Weather App
How do I make an weather app?
What is a weather app? How do you make a weather app? This script and codes were developed by Tushar Singh on 08 July 2022, Friday.
Weather App - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Weather App</title> <link rel='stylesheet prefetch' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <link href='https://fonts.googleapis.com/css?family=Lato:400,700' rel='stylesheet' type='text/css'>
<div class="container" id="weather-page"> <h1 id="location">Your location</h1> <div class="row"> <div class="col-md-6 left-col"> <span id="current-temp" class="text-left">37 ° </span><br> <button class="btn btn-default" id="c">° Celsius</button> <button class="btn btn-default" id="f">° Fahrenheit</button> </div> <div class="col-md-6 right-col"> <img src="http://icons.iconarchive.com/icons/oxygen-icons.org/oxygen/256/Status-weather-showers-day-icon.png" width="100px" id="icon"> <span id="description">Weather</span><br> <div class="hum"> <img src="http://icons.iconarchive.com/icons/custom-icon-design/lovely-weather-2/512/Humidity-icon.png" height="50x" class="drop"> <span id="humidity">0 </span><br> </div> <img src="http://www.seos-project.eu/modules/oceancurrents/images/currents_ch2_wind_cartoon.png" width="60px"> <span id="wind">0 </span> mph <span id="direction"> N </span> </div> </div>
</div> <script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.2/jquery.min.js'></script>
<script src='http://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Weather App - Script Codes CSS Codes
/*dosen't look good on smaller screens, working on it*/
body { background-image: url('http://freetopwallpaper.com/wp-content/uploads/2013/10/beautiful-mist-wallpaper-hd-66.jpg'); color: white; font-family: 'Lato', sans-serif;
}
#weather-page { width: 50%; background-color: black; margin-top: 5%; border-radius: 10px; -webkit-box-shadow: 0 2px 6px rgba(0, 0, 0, 0.5), inset 0 1px rgba(255, 255, 255, 0.3), inset 0 10px rgba(255, 255, 255, 0.2), inset 0 10px 20px rgba(255, 255, 255, 0.25), inset 0 -15px 30px rgba(0, 0, 0, 0.3); -moz-box-shadow: 0 2px 6px rgba(0, 0, 0, 0.5), inset 0 1px rgba(255, 255, 255, 0.3), inset 0 10px rgba(255, 255, 255, 0.2), inset 0 10px 20px rgba(255, 255, 255, 0.25), inset 0 -15px 30px rgba(0, 0, 0, 0.3); box-shadow: 0 2px 6px rgba(0, 0, 0, 0.5), inset 0 1px rgba(255, 255, 255, 0.3), inset 0 10px rgba(255, 255, 255, 0.2), inset 0 10px 20px rgba(255, 255, 255, 0.25), inset 0 -15px 30px rgba(0, 0, 0, 0.3); background: rgba(0, 0, 0, 0.25); padding-bottom:30px;
}
h1 { border-bottom: 1px solid white; padding-bottom: 20px; text-align: center;
}
#current-temp { font-size: 8em; margin-left: 25%; padding-right: 30%; width: 40%;
}
.left-col { border-right: 1px solid white;
}
.right-col { padding-left: 10%;
}
.btn { margin-left: 10%; width: 100px; background: none; border: 2px solid white; border-radius: 5%; color: white
}
.btn:hover { background-color: #333; outline: none; color: white;
}
.btn:focus, .btn:active { outline:none;
}
#humidity,
#wind,
#direction { font-size: 1.5em;
}
.hum { padding-bottom: 10px;
}
@media (max-width: 580px) { #weather-page { width: 90%; font-size: 0.5em; }
}
Weather App - Script Codes JS Codes
//1. Use geolocation
//2. ajax call
//3. gather data and append it to the body
//4. convert C to F
//5. Background images accoring to the current weather
//6. wind- degrees into NEWS
// assigining directions-NEWS to wind direction
function degToDir(degree) { if (degree > 315 || degree < 45) { return "N"; } else if (degree >= 45 & degree < 135) { return "E"; } else if (degree >= 135 & degree < 225) { return "S"; } else { return "W" }
}
$(document).ready(function() { var lat, lon, api; var tempC; // geolocation if ("geolocation" in navigator) { navigator.geolocation.getCurrentPosition(gotLocation); function gotLocation(position) { lat = position.coords.latitude; lon = position.coords.longitude; api = 'http://api.openweathermap.org/data/2.5/weather?lat=' + lat + '&lon=' + lon + '&units=metric&appid=0c11494b22283368ff17b460898a6c8c'; // AJAX call $.ajax({ url: api, method: 'GET', success: function(data) { var location = data.name; tempC = Math.round(data.main.temp); var humidity = data.main.humidity; var wind = data.wind.speed; var direction = degToDir(data.wind.deg); var country = data.sys.country var description = data.weather[0].description; var iconURL = 'http://openweathermap.org/img/w/' + data.weather[0].icon + '.png'; var id = data.weather[0].id; // Use the data from api $('#location').text(location + ', ' + country); $('#current-temp').text(tempC + '°'); $('#humidity').text(humidity + " %"); $('#wind').text(wind); $('#direction').text(direction); $('#description').text(description.toUpperCase()); $("#icon").attr('src', iconURL); // converting metric to imperial var tempF = Math.round(tempC * 1.8 + 32); // button function function changeUnit() { $("#c").click(function() { $("#current-temp").empty().html(tempC + "°"); }); $("#f").click(function() { $("#current-temp").empty().html(tempF + "°"); }); } // change background with respect to the id function changeBackground() { if (id >= 200 & id < 600) { $('body').css("background", "url('http://wallpapercave.com/wp/OSPaSJC.jpg')"); } else if (id >= 600 & id < 700) { $('body').css("background", "url('http://cdn1.theodysseyonline.com/files/2015/12/20/635861833670816810507191518_6670-perfect-snow-1920x1080-nature-wallpaper.jpg')"); } else if (id > 700 & id < 800) { $('body').css("background", "url('http://freetopwallpaper.com/wp-content/uploads/2013/10/beautiful-mist-wallpaper-hd-66.jpg')"); } else if (id >= 800 & id < 804) { $('body').css("background", "url('http://i.jootix.com/o/unnamed--0a8c5fdd74.jpg')"); } else if (id === 804) { $('body').css("background", "url('https://upload.wikimedia.org/wikipedia/commons/a/a3/Overcast_skies_from_Tropical_Storm_Danny.jpg')"); } } // call functions changeUnit(); changeBackground(); } }); // end ajax } // end gotLocation } // end if statement else { alert("Your browser dosen't support Geolocation, Sorry."); }
}); // end document.ready
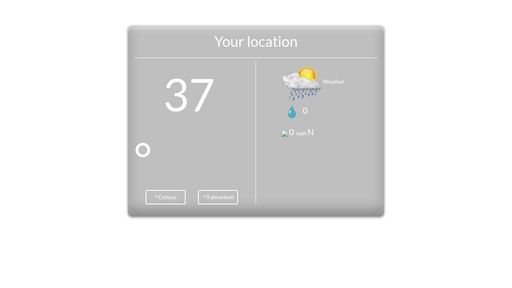
Developer | Tushar Singh |
Username | tushar_13 |
Uploaded | July 08, 2022 |
Rating | 3 |
Size | 3,952 Kb |
Views | 52,624 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Wikipedia Viewer | 2,993 Kb |
Tic Tac Toe | 3,335 Kb |
Hamburger for smaller screens | 3,991 Kb |
Portfolio | 5,673 Kb |
Twitch.tv | 4,305 Kb |
Tribute Page | 2,864 Kb |
Random Quote Generator | 4,395 Kb |
Experiments | 2,004 Kb |
Pomodoro Clock | 4,777 Kb |
Random Stuff | 1,762 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Preloader | Rikki_Sixx | 2,815 Kb |
Bootstrap Carousel Fade Transition | Rowno | 2,484 Kb |
Custom checkbox example | Capelo | 3,495 Kb |
CMP5-Opdracht15 | SannevanGastel | 2,733 Kb |
Beautiful canvas stars | Matths | 2,399 Kb |
Fullscreen Parallax | Bassta | 3,313 Kb |
Playing with FlexBox | _Billy_Brown | 3,162 Kb |
Hover Animation from UNIQLO | Insprd | 3,772 Kb |
Product item | Mymahesh11 | 2,256 Kb |
Revolving Text Landing Page Trial | TimRuby | 2,976 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!