Turret of Order
How do I make an turret of order?
Keeping the funny squares away who are trying to make it join the fun. Here's a secret: the turret is actually funny inside, but doesn't want to admit it. What is a turret of order? How do you make a turret of order? This script and codes were developed by Matei Copot on 12 June 2022, Sunday.
Turret of Order - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Turret of Order</title> <style> /* NOTE: The styles were added inline because Prefixfree needs access to your styles and they must be inlined if they are on local disk! */ body { overflow: hidden; background-color: black;
}
canvas { position: absolute; top: calc( 50% - 300px ); left: calc( 50% - 300px );
}
#overlay { width: 600px; height: 600px; position: absolute; top: calc( 50% - 300px ); left: calc( 50% - 300px ); background: radial-gradient( 300px at 300px 300px, rgba(0,0,0,0) 0%, rgba(0,0,0,1) 100% );
} </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/prefixfree/1.0.7/prefixfree.min.js"></script>
</head>
<body> <canvas id=c></canvas>
<div id=overlay></div> <script src="js/index.js"></script>
</body>
</html>
Turret of Order - Script Codes CSS Codes
body { overflow: hidden; background-color: black;
}
canvas { position: absolute; top: calc( 50% - 300px ); left: calc( 50% - 300px );
}
#overlay { width: 600px; height: 600px; position: absolute; top: calc( 50% - 300px ); left: calc( 50% - 300px ); background: radial-gradient( 300px at 300px 300px, rgba(0,0,0,0) 0%, rgba(0,0,0,1) 100% );
}
Turret of Order - Script Codes JS Codes
var w = c.width = 600, h = c.height = 600, ctx = c.getContext( '2d' ), opts = { shotBaseDelay: 10, shotAddedDelay: 8, radius: 400, bulletVel: 1, bulletAcc: .1, targetSpeed: 2, targetSpawnDelay: 18, turretBarrelLength: 15, turretBarrelWidth: 5, turretRadius: 10, targetBaseSquareSize: 10, targetAddedSquareSize: 3, targetBaseShine: 50, targetAddedShine: 5, baseTargetRotSpeed: .08, addedTargetRotSpeed: .01, baseTargetResizeSpeed: .08, addedTargetResizeSpeed: .01, baseTargetShineSpeed: .08, addedTargetShineSpeed: .01, targetDespawnDelay: 40, targetDespawnCircleWidth: 3, targetParticles: 3, targetParticleDistance: 10, targetParticleSize: 5, turretParticleRadius: 4, turretInnerEmptyRadius: 6, backgroundColor: 'rgba(20,20,20,.1)', turretBaseColor: 'rgba(230,230,230,1)', turretBarrelColor: '#fff', turretParticleTemplateColor: 'hsla(hue,100%,40%,1)', bulletColor: '#eee', templateTargetSquareColor: 'hsl(hue,80%,light%)', templateTargetSquareAlpColor: 'hsla(hue,80%,light%,alp)' }, tau = Math.PI * 2, tick = 0, turret, bullets = [], targets = [];
function Turret(){ this.dir = this.dirTarget = this.dirDelta = this.tick = 0; this.delay = 0; this.target; this.genTarget(); this.pickTarget();
}
Turret.prototype.genTarget = function(){ targets.push( new Target( Math.random() * tau ) );
}
Turret.prototype.shoot = function(){ if( !this.rest ) bullets.push( new Bullet( this.target ) ); this.pickTarget();
}
Turret.prototype.pickTarget = function(){ this.delay = opts.shotBaseDelay + opts.shotAddedDelay * Math.random() |0; this.tick = 0; var i = 0; while( i < targets.length && ( targets[ i ].len > 200 || targets[ i ].targeted ) ) ++i; this.rest = i >= targets.length; if( this.rest ) return -1; this.target = targets[ i ]; this.target.targeted = true; this.dir = this.dirTarget; this.dirTarget = this.target.ang; this.dirDelta = this.dirTarget - this.dir; if( this.dirDelta > Math.PI ) this.dirDelta -= tau; if( this.dirDelta < -Math.PI ) this.dirDelta += tau;
}
Turret.prototype.update = function(){ ++this.tick; if( this.tick > this.delay ) this.shoot();
}
Turret.prototype.render = function(){ var linear = this.rest ? 1 : this.tick / this.delay, armonic = -Math.cos( linear * Math.PI ) / 2 + .5, angle = this.dir + this.dirDelta * armonic; ctx.fillStyle = opts.turretBarrelColor; ctx.rotate( angle ); ctx.fillRect( opts.turretInnerEmptyRadius, -opts.turretBarrelWidth / 2, opts.turretBarrelLength - opts.turretInnerEmptyRadius, opts.turretBarrelWidth ); ctx.rotate( -angle ); ctx.fillStyle = opts.turretBaseColor; ctx.beginPath(); ctx.arc( 0, 0, opts.turretRadius, 0, tau ); ctx.arc( 0, 0, opts.turretInnerEmptyRadius, 0, tau, true ); ctx.fill(); ctx.fillStyle = opts.turretParticleTemplateColor.replace( 'hue', tick ); ctx.beginPath(); ctx.arc( 0, 0, opts.turretParticleRadius, 0, tau ); ctx.fill();
}
function Bullet( target ){ var cos = Math.cos( target.ang ), sin = Math.sin( target.ang ); this.target = target; this.len = opts.turretBarrelLength; this.px = cos * this.len; this.py = sin * this.len; this.vel = opts.bulletVel; this.vx = cos * opts.bulletVel; this.vy = sin * opts.bulletVel; // this.acc = opts.bulletAcc; this.ax = cos * opts.bulletAcc; this.ay = sin * opts.bulletAcc;
}
Bullet.prototype.update = function(){ this.len += this.vel += opts.bulletAcc; this.px += this.vx += this.ax; this.py += this.vy += this.ay; if( this.len >= this.target.len ){ this.target.getHit(); this.dead = true; }
}
Bullet.prototype.render = function(){ ctx.lineWidth = opts.turretBarrelWidth / ( this.vel / opts.bulletVel ); ctx.beginPath(); ctx.moveTo( this.px, this.py ); ctx.lineTo( this.px - this.vx * 2, this.py - this.vy * 2 ); ctx.stroke();
}
function Target( ang ){ var cos = Math.cos( ang ), sin = Math.sin( ang ); this.ang = ang; this.cos = cos; this.sin = sin; this.len = opts.radius; this.px = cos * opts.radius; this.py = sin * opts.radius; // this.vel = opts.targetSpeed; this.vx = cos * -opts.targetSpeed; this.vy = sin * -opts.targetSpeed; this.tick = 0; this.color = opts.templateTargetSquareColor.replace( 'hue', ang / tau * 360 ); this.alpColor = opts.templateTargetSquareAlpColor.replace( 'hue', ang / tau * 360 ); this.rotateSpeed = opts.baseTargetRotSpeed + opts.addedTargetRotSpeed * Math.random(); this.resizeSpeed = opts.baseTargetResizeSpeed + opts.addedTargetResizeSpeed * Math.random(); this.shineSpeed = opts.baseTargetShineSpeed + opts.addedTargetShineSpeed * Math.random();
}
Target.prototype.update = function(){ if( this.hit ) return this.updateHit(); this.len -= opts.targetSpeed; this.px += this.vx; this.py += this.vy; ++this.tick;
}
Target.prototype.getHit = function(){ this.hit = true; this.tick = 0;
}
Target.prototype.updateHit = function(){ ++this.tick; if( this.tick > opts.targetDespawnDelay ) this.dead = true;
}
Target.prototype.render = function(){ if( this.hit ) return this.renderHit(); var ang = this.tick * this.rotateSpeed, size = opts.targetBaseSquareSize + opts.targetAddedSquareSize * Math.cos( this.resizeSpeed * this.tick ); ctx.fillStyle = this.color.replace( 'light', opts.targetBaseShine + opts.targetAddedShine * Math.cos( this.shineSpeed * this.tick ) );; ctx.translate( this.px, this.py ); ctx.rotate( ang ); ctx.fillRect( -size / 2, -size / 2, size, size ); ctx.rotate( -ang ); ctx.translate( -this.px, -this.py ); for( var i = 0; i < opts.targetParticles; ++i ){ var len = Math.random() * opts.targetParticleDistance, ang = Math.random() * tau, size = Math.random() * opts.targetParticleSize; ctx.fillRect( this.px + len * Math.cos( ang ) - size / 2, this.py + len * Math.sin( ang ) - size / 2, size, size ); }
}
Target.prototype.renderHit = function(){ var linear = this.tick / opts.targetDespawnDelay, armonic = -Math.cos( linear * Math.PI ) / 2 + .5; ctx.fillStyle = this.alpColor.replace( 'light', 25 + 75 * ( 1 - linear ) ).replace( 'alp', .3 * ( 1 - linear ) ); var spacing = opts.targetDespawnCircleWidth * armonic; ctx.beginPath(); ctx.arc( 0, 0, this.len, 0, tau ); ctx.arc( -this.cos * spacing, -this.sin * spacing, this.len - spacing, 0, tau, true ); ctx.fill();
}
function anim(){ window.requestAnimationFrame( anim ); ++tick; ctx.fillStyle = opts.backgroundColor; ctx.fillRect( 0, 0, w, h ); if( tick % opts.targetSpawnDelay === 0 ) turret.genTarget(); ctx.translate( w / 2, h / 2 ); turret.update(); targets.map( function( target ){ target.update(); } ); bullets.map( function( bullet ){ bullet.update(); } ); for( var i = 0; i < bullets.length; ++i ) if( bullets[ i ].dead ){ bullets.splice( i, 1 ); --i; } for( var i = 0; i < targets.length; ++i ) if( targets[ i ].dead ){ targets.splice( i, 1 ); --i; } ctx.fillStyle = 'rgb(30,30,30)'; ctx.beginPath(); ctx.arc( 0, 0, opts.turretBarrelLength, 0, tau ); ctx.arc( 0, 0, opts.turretRadius - 1, 0, tau, true ); ctx.fill(); turret.render(); ctx.globalCompositeOperation = 'lighter'; ctx.strokeStyle = opts.bulletColor; targets.map( function( target ){ target.render(); } ); bullets.map( function( bullet ){ bullet.render(); } ); ctx.globalCompositeOperation = 'source-over'; ctx.translate( -w / 2, -h / 2 );
}
turret = new Turret();
ctx.fillStyle = '#222';
ctx.fillRect( 0, 0, w, h );
anim();
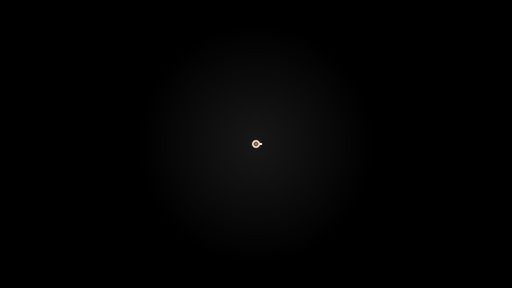
Developer | Matei Copot |
Username | towc |
Uploaded | June 12, 2022 |
Rating | 4.5 |
Size | 4,000 Kb |
Views | 54,648 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Organic lines of staring-atness | 2,415 Kb |
Mathematically armonic circular lines | 2,476 Kb |
Neural Network visualization | 4,562 Kb |
Experiment with basic 3d on 2d canvas | 2,577 Kb |
Rotating tunnel of rainbowness | 2,885 Kb |
Superimpositional garden | 3,307 Kb |
Wavy 3d rainbow lattice | 2,951 Kb |
Invasion of creepy crawly rainbow fireflies | 3,094 Kb |
Swirly electric rainbow lines | 2,505 Kb |
Springing Particles | 2,493 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
SlideDown FixedMenu | Mp_graphic | 5,602 Kb |
A Pen by Alex Edwards | Exards | 8,218 Kb |
JavaScript constructors | Simboonlong | 2,415 Kb |
Price table | Serluk | 5,928 Kb |
CSS3 keyframe onload animation. | Samueljseay | 1,706 Kb |
Blog | Rottingroom | 1,430 Kb |
Responsive Section hover effect to show content | Berdejitendra | 2,540 Kb |
CSS Variables | Jdsteinbach | 4,759 Kb |
Social buttons | Flacu | 2,022 Kb |
Bootstrap 4 Gridsystem Demo | Rivella50 | 1,535 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!