Drawing Application
How do I make an drawing application?
What is a drawing application? How do you make a drawing application? This script and codes were developed by Milos Stankovic on 14 September 2022, Wednesday.
Drawing Application - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Drawing Application</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <canvas width="600" height="400"></canvas> <div class="controls"> <ul> <li class="red selected"></li> <li class="blue"></li> <li class="yellow"></li> </ul> <button id="revealColorSelect">New Color</button> <div id="colorSelect"> <span id="newColor"></span> <div class="sliders"> <p> <label for="red">Red</label> <input id="red" name="red" type="range" min=0 max=255 value=0> </p> <p> <label for="green">Green</label> <input id="green" name="green" type="range" min=0 max=255 value=0> </p> <p> <label for="blue">Blue</label> <input id="blue" name="blue" type="range" min=0 max=255 value=0> </p> </div> <div> <button id="addNewColor">Add Color</button> </div> </div> </div> <script src="https://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script> <script src="js/index.js"></script>
</body>
</html>
Drawing Application - Script Codes CSS Codes
body { background: #384047; font-family: sans-serif;
}
canvas { background: #fff; display: block; margin: 50px auto 10px; border-radius: 5px; box-shadow: 0 4px 0 0 #222; cursor: url(../img/cursor.png), crosshair;
}
.controls { min-height: 60px; margin: 0 auto; width: 600px; border-radius: 5px; overflow: hidden;
}
ul { list-style:none; margin: 0; float: left; padding: 10px 0 20px; width: 100%; text-align: center;
}
ul li, #newColor { display:block; height: 54px; width: 54px; border-radius: 60px; cursor: pointer; border: 0; box-shadow: 0 3px 0 0 #222;
}
ul li { display: inline-block; margin: 0 5px 10px;
}
.red { background: #fc4c4f;
}
.blue { background: #4fa3fc;
}
.yellow { background: #ECD13F;
}
.selected { border: 7px solid #fff; width: 40px; height: 40px;
}
button { background: #68B25B; box-shadow: 0 3px 0 0 #6A845F; color: #fff; outline: none; cursor: pointer; text-shadow: 0 1px #6A845F; display: block; font-size: 16px; line-height: 40px;
}
#revealColorSelect { border: none; border-radius: 5px; margin: 10px auto; padding: 5px 20px; width: 160px;
}
/* New Color Palette */
#colorSelect { background: #fff; border-radius: 5px; clear: both; margin: 20px auto 0; padding: 10px; width: 305px; position: relative; display:none;
}
#colorSelect:after { bottom: 100%; left: 50%; border: solid transparent; content: " "; height: 0; width: 0; position: absolute; pointer-events: none; border-color: rgba(255, 255, 255, 0); border-bottom-color: #fff; border-width: 10px; margin-left: -10px;
}
#newColor { width: 80px; height: 80px; border-radius: 3px; box-shadow: none; float: left; border: none; margin: 10px 20px 20px 10px;
}
.sliders p { margin: 8px 0; vertical-align: middle;
}
.sliders label { display: inline-block; margin: 0 10px 0 0; width: 35px; font-size: 14px; color: #6D574E;
}
.sliders input { position: relative; top: 2px;
}
#colorSelect button { border: none; border-top: 1px solid #6A845F; border-radius: 0 0 5px 5px; clear: both; margin: 10px -10px -7px; padding: 5px 10px; width: 325px;
}
Drawing Application - Script Codes JS Codes
// get the color of selected color button
var color = $(".selected").css("background-color");
// cache canvas element
var $canvas = $("canvas");
// get 2d context of canvas
var context = $canvas[0].getContext("2d");
var lastEvent;
// on load, make sure mouse is not clicked
var mouseDown = false;
// when color button is clicked
$(".controls").on("click", "li", function(){ // remove selected class from current $(this).siblings().removeClass("selected"); // set selected class to the clicked button $(this).addClass("selected"); // get the color of the clicked button color = $(this).css("background-color");
});
// on click on #revealColorSelect button...
$("#revealColorSelect").click(function(){ // runs function that make r, g and b vars from appropriate selectors changeColor(); // ...open/close select color pane $("#colorSelect").toggle();
});
// make r, g and b vars from appropriate selectors (type=range)
function changeColor() { var r = $("#red").val(); var g = $("#green").val(); var b = $("#blue").val(); // set value to the span#newColor from r,g and b values $("#newColor").css("background-color", "rgb(" + r + "," + g + "," + b + ")");
}
// on each change on any of rgb selectors, run changeColor() to set value to span#newColor
$("input[type=range]").change(changeColor);
// when click #addNewColor button, we need to add new color to the .controls ul
$("#addNewColor").click(function(){ // make new $ object that we'll append var $newColor = $("<li></li>"); // it's background color will be set to what we selected using color selectors r,g and b $newColor.css("background-color", $("#newColor").css("background-color")); // append it $(".controls ul").append($newColor); //make it selected as soon as it's added $newColor.click();
});
/*
$canvas.mousedown(function(e) { // last event will become an object with plenty of properties // enter lastEvent in console after mouse click to see the object lastEvent = e; mouseDown = true;
}).mousemove(function(e){ // we draw only when mouse is clicked if(mouseDown){ context.beginPath(); context.moveTo(lastEvent.offsetX,lastEvent.offsetY); context.lineTo(e.offsetX,e.offsetY); context.strokeStyle = color; context.stroke(); lastEvent = e; }
}).mouseup(function(){ // we are not drawing anymore when mouse is up mouseDown = false;
}).mouseleave(function(){ // prevent drawing when mouse is not within canvas $canvas.mouseup();
});*/
$canvas.mousedown(function(e) { // last event will become an object with plenty of properties // enter lastEvent in console after mouse click to see the object lastEvent = e; mouseDown = true;
}).mousemove(function(e){ // we draw only when mouse is clicked if(mouseDown){ }
}).mouseup(function(e){ // we are not drawing anymore when mouse is up context.beginPath(); context.moveTo(lastEvent.offsetX,lastEvent.offsetY); context.lineTo(e.offsetX,e.offsetY); context.strokeStyle = color; context.stroke(); lastEvent = e;
}).mouseleave(function(){ // prevent drawing when mouse is not within canvas $canvas.mouseup();
});
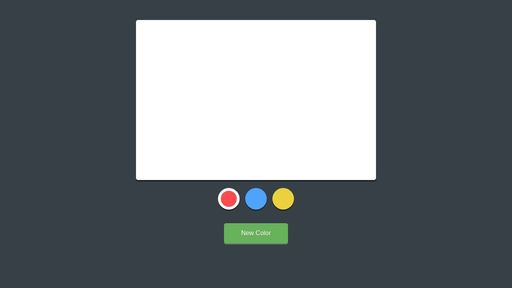
Developer | Milos Stankovic |
Username | milstanyu |
Uploaded | September 14, 2022 |
Rating | 3 |
Size | 3,534 Kb |
Views | 20,240 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Login Form | 2,838 Kb |
FUNCTIONS Intro | 2,261 Kb |
Students Database | 2,174 Kb |
Color Guess Game | 2,795 Kb |
Responsive Menu | 2,539 Kb |
Search Stock | 1,680 Kb |
While loop that show n random numbers | 1,434 Kb |
Lightbox | 2,798 Kb |
Objects | 1,936 Kb |
Array | 1,753 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Google Chrome Icon using Pure CSS in one DIV | Grssam | 3,627 Kb |
Easing | GreenSock | 2,043 Kb |
Disable JavaScript execution from console | Ludviglindblom | 2,534 Kb |
Cartoon Bomb | Tcmulder | 4,929 Kb |
Pure CSS Animated Photo Stack | Depthdev | 2,486 Kb |
Angular Route | Arun_v606 | 1,837 Kb |
Cant get enough icecream in pure css3 | Melawire | 4,322 Kb |
Word Wrap Algorithm for Multiline Canvas Text | Peterhry | 2,349 Kb |
Nav Test -- cats 1 | Payls | 4,735 Kb |
A Pen by Ben Babics | Benbabics | 2,957 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!