Depth First Search Connectivity Test
How do I make an depth first search connectivity test?
What is a depth first search connectivity test? How do you make a depth first search connectivity test? This script and codes were developed by Teo Litto on 26 August 2022, Friday.
Depth First Search Connectivity Test - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Depth First Search Connectivity Test</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="instructions"> <h1>Connectivity check using DFS Algo</h1> <ol> <li>1) Click Check Connectivity button to find all lit squares connected to base squares</li> <li>2) Click Randomize button to generate a new random pattern</li> <li>3) Rinse and repeat</li> </ol> <button onclick="checkConnectivity()">Check Connectivity</button> <button onclick="resetGrid()">Randomize</button>
</div> <script src='https://cdnjs.cloudflare.com/ajax/libs/stats.js/r14/Stats.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Depth First Search Connectivity Test - Script Codes CSS Codes
html { background: #000; color: #fff;
}
canvas { display: block; margin: 0 auto; border: solid 1px #aaa;
}
#instructions { margin: 20px; float: left; max-width: 25%;
}
h1 { font-size: 1.1em
}
p { color: #fff; margin: 1em 0;
}
li{ margin: 1em 0;
}
button { font-size: 1em; margin: 3em 0;
}
Depth First Search Connectivity Test - Script Codes JS Codes
//config
cellWidth = 30; //cell width in px
cellHeight = 30; //cell height in px
gridWidth = 10; //grid width in cells
gridHeight = 10; //grid height in cells
litRatio = 0.35; //percent chance that a cell will be lit when randomized
checkDiagonals = true; // if true, we will consider squares at a diagonal to be connected. If false, only squares sharing an edge are connected.
//stats
var renderStats = new Stats();
renderStats.setMode(0); // 0: fps, 1: ms, 2: mb
renderStats.domElement.style.position = 'absolute';
renderStats.domElement.style.right = '0px';
renderStats.domElement.style.top = '0px';
document.body.appendChild(renderStats.domElement);
var loopStats = new Stats();
loopStats.setMode(1); // 0: fps, 1: ms, 2: mb
loopStats.domElement.style.position = 'absolute';
loopStats.domElement.style.right = '0px';
loopStats.domElement.style.top = '50px';
document.body.appendChild(loopStats.domElement);
//canvas
c = document.createElement('canvas');
c.width = gridWidth * cellWidth;
c.height = gridHeight * cellHeight;
ctx = c.getContext('2d');
document.body.appendChild(c);
//cell
Cell = function(x, y) { this.x = x; this.y = y; this.filled = 0; this.based = 0; this.visited = 0; this.falling = 0;
}
Grid = function() { var _g = createArray(gridWidth, gridHeight); for (var x = 0; x < gridWidth; x++) { for (var y = 0; y< gridHeight; y++) { _g[x][y] = new Cell(x,y); } } return _g;
}
function clearGrid() { for (var x = 0; x < gridWidth; x++) { for (var y = 0; y< gridHeight; y++) { grid[x][y] = new Cell(x,y); } }
}
function randomizeGrid() { for (var x = 0; x<gridWidth; x++) { for (var y = 0; y<gridHeight; y++) { //if y === 0, filled and based = 1 //else, filled is randomly 0 or 1 if (y == 0) { grid[x][y].filled = 1; grid[x][y].based = 1; } else { rand = (Math.random() > 1 - litRatio)? 1 : 0; //random chance to be filled grid[x][y].filled = rand } } }
}
function resetGrid() { clearGrid(); randomizeGrid();
}
function drawCell(x, y) { var cell = grid[x][y]; ctx.fillStyle = "rgb(50,50,50)" if (cell.filled) { ctx.fillStyle = "rgb(200,200,200)" } if (cell.based) { ctx.fillStyle = "rgb(0,255,0)" } if (cell.falling) { ctx.fillStyle = "rgb(255,0,0)" } ctx.fillRect( x * cellWidth, y * cellHeight, cellWidth, cellHeight )
}
function drawGrid() { for (var x = 0; x < gridWidth; x++) { for (var y = 0; y < gridHeight; y++) { drawCell(x, y); } }
}
function checkConnectivity() { loopStats.begin(); resetVisits(); totalCells = gridWidth * gridHeight; currentCell = grid[0][0]; currentCell.visited = 1; visitedCells = 1; cellStack = []; while (visitedCells < totalCells) { //console.log(currentCell.x,currentCell.y) //find all neighbors of currentCell that have not been visited var neighbors = []; //N if (grid[currentCell.x] && grid[currentCell.x][currentCell.y - 1] && grid[currentCell.x][currentCell.y - 1].filled === 1 && grid[currentCell.x][currentCell.y - 1].visited === 0 ) { neighbors.push(grid[currentCell.x][currentCell.y - 1]) }; //E if (grid[currentCell.x + 1] && grid[currentCell.x + 1][currentCell.y] && grid[currentCell.x + 1][currentCell.y] && grid[currentCell.x + 1][currentCell.y].filled === 1 && grid[currentCell.x + 1][currentCell.y].visited === 0 ) { neighbors.push(grid[currentCell.x + 1][currentCell.y]) }; //S if (grid[currentCell.x] && grid[currentCell.x][currentCell.y + 1] && grid[currentCell.x][currentCell.y + 1].filled === 1 && grid[currentCell.x][currentCell.y + 1].visited === 0 ) { neighbors.push(grid[currentCell.x][currentCell.y + 1]) }; //W if (grid[currentCell.x - 1] && grid[currentCell.x - 1][currentCell.y] && grid[currentCell.x - 1][currentCell.y].filled === 1 && grid[currentCell.x - 1][currentCell.y].visited === 0 ) { neighbors.push(grid[currentCell.x - 1][currentCell.y]) }; //if we're checking diagonals, check NE, NW, SE, SW as well. if (checkDiagonals) { //NE if (grid[currentCell.x + 1] && grid[currentCell.x + 1][currentCell.y - 1] && grid[currentCell.x + 1][currentCell.y - 1].filled === 1 && grid[currentCell.x + 1][currentCell.y - 1].visited === 0 ) { neighbors.push(grid[currentCell.x + 1][currentCell.y - 1]) }; //SE if (grid[currentCell.x + 1] && grid[currentCell.x + 1][currentCell.y + 1] && grid[currentCell.x + 1][currentCell.y + 1].filled === 1 && grid[currentCell.x + 1][currentCell.y + 1].visited === 0 ) { neighbors.push(grid[currentCell.x + 1][currentCell.y + 1]) }; //SW if (grid[currentCell.x - 1] && grid[currentCell.x - 1][currentCell.y + 1] && grid[currentCell.x - 1][currentCell.y + 1].filled === 1 && grid[currentCell.x - 1][currentCell.y + 1].visited === 0 ) { neighbors.push(grid[currentCell.x - 1][currentCell.y + 1]) }; //NW if (grid[currentCell.x - 1] && grid[currentCell.x - 1][currentCell.y - 1] && grid[currentCell.x - 1][currentCell.y - 1].filled === 1 && grid[currentCell.x - 1][currentCell.y - 1].visited === 0 ) { neighbors.push(grid[currentCell.x - 1][currentCell.y - 1]) }; } //if number of filled non-visited neighbors is non-zero if (neighbors.length) { //pick one at random var newCell = neighbors[Math.round(Math.random() * (neighbors.length-1))] //if current cell is filled and new cell is based, set current cell to based if (currentCell.filled && newCell.based) { currentCell.based = 1; } else if (currentCell.based && newCell.filled) { newCell.based = 1; } //set new cell to visited, push current cell to cellStack newCell.visited = 1; cellStack.push(currentCell); //set new cell to currentCell currentCell = newCell; //IMPORTANT - ADD 1 to VISITED CELLS OR WE WILL INFINITE LOOP visitedCells++ } else { //if cellStack has cells still on it, pull previous cell off cellStack into currentCell, try again from there. //else we've tried all options, break. if (cellStack.length) { currentCell = cellStack.pop(); } else { break; } } //repeat from new currentCell } //set all filled cells that are not based to falling for (var x = 0; x < gridWidth; x++) { for (var y = 0; y < gridHeight; y++) { var gc = grid[x][y]; if (gc.filled && !gc.based) { gc.falling = 1; } } } loopStats.end();
}
function resetVisits() { for (var x = 0; x < gridWidth; x++) { for (var y = 0; y < gridHeight; y++) { var gc = grid[x][y]; gc.visited = 0; } }
}
function render() { renderStats.begin(); drawGrid(); requestAnimationFrame(render); renderStats.end();
}
//kickoff
grid = new Grid();
randomizeGrid();
render();
//UTIL
function createArray(length) { //create multiDimensional arrays var arr = new Array(length || 0), i = length; if (arguments.length > 1) { var args = Array.prototype.slice.call(arguments, 1); while (i--) arr[length - 1 - i] = createArray.apply(this, args); } return arr;
}
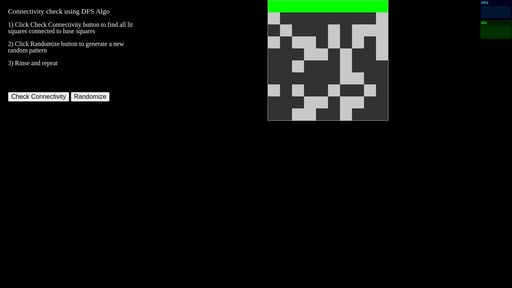
Developer | Teo Litto |
Username | teolitto |
Uploaded | August 26, 2022 |
Rating | 3 |
Size | 3,775 Kb |
Views | 20,240 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Parallaxadise - Quick n dirty scrollytelling demo | 8,928 Kb |
Powers Sniffer | 2,663 Kb |
Kitten Sniffer | 2,892 Kb |
Liquid Loader | 2,009 Kb |
Dreamforce.com 2017 | 11,454 Kb |
Color morphing | 3,764 Kb |
Lock Picking in the DOM | 5,847 Kb |
WebGL Smoke | 3,126 Kb |
Starfall | 3,863 Kb |
Konami Code Easter Egg | 3,051 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Fluid Responsive Typography | Jonmilner | 4,205 Kb |
Sketchy Box | Mnicpt | 3,033 Kb |
Test | Dviate | 2,668 Kb |
Automatic scroll | Skeurentjes | 4,042 Kb |
Compare resources on mobile sites | Gyusza | 3,226 Kb |
Flexbox Grid - equal height | DaveOrDead | 2,855 Kb |
CSS Org Chart | Appirio-ux | 3,882 Kb |
STAR WARS LIGHTSABER | Francoiscoron | 4,420 Kb |
Resume | Rottingroom | 5,483 Kb |
Custom Select Element | Agrayson | 3,616 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!