Generic Stock
How do I make an generic stock?
What is a generic stock? How do you make a generic stock? This script and codes were developed by Andy Vanee on 21 September 2022, Wednesday.
Generic Stock - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Generic Stock</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="historical-data-graph"> <div class="container"></div>
</div> <script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.0/jquery.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/d3/3.5.17/d3.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Generic Stock - Script Codes CSS Codes
.container { max-width: 975px; margin: 0 auto;
}
.historical-data-graph .graph-container { border: 1px solid transparent;
}
.historical-data-graph .axis path,
.historical-data-graph .axis line { fill: none; stroke: #000; shape-rendering: crispEdges;
}
.historical-data-graph .y.axis path,
.historical-data-graph .x.axis path { stroke-width: 2px; stroke: rgba(0, 0, 0, 0.2);
}
.historical-data-graph rect { fill: transparent;
}
.historical-data-graph .bar { fill: #888; stroke: #888; stroke-width: 16px;
}
.historical-data-graph .line { fill: none; stroke: #888; stroke-width: 16px; stroke-miterlimit: 10; stroke-linejoin: miter;
}
.historical-data-graph .vol, .historical-data-graph .close { text-anchor: middle; background-color: black; font-size: 24px;
}
.historical-data-graph .vline { display: none; fill: #888;
}
.historical-data-graph .close-box { fill: #fadf6e; stroke: transparent; stroke-width: 2px;
}
.historical-data-graph .overlay { fill: none; pointer-events: all; cursor: crosshair;
}
.historical-data-graph .point { fill: #888; opacity: 0;
}
.historical-data-graph .focus { display: none;
}
.historical-data-graph .focus circle { fill: none; stroke: steelblue;
}
.historical-data-graph .focus text { fill: black; font-weight: bold; font-size: 15px;
}
.historical-data-graph .tick line { stroke-width: 2px; stroke: rgba(0, 0, 0, 0.2);
}
.historical-data-graph .tick text { fill: black; font-weight: bold; font-size: 12px;
}
Generic Stock - Script Codes JS Codes
'use strict';
$(function () { var csv_source = "https://gist.githubusercontent.com/andyvanee/789b9dd9cfe4f337eaafbd9392c045d6/raw/d8ac8772d85aadf9e14bac3fda446968a279fb4c/graph.csv"; var graph = { top: 20, right: 20, bottom: 70, left: 30, outerWidth: 650, outerHeight: 500, width: function width() { return graph.outerWidth - graph.left - graph.right; }, height: function height() { return graph.outerHeight - graph.top - graph.bottom; } }, volume_graph = { 'height': 0, 'width': 725 }; var lineTransition = d3.transition().duration(1).ease('linear'); var parseDate = d3.time.format("%Y-%m-%d").parse, bisectDate = d3.bisector(function (d) { return d.date; }).left, formatValue = d3.format(",.2f"), formatCurrency = function formatCurrency(d) { return "$" + formatValue(d); }; var x = d3.time.scale().range([0, graph.width()]); var xvol = d3.time.scale().range([0, graph.width()]); var y = d3.scale.linear().range([graph.height(), 0]); var yvol = d3.scale.linear().range([volume_graph.height, 0]); var xAxis = d3.svg.axis().scale(x).orient("bottom").ticks(d3.time.years, 1); var yAxis = d3.svg.axis().scale(y).orient("left").ticks(2).tickFormat(function (d) { return "$" + d; }); var line = d3.svg.line().x(function (d) { return x(d.date); }).y(function (d) { return y(d.close); }); var svg = d3.select(".container").append("svg").attr("class", "graph-container").attr("width", graph.outerWidth - 5).attr("height", graph.outerHeight).append("g").attr("transform", "translate(" + graph.left + "," + graph.top + ")"); var gradient = d3.select(".graph-container").append("defs").append("linearGradient").attr("id", "gradient"); gradient.append("stop").attr("offset", "0%").attr("stop-color", "#018442").attr("stop-opacity", 1); gradient.append("stop").attr("offset", "100%").attr("stop-color", "#005229").attr("stop-opacity", 1); d3.csv(csv_source, function (error, data) { if (error) throw error; data.forEach(function (d) { d.date = parseDate(d.Date); d.close = +d.Close; d.vol = +d.Volume; }); data.sort(function (a, b) { return a.date - b.date; }); x.domain([data[0].date, data[data.length - 1].date]); xvol.domain([data[0].date, data[data.length - 1].date]); y.domain(d3.extent(data, function (d) { return d.close; })).nice(4); yvol.domain(d3.extent(data, function (d) { return d.vol; })); var volumeGraph = d3.select('.graph-container').append('g').attr('class', 'volume-graph').attr("transform", "translate(" + graph.left + "," + (graph.outerHeight - volume_graph.height) + ")"); volumeGraph.selectAll(".bar").data(data).enter().append("rect").attr("class", "bar").attr("x", function (d) { return xvol(d.date); }).attr("width", 3).attr("y", function (d) { return yvol(d.vol); }).attr("height", function (d) { return volume_graph.height - yvol(d.vol); }); svg.append("g").attr("class", "x axis").attr("transform", "translate(0," + graph.height() + ")").call(xAxis); svg.append("g").attr("class", "y axis").call(yAxis); svg.append("path").datum(data).attr("class", "line").attr("d", line).attr("transform", "translate(2, 0)"); // .style("stroke", "url(#gradient)"); // // circles for discrete points on line // // svg.selectAll('.point') // .data(data) // .enter().append("circle") // .attr("class", "point") // .attr("cx", function(d) { // return x(d.date); // }) // .attr("cy", function(d) { // return y(d.close); // }) // .attr("r", 3); var focus = svg.insert("g").attr("class", "focus").style("display", "none"); var vline = svg.append('rect').attr('class', 'vline').attr('x', 0).attr('y', 0).attr('width', 1).attr('height', graph.outerHeight); focus.append("rect").attr('class', 'close-box').attr('width', 80).attr('height', 36); focus.append("text").attr('class', 'close').attr("x", 40).attr("y", 11).attr("dy", ".35em"); focus.append("text").attr('class', 'vol').attr("x", 40).attr("y", 25).attr("dy", ".35em"); svg.append("rect").attr("class", "overlay").attr("width", graph.width()).attr("height", graph.outerHeight).on("mouseover", function () { focus.style("display", null); }).on("mouseout", function () { // focus.style("display", "none"); }).on("mousemove", mousemove); function mousemove() { var x0 = x.invert(d3.mouse(this)[0]), i = bisectDate(data, x0, 1), d0 = data[i - 1], d1 = data[i], d = x0 - d0.date > d1.date - x0 ? d1 : d0, x_translate = x(d.date); if (x_translate > graph.width() / 2) { x_translate -= 80; } focus.attr("transform", "translate(" + x_translate + "," + y(d.close) + ")"); vline.attr("transform", 'translate(' + x(d.date) + ',0)'); focus.select(".close").text(formatCurrency(d.close)); focus.select(".vol").text(d.vol); } });
});
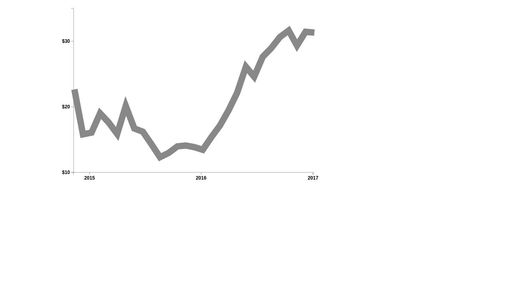
Developer | Andy Vanee |
Username | andyvanee |
Uploaded | September 21, 2022 |
Rating | 3 |
Size | 6,058 Kb |
Views | 22,264 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Ticker Example | 8,789 Kb |
Parallax Backgrounds | 4,281 Kb |
MaybeOn | 3,129 Kb |
Ambient Animation | 4,186 Kb |
CSS Blend Modes 1 | 2,911 Kb |
Some perspective 2 | 4,282 Kb |
Harmoniclock-16 | 3,278 Kb |
Responsive Banner with VW | 1,688 Kb |
Basic Flexbox | 2,451 Kb |
Harmoniclock | 2,966 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
3D-box | Parthviroja | 2,346 Kb |
Navier Stoke Fluid Simulation | Esimov | 8,584 Kb |
Video mute | Leon9208 | 2,131 Kb |
Bootstrap example | Ssaakkaa | 2,716 Kb |
Button fills | Zubfatal | 5,205 Kb |
Highbrow Basic HTML Lesson 7 | Kimlarocca | 1,662 Kb |
Testing Portfolio Page | Sideshowli | 3,395 Kb |
Mini Profile | Frytyler | 3,828 Kb |
Main page display | BarryKe | 4,562 Kb |
Importable Clearfix | Corysimmons | 1,411 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!