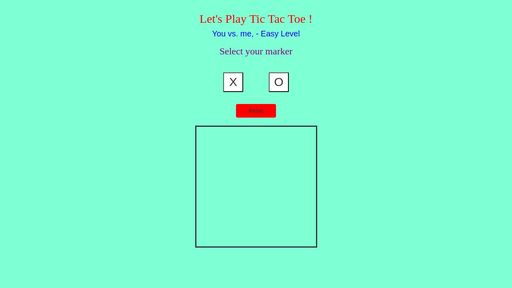
Developer | Donald |
Username | donaldk7 |
Uploaded | September 10, 2022 |
Rating | 3 |
Size | 3,361 Kb |
Views | 26,312 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Simon game | 3,768 Kb |
Twitch TV Streamer | 3,006 Kb |
Personal Portfolio | 3,826 Kb |
Pomodoro clock | 2,859 Kb |
Wikipedia Viewer | 2,469 Kb |
Tribute page | 2,142 Kb |
Quote of the Day | 2,224 Kb |
Timestamp | 1,757 Kb |
Show the Local Weather | 3,163 Kb |
Code playground | 2,078 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Highbrow Basic HTML Lesson 8 | Kimlarocca | 2,094 Kb |
GLSL Hills | Ykob | 6,991 Kb |
Pericles mi loro... | Judag | 4,125 Kb |
A Pen by Jess | Jessamyne | 5,100 Kb |
A Pen by Brendan Skousen | Bskousen | 2,954 Kb |
Spin | Elalemanyo | 8,262 Kb |
Mandelbrot Fractal | _Billy_Brown | 2,706 Kb |
Awesome textarea | Ayoungh | 1,977 Kb |
Cool Page Split Effect | Anthonyadamski | 6,128 Kb |
Loader | MikitaLisavets | 3,321 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!