Quick Weather App
How do I make an quick weather app?
Next freeCodeCamp assignment. User stories: 1. I can see the weather in my current location. 2. I can see a different icon or background image (e.g. snowy mountain, hot desert) depending on the weather.. What is a quick weather app? How do you make a quick weather app? This script and codes were developed by Tomasz on 10 August 2022, Wednesday.
Quick Weather App - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Quick Weather App</title> <link href='https://fonts.googleapis.com/css?family=Montserrat:400,700' rel='stylesheet' type='text/css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <header> <div class="container"> <div id="brand"> <h1><span>Quick</span>Weather</h1> </div> <div id="toggle-unit"> <div id="unit">Celsius</div> <div id="button-toggle"> <div id="selector"></div> </div> </div> </div>
</header>
<p id="info">Please, enable <strong>geolocation</strong> in your browser to use the full functionality of the page.</p>
<div id="display"> <div class="container"> <div id="location"> <div class="description"> Location </div> <div id="city"> City, Country </div> </div> <div class="line"></div> <div class="column"> <div id="weather-desc"> <div class="description"> Weather conditions </div> <div id="conditions" class="answer"> Conditions </div> </div> <div id="humidity"> <div class="description"> Humidity </div> <div id="hum" class="answer"> Conditions </div> </div> <div id="clouds"> <div class="description"> Cloudiness </div> <div id="cloudiness" class="answer"> Conditions </div> </div> </div> <div class="column"> <div id="temperature"> <div class="description"> Temperature </div> <div id="temp"> 0°C </div> </div> </div> </div>
</div> <script src='https://code.jquery.com/jquery-2.2.4.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Quick Weather App - Script Codes CSS Codes
* { font-family: "Montserrat", sans-serif;
}
body { margin: 0; background: #c9cfd6; background-repeat: no-repeat; background-position: bottom center; background-size: cover; background-attachment: fixed;
}
header { background-color: #f7f7ff; width: 100%; z-index: 1;
}
header h1 { display: inline-block; text-transform: uppercase; letter-spacing: 0.05em; font-size: 1em; color: #424b54;
}
header h1 span { color: #72b01d;
}
header #toggle-unit #unit { color: #424b54; font-size: 10pt;
}
header #toggle-unit #button-toggle { width: 2em; height: 1em; background-color: #e8edf2; border: 3px solid #c9cfd6; cursor: pointer;
}
header #toggle-unit #button-toggle #selector { position: relative; width: 1em; height: 1em; background-color: #72b01d;
}
#display { background-color: #424b54; opacity: 0.9; color: #f7f7ff; font-size: 36pt;
}
.line { width: 100%; height: 2px; background-color: #c9cfd6;
}
.description { font-size: 10pt; color: #c9cfd6;
}
#temperature { font-size: 80pt;
}
.column { width: 50%;
}
.answer { font-size: 18pt; margin-top: 0.2rem; margin-bottom: 1rem;
}
#info { color: #424b54; text-align: center;
}
#info strong { color: #72b01d;
}
.container { margin: 0 auto; max-width: 640px; padding: 1rem 2rem; display: flex; flex-wrap: wrap; justify-content: space-between;
}
#toggle-unit { display: flex; justify-content: space-between; align-items: center;
}
#toggle-unit #unit { margin-right: 1em;
}
.line { margin-bottom: 2.5rem;
}
#display { height: 100%; padding-top: 1rem;
}
#location { margin-bottom: 2rem;
}
#location { width: 100%;
}
.column { margin-bottom: 1rem;
}
#temp { margin-top: -0.5rem;
}
#info { margin: 5rem auto; max-width: 15rem;
}
Quick Weather App - Script Codes JS Codes
var weatherMemberKey = '243312e469ebf37a284f9e14fbfac6b8';
var apiHTML = 'http://api.openweathermap.org/data/2.5/'
var temperature = 0;
function toFahrenheit(temp) { return Math.round(temp * 1.8 + 32);
}
function toCelsius(temp) { return Math.round((temp -32) / 1.8);
}
function firstToUpper(str) { return str[0].toUpperCase() + str.substr(1);
}
function toggleUnit() { $("#button-toggle").on('click', function() { $("#selector").toggleClass("switched"); if($("#selector").hasClass("switched")) { $("#unit").html("Fahrenheit"); temperature = toFahrenheit(temperature); $("#temp").html(temperature + "°F"); $("#selector").animate({right: '-=1em'}, 300); } else { $("#unit").html("Celsius"); temperature = toCelsius(temperature); $("#temp").html(temperature + "°C"); $("#selector").animate({right: '+=1em'}, 300); } });
}
function changeBackground(desc) { var url; switch(desc) { case 'scattered clouds': url = "https://images.unsplash.com/photo-1430950716796-677ecbc99485?dpr=1&auto=format&crop=entropy&fit=crop&w=1500&h=1000&q=80"; break; case 'clear sky': url = "https://images.unsplash.com/photo-1464495310703-83aac94583ee?dpr=1&auto=format&crop=entropy&fit=crop&w=1500&h=1125&q=80"; break; case 'broken clouds': break; case 'few clouds': url = "https://images.unsplash.com/photo-1446483050676-bd2fdf3ac2d6?dpr=1&auto=format&crop=entropy&fit=crop&w=1080&h=720&q=80"; break; case 'light rain': url = "https://images.unsplash.com/photo-1462040700793-fcd2dbc0edf0?dpr=1&auto=format&crop=entropy&fit=crop&w=1500&h=1000&q=80"; break; } $("body").css('background-image', 'url(' + url +')');
}
function setWeatherInfo(weather) { $("#city").html(weather.name + ", " + weather.sys.country); var description = firstToUpper(weather.weather[0].description); $("#conditions").html(description); console.log(weather); $("#hum").html(weather.main.humidity + "%"); $("#cloudiness").html(weather.clouds.all + "%"); var temp = Math.round(weather.main.temp - 273.15); if($("#selector").hasClass("switched")) { temperature = toFahrenheit(temp) $("#temp").html(temperature + "°F"); } else { temperature = temp; $("#temp").html(temperature + "°C"); } changeBackground(weather.weather[0].description);
}
function getWeatherInfo(lat, lon) { var url = apiHTML + 'weather?lat=' + lat + '&lon=' + lon + '&APPID=' + weatherMemberKey; $.getJSON(url, function(json) { setWeatherInfo(json); });
}
$(document).ready(function() { $("#display").hide(); if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function(position) { var lat = position.coords.latitude; var lon = position.coords.longitude; getWeatherInfo(lat, lon); $("#info").fadeOut(1000); setTimeout(function() { $("#display").slideDown(1000); }, 1000); }); } toggleUnit();
});
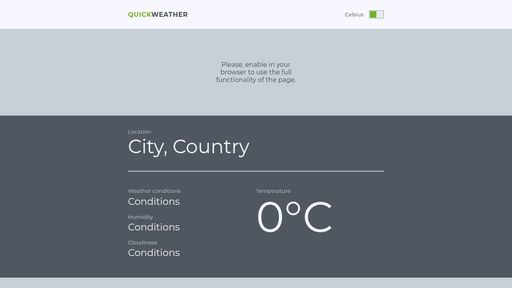
Developer | Tomasz |
Username | tomaszgil |
Uploaded | August 10, 2022 |
Rating | 3 |
Size | 4,600 Kb |
Views | 38,456 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Twitch App | 4,824 Kb |
JavaScript Calculator | 3,518 Kb |
A Pen by Tomasz | 1,884 Kb |
Simon Game | 5,103 Kb |
Random Quote Generator | 3,694 Kb |
TicTacToe Game | 4,158 Kb |
Wiki Search Box | 4,020 Kb |
Personal Portfolio | 5,159 Kb |
Pomodoro Clock | 4,180 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
WRENCH - STAFF | Lolita-adams | 1,608 Kb |
Objects | Bonzaipenguin | 2,616 Kb |
Page Transitions in Backbone | Mikefowler | 3,691 Kb |
Simple Carousel Pure CSS | Dangvanthanh | 4,080 Kb |
Bubble animation | Ftabor | 6,565 Kb |
Boxes | H3l1um | 2,563 Kb |
Lightning | Akm2 | 19,150 Kb |
Blog demo to use given styling | Andygirl | 2,412 Kb |
Shop Talk logo made in CSS | Hugo | 19,368 Kb |
Spinners using Font Icons | Keyamoon | 3,007 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!