JS canvas 3d tree generator
How do I make an js canvas 3d tree generator?
Click to make a new one. I just love trees. this is 2d canvas btw, no libraries. What is a js canvas 3d tree generator? How do you make a js canvas 3d tree generator? This script and codes were developed by Matei Copot on 15 December 2022, Thursday.
JS canvas 3d tree generator - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>JS canvas 3d tree generator</title> <style> /* NOTE: The styles were added inline because Prefixfree needs access to your styles and they must be inlined if they are on local disk! */ canvas { position: absolute; top: 0; left: 0;
} </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/prefixfree/1.0.7/prefixfree.min.js"></script>
</head>
<body> <canvas id=c></canvas> <script src="js/index.js"></script>
</body>
</html>
JS canvas 3d tree generator - Script Codes CSS Codes
canvas { position: absolute; top: 0; left: 0;
}
JS canvas 3d tree generator - Script Codes JS Codes
var w = c.width = window.innerWidth, h = c.height = window.innerHeight, ctx = c.getContext( '2d' ), opts = { speed: 1, splitSizeProbabilityMultiplier: 1/1000, maxIterations: 8, startSize: 20, baseSizeMultiplier: .7, addedSizeMultiplier: .2, baseAngleVariation: -Math.PI / 16, addedAngleVariation: Math.PI / 8, angleVariationIterationMultiplier: .8, baseLeafSize: 20, addedLeafSize: 15, rotYVel: .01, focalLength: 250, vanishPoint: { x: w / 2, y: h / 2 }, translations: { x: 0, y: 200, z: 400 } }, rotY = 0, rotYsin = 0, rotYcos = 1, lines = [];
function Line( parent ){ this.iteration = parent.iteration + 1; this.start = parent.end; this.angle = { a: parent.angle.a + this.iteration * opts.angleVariationIterationMultiplier * ( opts.baseAngleVariation + opts.addedAngleVariation * Math.random() ), b: parent.angle.b + this.iteration * opts.angleVariationIterationMultiplier * ( opts.baseAngleVariation + opts.addedAngleVariation * Math.random() ), }; this.size = ( opts.baseSizeMultiplier + opts.addedSizeMultiplier * Math.random() ) * parent.size; this.color = 'hsla(hue,80%,50%,alp)' .replace( 'hue', ( 1 - this.iteration / opts.maxIterations ) * 40 ) .replace( 'alp', 1 - ( this.iteration / opts.maxIterations ) * .9 ); var sinA = Math.sin( this.angle.a ), sinB = Math.sin( this.angle.b ), cosA = Math.cos( this.angle.a ), cosB = Math.cos( this.angle.b ); this.speed = { x: opts.speed * cosA * sinB, y: opts.speed * sinA * sinB, z: opts.speed * cosB }; this.end = this.closest = new Point( this.start.x, this.start.y, this.start.z ); this.done = false; this.time = 0;
}
Line.prototype.update = function(){ if( !this.done ){ this.end.x += this.speed.x; this.end.y += this.speed.y; this.end.z += this.speed.z; this.time += .1; if( Math.random() < this.size * opts.splitSizeProbabilityMultiplier || this.time > this.size ){ if( this.iteration < opts.maxIterations ){ lines.push( new Line( this ) ); lines.push( new Line( this ) ); } else { lines.push( new Leaf( this ) ); } this.done = true; } } // some lines can share their start if( this.start.hasntCalculatedScreen ) this.start.calculateScreen(); // but not their end this.end.calculateScreen(); this.closest = this.start; if( this.end.transformed.z < this.start.transformed.z ) this.closest = this.end;
}
Line.prototype.render = function(){ ctx.strokeStyle = this.color; ctx.lineWidth = this.size * this.start.screen.scale; ctx.beginPath(); ctx.moveTo( this.start.screen.x, this.start.screen.y ); ctx.lineTo( this.end.screen.x, this.end.screen.y ); ctx.stroke(); this.start.hasntCalculatedScreen = this.end.hasntCalculatedScreen = true;
}
function Leaf( parent ){ this.point = this.closest = parent.end; this.size = opts.baseLeafSize + opts.addedLeafSize * Math.random(); this.time = -Math.PI / 2; this.speed = .03 + .03 * Math.random(); this.color = 'hsla(hue,80%,50%,.5)' .replace( 'hue', 80 + 20 * Math.random() );
}
Leaf.prototype.update = function(){ this.time += this.speed;
}
Leaf.prototype.render = function(){ ctx.fillStyle = this.color; var size = ( Math.sin( this.time ) / 2 + .5 ) * this.size * this.point.screen.scale; ctx.fillRect ( this.point.screen.x - size / 2, this.point.screen.y - size / 2, size, size )
}
function Point( x, y, z ){ this.x = x; this.y = y; this.z = z; this.screen = {}; this.transformed = {}; this.hasntCalculatedScreen = true;
}
Point.prototype.calculateScreen = function(){ var x = this.x, y = this.y, z = this.z; // rotate around Y var X = x; x = x * rotYcos - z * rotYsin; z = z * rotYcos + X * rotYsin; // translate x += opts.translations.x; y += opts.translations.y; z += opts.translations.z; // I only need the z for now this.transformed.z = z; this.screen.scale = opts.focalLength / z; this.screen.x = opts.vanishPoint.x + x * this.screen.scale; this.screen.y = opts.vanishPoint.y + y * this.screen.scale; this.hasntCalculatedScreen = false;
}
function anim(){ window.requestAnimationFrame( anim ); ctx.fillStyle = 'white'; ctx.fillRect( 0, 0, w, h ); rotY += opts.rotYVel; rotYcos = Math.cos( rotY ); rotYsin = Math.sin( rotY ); lines.map( function( line ){ line.update(); } ); lines.sort( function( a, b ){ return b.closest.transformed.z - a.closest.transformed.z } ); lines.map( function( line ){ line.render(); } );
}
lines.push( new Line( { end: new Point( 0, 0, 0 ), angle: { a: Math.PI/2, b: -Math.PI / 2 }, size: opts.startSize, iteration: 0 }
) );
anim();
window.addEventListener( 'click', function(){ lines.length = 0; lines.push( new Line( { end: new Point( 0, 0, 0 ), angle: { a: Math.PI/2, b: -Math.PI / 2 }, size: opts.startSize, iteration: 0 } ) );
})
window.addEventListener( 'resize', function(){ w = c.width = window.innerWidth; h = c.height = window.innerHeight; opts.vanishPoint.x = w / 2; opts.vanishPoint.y = h / 2;
})
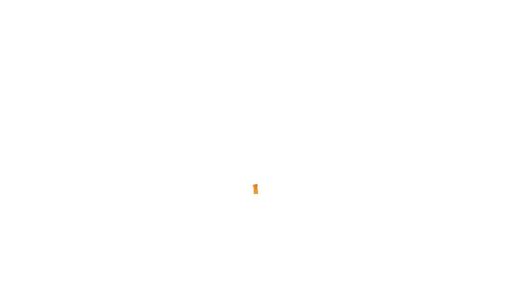
Developer | Matei Copot |
Username | towc |
Uploaded | December 15, 2022 |
Rating | 4 |
Size | 3,290 Kb |
Views | 12,144 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Neon hexagon-forming particles | 2,821 Kb |
Turret of Order | 4,000 Kb |
Rainbow galaxy | 3,633 Kb |
Organic lines of staring-atness | 2,415 Kb |
Rainbow Firestorm recreation | 3,567 Kb |
Pacman | 3,859 Kb |
Random curve-pathed particles of rainbowness | 2,576 Kb |
Invasion of creepy crawly rainbow fireflies | 3,094 Kb |
Plasma background | 3,338 Kb |
Eiffel Tower | 3,692 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Update CSS Variables with JS | Wesbos | 2,335 Kb |
STAR WARS LIGHTSABER | Francoiscoron | 4,420 Kb |
Sass Radar | Jlong | 6,887 Kb |
Ripples in water | Nobitagit | 2,704 Kb |
Table border-collapse property | Maxds | 1,672 Kb |
Animated Slide Hamburger Mobile Menu | BJack | 2,247 Kb |
Google Maps API Ground Overlay | Boycetrus | 2,961 Kb |
Typefaces with descriptions | Megandoty | 2,944 Kb |
Filtering with shuffle.js | Deyand | 2,712 Kb |
Svg sky | Omodev | 7,070 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!