Calender with ReactJS
How do I make an calender with reactjs?
What is a calender with reactjs? How do you make a calender with reactjs? This script and codes were developed by Ajala Comfort on 11 January 2023, Wednesday.
Calender with ReactJS - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Calender with ReactJS</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.6.3/css/font-awesome.css" rel="stylesheet">
<link href="https://fonts.googleapis.com/css?family=Roboto:300,400,700" rel="stylesheet"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <body class="nopaddingnomargin"> <div id="space"></div>
</body> <script src='https://code.jquery.com/jquery-2.2.4.min.js'></script>
<script src='https://fb.me/react-15.1.0.min.js'></script>
<script src='https://fb.me/react-with-addons-15.0.1.min.js'></script>
<script src='https://npmcdn.com/[email protected]/dist/react-dom.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Calender with ReactJS - Script Codes CSS Codes
body{width:100%;height:700px;}
#space{width:400px;margin:auto;margin-top:100px;font-family: 'Roboto', sans-serif;}
.nopaddingnomargin{margin:0;padding:0}
#calender{background:#303f9f;min-height:400px;width:100%;}
#header{width:90%;margin-left:5%;height:30px;color:white;padding-top:20px;}
#header i {width:13%;}
#header p{width:60%;}
#header i, #header p{float:left;height:100%;font-size:18px;}
ul{list-style:none;}
#dates {margin-top:20px;min-height:400px;}
#dates > div{width:14%;float:left;}
#dates > div ul{min-height:300px;width:100%;}
#dates p{text-align:center;color:rgba(189, 195, 199,0.6)}
#dates > div > ul > li{margin-top:15%;text-align:center;color:white;width:50px;height:40px;cursor:pointer;border-radius:50%;padding-top:20px;}
#dates li:hover{animation-name:circle;animation-duration:4s;}
.today{color:#e91e63 !important;}
#taskList{width:100%;min-height:110px;background:rgba(236, 240, 241,1.0);}
#taskheader{color:#7f8c8d;font-size:20px;padding-top:10px;width:90%;margin-left:5%;}
#taskheader input{border:none;height:30px;width:70%;}
#taskList button{width:60px;height:30px;border:none;background:#f8bbd0;}
#taskList ul{margin:0;padding:0;margin-top:20px;color:#9e9e9e;font-size:15px;}
#taskList li{min-height:50px;width:100%;background:#eeeeee;color:black;margin-top:5px;}
#taskList > p {width:100%;padding:0;margin:0;margin-left:5%;}
.delete, .update {width:20%;text-align:center;padding-top:25px;height:25px;display:inline-block;}
#taskList p{display:inline-block;float:left;height:50px;}
#taskList li > p{width:50%;margin-left:5%;}
#taskList li > div > input {background:rgba(241, 196, 15,0.2);width:70%;height:50px;border:none;text-align:center;display:inline-block;}
#taskList li > div > button {width:29%;border:none;background:white;color:gold;height:50px;display:inline-block;}
.delete{background: #e91e63;}
.update{background:white;}
@keyframes circle{ from{background:rgba(189, 195, 199,0.2)} to{white;color:black;background:white;}
}
/**************TRANSITION **/
.slideoff-leave.slideoff-leave-active{ opacity:0.01; margin-left:-90%; transition:all 2s ease-in;
}
Calender with ReactJS - Script Codes JS Codes
'use strict';
var Trans = React.addons.CSSTransitionGroup;
//TILL NEXT TUTORIALLLLL!!!!!!
/********* LOGIC OF THE CALENDER ****
1.Create Display / Look
2. CREATE A MONTH OBJECT AT EACH DISPLAY
*******/
var daysOfWeek = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
var MonthNames = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
var abbrev = ["Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"];
function Month(month, year, dates) { //Create a contructor to create month objects this.date = new Date(year, month, 0); this.numberofdays = this.date.getDate(); this.nameofmonth = MonthNames[this.date.getMonth()]; this.firstday = 1; this.year = this.date.getFullYear(); this.calender = generateCalender(this.numberofdays, month - 1, this.firstday, year, dates);
}
function Date2Day(year, month, day) { //this is to determne the week day based on the date return new Date(year, month, day).getDay();
}
function generateCalender(numberofdays, month, day, year, dates) { //this generates an array of week dey objects which have array of days ( in number) var WEEKDAY = daysOfWeek[Date2Day(year, month, day)]; if (WEEKDAY in dates) { dates[WEEKDAY].push(day); } else { dates[WEEKDAY] = [day]; } day++; return day > numberofdays ? dates : generateCalender(numberofdays, month, day, year, dates);
}
var Calender = React.createClass({ displayName: 'Calender', getInitialState: function getInitialState() { return this.generateCalender(); }, generateCalender: function generateCalender() { var today = new Date(); var month = {}; month = new Month(today.getMonth() + 1, today.getFullYear(), month); console.log(today.getDate()); return { dates: month, today: today, taskadd: false, taskday: "", tasklist: [], activeList: [] }; }, addTask: function addTask(day) { day = day + " " + this.state.dates.nameofmonth + " " + this.state.dates.year; this.search(); return this.setState({ taskadd: true, taskday: day }); }, enterTask: function enterTask() { var new_task = {}; if ($("#task").val() && $("#task").val().length > 0) { //console.log(this.state.taskday) //create a task object and use the selected date as the key and the task as the propertiy new_task[this.state.taskday] = [$("#task").val()]; //update the tasklist by checking if the tasklist has the date already store or not //I'll use my logic -- if there is one. ... hmm var positionInList; this.state.tasklist.forEach(function (taskObj, i) { if (this.state.taskday in taskObj && positionInList === undefined) { positionInList = i; } }.bind(this)); var list = this.state.tasklist; if (positionInList != undefined) { list[positionInList][this.state.taskday].push($("#task").val()); } else { list.push(new_task); } //YEAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA!!!! //TILL NEXT TIME this.search(); return this.setState({ tasklist: list }); } else { console.log("Done nothing"); } }, search: function search() { ///SEARCH FOR DAY var result = $.grep(this.state.tasklist, function (taskObj) { return this.state.taskday in taskObj; }.bind(this)); if (result && result.length > 0) { this.setState({ activeList: result[0][this.state.taskday] }); } }, updateTask: function updateTask(i, id, e) { //update the active List and use the active List to update the tasklist based the taskday this.state.activeList[i] = $("#" + id).val(); return this.updateTaskList(this.state.activeList); }, updateTaskList: function updateTaskList(list) { var tasklist = this.state.tasklist; tasklist[this.state.taskday] = list; return this.setState({ tasklist: tasklist, activeList: list }); }, deleteTask: function deleteTask(i) { var list = this.state.activeList; list.splice(i, 1); return this.setState({ activeList: list }); }, render: function render() { //console.log(this.state.dates) var calender = []; for (var property in this.state.dates.calender) { calender.push(this.state.dates.calender[property]); } //console.log(calender) return React.createElement( 'div', { id: 'calender' }, React.createElement( 'div', { id: 'header' }, React.createElement('i', { className: 'fa fa-bars', 'aria-hidden': 'true' }), React.createElement( 'p', { className: 'nopaddingnomargin' }, this.state.dates.nameofmonth, ' ', this.state.dates.year, ' ' ), React.createElement('i', { className: 'fa fa-calendar-check-o', 'aria-hidden': 'true' }), React.createElement('i', { className: 'fa fa-search', 'aria-hidden': 'true' }) ), React.createElement( 'div', { id: 'dates' }, Object.keys(this.state.dates.calender).map(function (weekday, i) { var list = this.state.dates.calender[weekday].map(function (day, i) { return React.createElement( 'li', { className: day == this.state.today.getDate() ? "today" : null, onClick: this.addTask.bind(null, day) }, day ); }.bind(this)); return React.createElement( 'div', null, React.createElement( 'p', { className: 'weekname' }, weekday.substring(0, 3) ), React.createElement( 'ul', { className: 'nopaddingnomargin' }, list ) ); }.bind(this)) ), this.state.taskadd ? React.createElement( 'div', { id: 'taskList' }, React.createElement( 'div', { id: 'taskheader' }, React.createElement( 'p', null, 'Entry Date: ', this.state.taskday ), React.createElement('input', { type: 'text', id: 'task' }), React.createElement( 'button', { onClick: this.enterTask }, 'Add' ) ), React.createElement( 'ul', null, React.createElement( Trans, { transitionName: 'slideoff', transitionEnterTimeout: 500, transitionLeaveTimeout: 2000 }, this.state.activeList.map(function (task, i) { return React.createElement( 'li', { key: task + i }, React.createElement( 'p', null, task ), React.createElement( 'span', { className: 'delete', onClick: this.deleteTask.bind(null, i) }, 'delete' ), React.createElement( 'span', { className: 'update' }, 'update' ), React.createElement( 'div', { id: 'updateRoom' }, React.createElement('input', { type: 'text', id: task + i }), React.createElement( 'button', { onClick: this.updateTask.bind(null, i, task + i) }, 'Update' ) ) ); }.bind(this)) ) ) ) : null ); }
});
ReactDOM.render(React.createElement(Calender, null), document.getElementById("space"));
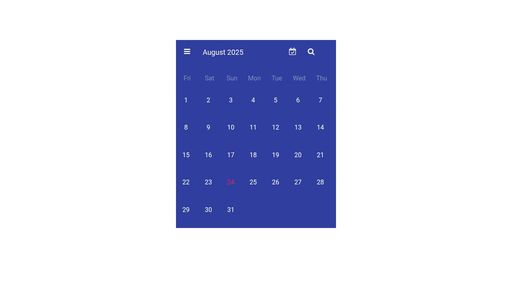
Developer | Ajala Comfort |
Username | AJALACOMFORT |
Uploaded | January 11, 2023 |
Rating | 3 |
Size | 6,983 Kb |
Views | 6,072 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Material Chat app Interface with ReactJS | 5,851 Kb |
Palindrome Word | 3,421 Kb |
Second Website | 6,234 Kb |
Recipe Book | 4,813 Kb |
Calender Update | 6,157 Kb |
Bill Splitting App | 6,825 Kb |
Calculator with ReactJs | 4,475 Kb |
Intermediate Todo List with React.js | 6,191 Kb |
Hashtables | 1,836 Kb |
COLOR PICKER | 8,468 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Blackberry Mock | Zacharyolson | 1,865 Kb |
Multiple jCarousel | Pafnuty | 2,461 Kb |
Fellowship of the Ring | Aussieyang | 2,639 Kb |
Falling Down the Rabbit Hole | Rachelnabors | 4,578 Kb |
Responsive Pricing Table | Jeremycaris | 2,690 Kb |
AngularJS Datalist Directive | M-e-conroy | 2,366 Kb |
A Pen by Moeid Saleem | Moeidsaleem | 1,862 Kb |
Project -Show the Local Weather | Luciano_Britis | 2,583 Kb |
Css3 slide | Nakome | 3,190 Kb |
Headroom.js demo | WickyNilliams | 3,982 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!