Colorized Perlin Noise Generator
How do I make an colorized perlin noise generator?
Experimenting with Perlin noise. Includes some colorization options and value easing functions.Choose presets from the dropdown or make your own.. What is a colorized perlin noise generator? How do you make a colorized perlin noise generator? This script and codes were developed by Chris Johnson on 01 December 2022, Thursday.
Colorized Perlin Noise Generator - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Colorized Perlin Noise Generator</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body>
<canvas></canvas>
<div class="presets-container"> <div class="preset" data-preset="Combustion"><img src="https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/perlin-gen-combustion.png"/></div> <div class="preset" data-preset="Terrain"><img src="https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/perlin-gen-terrain.png"/></div> <div class="preset" data-preset="Infrared"><img src="https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/perlin-gen-infrared.png"/></div> <div class="preset" data-preset="X-Ray"><img src="https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/perlin-gen-x-ray.png"/></div> <div class="preset" data-preset="Cloudy"><img src="https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/perlin-gen-clouds.png"/></div> <div class="preset" data-preset="Radiation"><img src="https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/perlin-gen-radiation.png"/></div>
</div>
<p class="message">Textures may take a moment to render/update</p>
<button class="show-presets">Select Preset</button> <script src='https://s3-us-west-2.amazonaws.com/s.cdpn.io/168886/recurve.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.6.1/dat.gui.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Colorized Perlin Noise Generator - Script Codes CSS Codes
html, body { width: 100%; height: 100%;
}
body { background: #000000; overflow: hidden;
}
canvas { -webkit-transform-origin: 0 0; transform-origin: 0 0;
}
.message { position: absolute; bottom: 0; left: 0; padding: 2px; margin: 0; color: #ffffff; font-family: sans-serif; font-size: 16px; text-shadow: 1px 1px 3px #000;
}
.fade { opacity: 0; -webkit-transition: 3s; transition: 3s; -webkit-transition-delay: 10s; transition-delay: 10s;
}
.presets-container { position: absolute; left: 0; top: 0; width: 768px; overflow: hidden; -webkit-transition: opacity 400ms; transition: opacity 400ms; -webkit-transform-origin: 0 0; transform-origin: 0 0;
}
.preset { cursor: pointer; float: left; width: 256px; height: 256px;
}
.preset img { width: 256px; height: 256px; margin: 0;
}
.hide { pointer-events: none; opacity: 0; -webkit-transition: opacity 0ms; transition: opacity 0ms;
}
button.show-presets { cursor: pointer; position: absolute; right: 20px; bottom: 10px; padding: 10px 20px; margin: 0; color: #fff; font-family: sans-serif; font-size: 20px; background: #333; border: none; border-radius: 24px;
}
button.show-presets:hover, button.show-presets:focus { background: #555;
}
Colorized Perlin Noise Generator - Script Codes JS Codes
var opts;
var presetsContainer;
var canvas;
/* * noise generation */
// simplified version of Perlin noise for JS adapted from https://github.com/josephg/noisejs
// util for dot products
function Grad(x, y) { this.x = x; this.y = y;
}
Grad.prototype.dot2 = function(x, y) { return this.x*x + this.y*y;
};
// permutations
var grads = [ new Grad(1,1),new Grad(-1,1),new Grad(1,-1),new Grad(-1,-1),new Grad(1,0),new Grad(-1,0), new Grad(1,0),new Grad(-1,0),new Grad(0,1),new Grad(0,-1),new Grad(0,1),new Grad(0,-1)
];
var p = [151,160,137,91,90,15,
131,13,201,95,96,53,194,233,7,225,140,36,103,30,69,142,8,99,37,240,21,10,23,
190, 6,148,247,120,234,75,0,26,197,62,94,252,219,203,117,35,11,32,57,177,33,
88,237,149,56,87,174,20,125,136,171,168, 68,175,74,165,71,134,139,48,27,166,
77,146,158,231,83,111,229,122,60,211,133,230,220,105,92,41,55,46,245,40,244,
102,143,54, 65,25,63,161, 1,216,80,73,209,76,132,187,208, 89,18,169,200,196,
135,130,116,188,159,86,164,100,109,198,173,186, 3,64,52,217,226,250,124,123,
5,202,38,147,118,126,255,82,85,212,207,206,59,227,47,16,58,17,182,189,28,42,
223,183,170,213,119,248,152, 2,44,154,163, 70,221,153,101,155,167, 43,172,9,
129,22,39,253, 19,98,108,110,79,113,224,232,178,185, 112,104,218,246,97,228,
251,34,242,193,238,210,144,12,191,179,162,241, 81,51,145,235,249,14,239,107,
49,192,214, 31,181,199,106,157,184, 84,204,176,115,121,50,45,127, 4,150,254,
138,236,205,93,222,114,67,29,24,72,243,141,128,195,78,66,215,61,156,180];
var perm = new Array(512);
var gradPerm = new Array(512);
// basic seeding
function updateSeed(seed) { if(seed > 0 && seed < 1) { seed *= 65536; } seed = Math.floor(seed); if(seed < 256) { seed |= seed << 8; } for(var i = 0; i < 256; i++) { var v; if (i & 1) { v = p[i] ^ (seed & 255); } else { v = p[i] ^ ((seed>>8) & 255); } perm[i] = perm[i + 256] = v; gradPerm[i] = gradPerm[i + 256] = grads[v % 12]; }
};
// interpolation and easing
function lerp(a, b, t) { return (1-t)*a + t*b;
}
function smootherstep(n) { return n*n*n*(n*(n*6-15)+10);
}
// 2d Perlin noise
function perlin(x, y) { // cell position var X = Math.floor(x), Y = Math.floor(y); x = x - X; y = y - Y; X = X & 255; Y = Y & 255; // noise corner contributions var n00 = gradPerm[X+perm[Y]].dot2(x, y); var n01 = gradPerm[X+perm[Y+1]].dot2(x, y-1); var n10 = gradPerm[X+1+perm[Y]].dot2(x-1, y); var n11 = gradPerm[X+1+perm[Y+1]].dot2(x-1, y-1); // interpolate var u = smootherstep(x); return lerp(lerp(n00, n10, u), lerp(n01, n11, u), smootherstep(y));
};
/* * Draw on canvas */
// renders perlin noise on canvas with given ease function applied to value
function drawCanvas() { canvas.width = canvas.height = opts.imageSize; var ctx = canvas.getContext('2d'); var image = ctx.createImageData(opts.imageSize, opts.imageSize); var data = image.data; var selectedGradient = opts.gradientChoices[opts.outputGradient]; for (var x = 0; x < canvas.width; x++) { for (var y = 0; y < canvas.height; y++) { var value = opts.valueOffset; for (var o = 0; o < opts.octaves.length; o++) { var cellSize = opts.imageSize / opts.octaves[o].freq; var perlinVal = perlin(x / cellSize, y / cellSize); perlinVal = 2 * opts.easeFunction(0.5*perlinVal + 0.5) - 1; // magic~~ value += perlinVal * opts.valueMultiplier * opts.octaves[o].amp; } value = Math.max(0.0, Math.min(1.0, value / 256)); var gradPos = value / (1 / (selectedGradient.length - 1)); var colorA = selectedGradient[Math.floor(gradPos)]; var colorB = selectedGradient[Math.floor(gradPos) + 1]; gradPos -= ~~gradPos; var bmdCell = (x + y * canvas.width) * 4; data[bmdCell] = lerp(colorA >> 16 & 0xff, colorB >> 16 & 0xff, gradPos) & 0xff; data[bmdCell + 1] = lerp(colorA >> 8 & 0xff, colorB >> 8 & 0xff, gradPos) & 0xff; data[bmdCell + 2] = lerp(colorA & 0xff, colorB & 0xff, gradPos) & 0xff; data[bmdCell + 3] = 255; // alpha } } ctx.putImageData(image, 0, 0);
}
/* * START! */
function resizeCanvas() { var bodyDims = document.body.getBoundingClientRect(); var transformString; transformString = 'scale('+(Math.min(bodyDims.width, bodyDims.height) / opts.imageSize)+')'; canvas.style.webkitTransform = transformString; canvas.style.MozTransform = transformString; canvas.style.msTransform = transformString; canvas.style.OTransform = transformString; canvas.style.transform = transformString; transformString = 'scale('+(Math.min(bodyDims.width/768, bodyDims.height/512))+')'; presetsContainer.style.webkitTransform = transformString; presetsContainer.style.MozTransform = transformString; presetsContainer.style.msTransform = transformString; presetsContainer.style.OTransform = transformString; presetsContainer.style.transform = transformString;
}
var Options = function() { this.imageSize = 256; this.valueMultiplier = 1.0; this.valueOffset = 128; this.octaves = [ { amp: 128, freq: 4 }, { amp: 64, freq: 8 }, { amp: 32, freq: 16 }, { amp: 16, freq: 32 }, { amp: 8, freq: 64 }, { amp: 4, freq: 128 }, ]; this.easing = 'Linear'; this.easeFunction = recurve.ease.Linear; this.outputGradient = 'flame'; this.gradientChoices = { 'grayscale': [ 0x000000, 0xffffff, ], 'flame': [ 0x000000, 0x210303, 0x420707, 0xb80d00, 0xfba012, 0xffeb21, 0xffffcf, ], 'severity': [ 0x006837, 0x1bb520, 0xfcee21, 0xf16f24, 0xeb232b, 0xfcfcfc, ], 'elevation': [ 0x169231, 0x76b844, 0xfcf053, 0xf7a648, 0xad7e52, 0xfaf0e6, ], 'ocean': [ 0x040e1a, 0x0e3a57, 0x0071bc, 0x29abe2, 0x91c8e2, 0xf0f4ff, ], 'forest': [ 0x423127, 0x73643f, 0x0a781d, 0x188730, 0x7ede66, 0xd8ff6e ], 'bubblegum': [ 0xe39a9a, 0xe36b97, 0xfa5f8e, 0x5215b5, 0xfa5f8e, 0xe36b97, 0xe39a9a, 0xf0e65e, 0xe36b97, 0xfa5f8e, 0xe39a9a, 0x0071bc, 0xe39a9a, 0xfa5f8e, 0xe36b97, ], }; this.seed = Math.random(); this.randomizeSeed = () => { this.seed = Math.random(); updateSeed(this.seed); drawCanvas(); }; this.reset = () => { this.easing = 'Linear'; this.easeFunction = recurve.ease.Linear; this.outputGradient = 'flame'; this.valueMultiplier = 1.0; this.valueOffset = 128; this.randomizeSeed(); drawCanvas(); };
};
var gui; //FOOBAR
function init() { canvas = document.querySelector('canvas'); presetsContainer = document.querySelector('.presets-container'); // Dat GUI Options opts = new Options(); gui = new dat.GUI({ load: { remembered: { "Default": {"0": {}}, "Terrain": {"0": { "easing": "Bounce.inOut", "outputGradient": "elevation", "valueMultiplier": 0.8113886536833192, "valueOffset": 110, "seed": 0.5106456165922355 }}, "Infrared": {"0": { "easing": "Circ.inOut", "outputGradient": "severity", "valueMultiplier": 1.0552497883149874, "valueOffset": 126, "seed": 0.9145358022463355 }}, "Combustion": {"0": { "easing": "Quad.in", "outputGradient": "flame", "valueMultiplier": 1.583615580016935, "valueOffset": 304, "seed": 0.11567481377018551 }}, "X-Ray": {"0": { "easing": "Bounce.out", "outputGradient": "grayscale", "valueMultiplier": 0.6894580863674852, "valueOffset": -57, "seed": 0.7006360587264471 }}, "Cloudy": {"0": { "easing": "Quint.out", "outputGradient": "ocean", "valueMultiplier": 1.2178238780694328, "valueOffset": -35, "seed": 0.9662128918639274 }}, "Radiation": {"0": { "easing": "Circ.in", "outputGradient": "bubblegum", "valueMultiplier": 1.2178238780694328, "valueOffset": 309, "seed": 0.8725990287820224 }}, } } }); gui.add(opts, 'easing', [ 'Quad.in', 'Quad.out', 'Quad.inOut', 'Cubic.in', 'Cubic.out', 'Cubic.inOut', 'Quart.in', 'Quart.out', 'Quart.inOut', 'Quint.in', 'Quint.out', 'Quint.inOut', 'Sine.in', 'Sine.out', 'Sine.inOut', 'Circ.in', 'Circ.out', 'Circ.inOut', 'Expo.in', 'Expo.out', 'Expo.inOut', 'Back.in', 'Back.out', 'Back.inOut', 'Elastic.in', 'Elastic.out', 'Elastic.inOut', 'Bounce.in', 'Bounce.out', 'Bounce.inOut', 'Linear' ]).onChange(function(easeSelection) { opts.easeFunction = recurve.ease; easeSelection.split('.').forEach(function(namePart) { opts.easeFunction = opts.easeFunction[namePart]; }); drawCanvas(); }).listen(); gui.add(opts, 'outputGradient', [ 'grayscale', 'flame', 'severity', 'elevation', 'ocean', 'forest', 'bubblegum', ]).onChange(drawCanvas) .listen(); gui.add(opts, 'valueMultiplier', 0.25, 4) .onFinishChange(drawCanvas) .listen(); gui.add(opts, 'valueOffset', -128, 384).step(1) .onFinishChange(drawCanvas) .listen(); gui.add(opts, 'seed', 0, 1) .onFinishChange(function() { updateSeed(opts.seed); drawCanvas(); }).listen(); gui.add(opts, 'randomizeSeed'); gui.add(opts, 'reset'); gui.remember(opts); updateSeed(opts.seed); drawCanvas(); window.addEventListener('resize', resizeCanvas); resizeCanvas(); document.querySelector('.message').classList.add('fade'); var presets = Array.prototype.slice.apply(presetsContainer.querySelectorAll('.preset')); presets.forEach(function(preset) { preset.addEventListener('click', function(evt) { presetsContainer.classList.add('hide'); gui.preset = evt.currentTarget.dataset.preset; }) }); var btnTogglePresets = document.querySelector('button.show-presets'); btnTogglePresets.addEventListener('click', function() { if (presetsContainer.classList.contains('hide')) { presetsContainer.classList.remove('hide'); } else { presetsContainer.classList.add('hide'); } }); presetsContainer.classList.add('hide');
}
init();
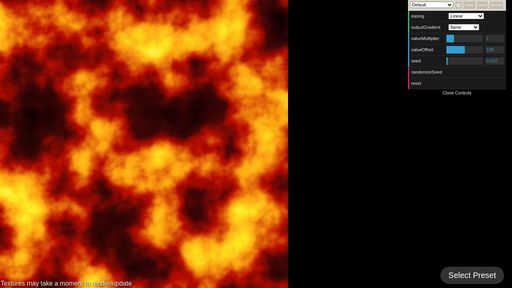
Developer | Chris Johnson |
Username | jhnsnc |
Uploaded | December 01, 2022 |
Rating | 3 |
Size | 6,714 Kb |
Views | 18,216 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Vector Star Eye | 1,788 Kb |
Text Effect - Optimist | 3,467 Kb |
Text Effect - Mystique | 3,405 Kb |
Web Animation Test | 2,721 Kb |
Incremental Delaunay Triangulation Demo | 8,204 Kb |
Shader Template | 3,345 Kb |
Headlander Title Animation | 11,702 Kb |
Text Effect - Radius | 3,762 Kb |
HAL9000 | 3,056 Kb |
F Loader | 1,981 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Alter bg opacity on hover... | Chrisboon27 | 2,054 Kb |
Resize image | Happyhj | 1,892 Kb |
Obligatory CSS3 UI Nav | Romandiaz | 9,017 Kb |
Portfolio Page | KaylaMT | 1,983 Kb |
Cartoon Bomb | Tcmulder | 4,929 Kb |
Scroll effect with text with help from Skrollr | Luxonglassing | 2,935 Kb |
Multi column experiment. | Spylefkaditis | 2,805 Kb |
Simple Weather App | Cmwebby | 0 Kb |
Funny menu | AxeLVaisper | 4,671 Kb |
700 Synapses Per Second | Silentkrange | 2,138 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!