Twitch API - FreeCodeCamp
How do I make an twitch api - freecodecamp?
Loads the first 16 users on a given user's followed list using the Twitch TV API. What is a twitch api - freecodecamp? How do you make a twitch api - freecodecamp? This script and codes were developed by Trevor Welch on 01 February 2023, Wednesday.
Twitch API - FreeCodeCamp - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Twitch API - FreeCodeCamp</title> <link href="https://fonts.googleapis.com/css?family=Open+Sans|Orbitron" rel="stylesheet"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <form onsubmit="event.preventDefault(); getFollowedByUser();">
<div id="loading" class="loading hidden"> <p class="loadingtext">Fetching User Data</p>
</div>
<div class="main"> <div class="container"> <div class="border"> <div class="application"> <div class="title-bar"> <img src="http://static1.squarespace.com/static/510d16d5e4b0e3b888b8ec28/t/546dae7ee4b01ffc563db48d/1416474238538.png" class="tLogo"> <h1 class="title">Streamer Status </h1> </div> <div class="search-bar"> <p>Show status of streamers that user follows</p> <input type="text" id="search" class="search" autocomplete="off"> </div> <div class="options"> <input type="radio" name="status" value="0" id="sAll" checked="checked"><label for="sAll">All</label><input type="radio" name="status" value="1" id="sOnline"><label for="sOnline">Online</label><input type="radio" name="status" value="2" id="sOffline"><label for="sOffline">Offline</label> </div> <div id="streamers" class="streamer-well"> </div> </div> </div> </div>
</div>
</form> <script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Twitch API - FreeCodeCamp - Script Codes CSS Codes
*{ margin:0; padding:0;
}
.hidden{ display:none;
}
body{ font-family: 'Open Sans', sans-serif; background:#fafafa;
}
.tLogo{ height:40px; float:left;
}
.title-bar{ padding:8px; text-align:center; display:inline-block; margin:auto; border-bottom:1px solid #6441A4;
}
.title{ display:inline; line-height:40px; font-family: 'Orbitron', sans-serif; padding-left:8px; color:#6441A4;
}
.main{ width:100vw; min-height:100vh;
}
.container{ width:100%; min-height:100vh; padding:40px 0; display:flex; align-items:center; justify-content:center;
}
.application{ background:#fff; display:flex; flex-direction:column; width:100%; border:2px solid #6441A4; margin-left:-2px; margin-top:-2px;
}
.border{ padding:4px 4px 2px 4px; border:4px solid #6441A4;
}
.options{ margin:auto; padding-bottom:8px;
}
input[type=radio]{ display:none;
}
label{ padding:4px 8px; width:50px; text-align:center; display:inline-block; transition:0.2s; color:#fff; background:#6441A4; opacity:0.3;
}
input[type=radio]:checked+label{ opacity:1;
}
.search-bar,.nouser{ padding-top:8px; margin:auto; text-align:center;
}
.search-bar p{ font-size:12px; color:#6441A4;
}
.search{ display:inline-block; width:200px; padding:4px 16px; border-radius:20px; border:2px solid #6441A4; font-size:18px;
}
.search:focus{ outline:0;
}
.streamer-well{ min-height:400px; padding-bottom:8px;
}
.user{ display:flex; align-items:center; justify-content:space-between; padding:8px; margin:0px 8px; border-left:2px solid #fff; border-right:2px solid #fff; max-width:600px; cursor:pointer;
}
.user:hover{ border-left:2px solid #B19DD8; border-right:2px solid #B19DD8; color:#6441A4;
}
.information{ display:flex; align-items:center;
}
.av{ height:50px; width:50px; border-radius:50px;
}
.details{ padding-left:8px;
}
.displayname{ line-height:22px; font-size:20px;
}
.streaming{ line-height:17px; font-size:14px; opacity:0.34;
}
.status{ padding-right:8px;
}
.online{ background:#14A71D; height:20px; width:20px; border-radius:30px;
}
.greyBall{ height:5px; width:5px; border-radius:10px; background:#EEEEEE; display:inline-block; margin-bottom:4px; margin-left:4px;
}
a{ text-decoration:none; color:inherit;
}
.loading{ width:100vw; height:100vh; display:flex; align-items:center; justify-content:center; position:fixed; z-index:999; background:rgba(0,0,0,0.5);
}
.loadingtext{ font-size:72px; line-height:80px; opacity:1; color:#fff; font-family: 'Orbitron', sans-serif;
}
Twitch API - FreeCodeCamp - Script Codes JS Codes
/*
Okay, there is a lot to this page.
The goal is to display a list of users and if they are streaming or not based on a given array.
Secondly, the user must be able to filter this result based on online or offline.
I've decided to add the ability for a user to search for the accountss another user followers.
A user may also search for specifically one user as well.
*/
//var vDom is a global variable that is never intialized, but for some reason it can be accessed and saved to.
//I use vDom to hold a JSON of the users after it is loaded from the API but before it is turned into HTML. //Initialize the page using sky_mp3's followers, but now disabled due to pen page taking forever to load.
function isOnline(user){ return user.online == true;
}
function isOffline(user){ return user.online == false;
}
function filterUsers(){ //This function is called when the radio buttons are clicked. By using radio buttons, a user can not click the same tab over and over and waste processing power var res = vDom; var howToFilter = $("input[name='status']:checked").val(); var filtered = []; switch(howToFilter){ case "1": filtered = res.filter(isOnline); break; case "2": filtered = res.filter(isOffline); break; default: filtered = res; break; } drawFromJSON(filtered);
}
function getFollowers(query){ //Does a query based on the username entered $("#loading").fadeIn(300); var res = []; $.ajax({ url:"https://api.twitch.tv/kraken/channels/"+query+"/follows/?limit=16&client_id=wq2a1r04kq5fp9trbtij0k71w78l90" }).done(function(data){ data["follows"].forEach(function(e){ res.push(e.user.name); }); searchByList(res); });
}
/* DEPRECATED FUNCTION
function searchByUser(){ //Loads a single status result var user = $("#search").val(); if(user!=""&&user.length>3){ var userJSON=[]; userJSON[0] = getUserData(user); if(userJSON[0].username){ drawFromJSON(userJSON,true); }else{ $("#streamers").html("<div class='nouser'>No streamer found.</div>"); } }
} */
function getFollowedByUser(){ var user = $("#search").val(); if(user!=""&&user.length>3){ var userJSON=[]; userJSON[0] = getUserData(user); if(userJSON[0].username){ getFollowers(user); }else{ $("#streamers").html("<div class='nouser'>No user found.</div>"); } }
}
function searchByList(list){ //Loads the status result of a list of users var userJSON=[]; list.forEach(function(user){ userJSON.push(getUserData(user)); }); drawFromJSON(userJSON, true);
}
function getUserData(query){ //Gets the user object for the username given var res = {}; $.ajax({ url:"https://api.twitch.tv/kraken/channels/"+query+"?client_id=wq2a1r04kq5fp9trbtij0k71w78l90", async:false }).done(function(data){ res.username = data.display_name; res.game = data.status; res.id = data._id; res.url = data.url; if(data.logo!=null){ res.av = data.logo; }else{ res.av = "http://placehold.it/50x50"; } res.online = checkOnline(query); }); return res;
}
function checkOnline(user){ //Checks if user is online var res = false; $.ajax({ url:"https://api.twitch.tv/kraken/streams/"+user+"?client_id=wq2a1r04kq5fp9trbtij0k71w78l90", async: false }).done(function(data){ if(data.stream != null){ res = true; } }); return res;
}
function drawFromJSON(userlist, overwrite){ //Draws the page, if overwrite is set to true, it changes the virtual page so search results will be set based on the JSON given if(overwrite==true){ vDom = userlist.slice(0); } var dDom = []; userlist.forEach(function(user){ dDom += addUser(user); }); $("#streamers").html(dDom); $("#loading").fadeOut(300);
}
function addUser(user){ //This just creates a user tile from the JSON given. var res = ""; res += "<a href='"+user.url+"' target='_blank'><div class='user'><div class=information><img src='"+user.av+"' class='av'><div class='details'><p class='displayname'>"+user.username+"</p>"; if(user.online===true){ res+= "<p class='streaming'>"+user.game+"</p>"; } res += "</div></div><div class='status'>"; if(user.online===true){ res+="<div class='online'></div>"; }else{ res+="<div class='offline'><div class='greyBall'></div><div class='greyBall'></div><div class='greyBall'></div></div>"; } res+="</div></div></div></a>"; return res;
}
$("input[name='status']").change(filterUsers);
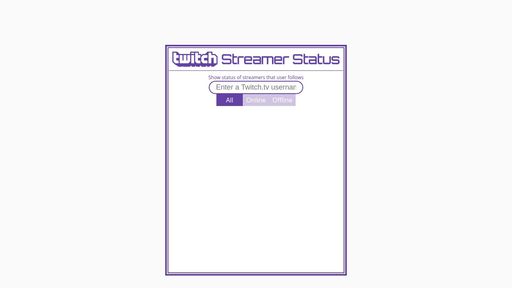
Developer | Trevor Welch |
Username | TrevorWelch |
Uploaded | February 01, 2023 |
Rating | 3 |
Size | 4,568 Kb |
Views | 2,024 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
React - Click the Cookie | 2,538 Kb |
Flat UI Calculator - FreeCodeCamp | 2,961 Kb |
Pomodoro Clock - FreeCodeCamp | 3,555 Kb |
FreeCodeCamp - Tribute Page | 3,005 Kb |
Button | 2,302 Kb |
Wikipedia API - FreeCodeCamp | 3,572 Kb |
FreeCodeCamp - Portfolio | 2,948 Kb |
TicTacToe - FreeCodeCamp | 5,031 Kb |
Random Quote Generator - FreeCodeCamp | 2,944 Kb |
StopButton MockUp | 6,562 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
CSS Social Media Icon | TychoBlender | 3,871 Kb |
Sticky div | Kaslab | 2,225 Kb |
RRC wrapSwitch | Pshrmn | 2,922 Kb |
Text Looping Transition | Agelber | 5,619 Kb |
Angular-HAML | Cwacht | 2,022 Kb |
Fixed Scrolling Nav Bar | Philsinatra | 0 Kb |
Hello People | Danburrows | 2,365 Kb |
Transitioning application screens with semantically named classes | Djgrant | 3,697 Kb |
Comment Jquery | SquishyAndroid | 2,421 Kb |
SVG Scalable Text | Said_FD | 1,451 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!