Simon Game
How do I make an simon game?
Last freeCodeCamp assignment. User stories: 1. I am presented with a random series of button presses. 2. Each time I input a series of button presses correctly, I see the same series of button presses but with an additional step.. What is a simon game? How do you make a simon game? This script and codes were developed by Tomasz on 10 August 2022, Wednesday.
Simon Game - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Simon Game</title> <link href='https://fonts.googleapis.com/css?family=Montserrat:300,400,700' rel='stylesheet' type='text/css'> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="wrapper"> <h1 class="title">Simon Game</h1> <button id="start" class="btn">Start</button> <div class="game"> <p class="counter">Current score: <span id="count">0</span></p> <p class="alert" id="alert"></p> <div class="control-pannel"> <button id="btn-0" class="control"></button> <button id="btn-1" class="control"></button> <button id="btn-2" class="control"></button> <button id="btn-3" class="control"></button> </div> <div class="footer-pannel"> <div class="toggle-mode"> <div id="button-mode"> <div id="selector-switch"></div> </div> <p id="mode-name">Regular mode</p> </div> <button id="restart" class="btn">Restart</button> </div> </div>
</div> <script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.0/jquery.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Simon Game - Script Codes CSS Codes
* { font-family: "Montserrat", sans-serif; margin: 0; padding: 0; color: #f1f7ed;
}
body { background-color: #107eba;
}
.wrapper { padding: 1em 3em; width: 36em; height: 24em; margin-top: 10vh; margin-right: auto; margin-left: auto; border-radius: 1em; box-shadow: 0 0.3rem 0.75em #12355b; background-color: #12355b;
}
.title { color: #f1f7ed; text-align: center; font-size: 26pt; font-weight: 400; margin-top: 1em;
}
.btn { background-color: #107eba; height: 3em; width: 8em; border-radius: 1.5em; border: none;
}
.btn:hover { -webkit-filter: brightness(125%); filter: brightness(125%);
}
.btn#start { margin: 5em 14em;
}
.game { height: 16em; display: -webkit-box; display: -ms-flexbox; display: flex; -webkit-box-orient: vertical; -webkit-box-direction: normal; -ms-flex-direction: column; flex-direction: column; -webkit-box-pack: justify; -ms-flex-pack: justify; justify-content: space-between;
}
.counter { font-size: 14pt; text-align: center;
}
.counter #count { font-weight: 700;
}
.alert { font-size: 10pt; font-weight: 400; text-align: center; height: 10pt;
}
.control-pannel { display: -webkit-box; display: -ms-flexbox; display: flex; -ms-flex-pack: distribute; justify-content: space-around;
}
.control { height: 5em; width: 5em; border-radius: 2.5em; border: none;
}
.control:hover { -webkit-filter: brightness(150%); filter: brightness(150%);
}
.control:nth-child(1) { background-color: #107eba;
}
.control:nth-child(2) { background-color: #2add33;
}
.control:nth-child(3) { background-color: #ef8a17;
}
.control:nth-child(4) { background-color: #ef2917;
}
.control-active { -webkit-filter: brightness(150%); filter: brightness(150%);
}
#mode-name { text-weight: 300; display: inline-block;
}
.footer-pannel { display: -webkit-box; display: -ms-flexbox; display: flex; -webkit-box-align: center; -ms-flex-align: center; align-items: center; -webkit-box-pack: justify; -ms-flex-pack: justify; justify-content: space-between;
}
.toggle-mode { display: -webkit-box; display: -ms-flexbox; display: flex;
}
#button-mode { width: 2.5em; height: 1.3em; border-radius: 1.2em; background-color: #f1f7ed; cursor: pointer; display: inline-block; margin-right: 0.5em;
}
#selector-switch { position: relative; top: 0.125em; width: 1em; height: 1em; margin: 0 0.2em; border-radius: 50%; background-color: #107eba;
}
Simon Game - Script Codes JS Codes
$(".game").hide();
$(document).ready(main);
function handleStartButton() { $("#start").click(function() { $(this).fadeOut(300, function() { $(".game").fadeIn(300); var samplesLinks = ["https://s3.amazonaws.com/freecodecamp/simonSound1.mp3", "https://s3.amazonaws.com/freecodecamp/simonSound2.mp3", "https://s3.amazonaws.com/freecodecamp/simonSound3.mp3", "https://s3.amazonaws.com/freecodecamp/simonSound4.mp3"]; var game = new SimonGame(samplesLinks); game.playRound(); handleModeSwitching(game); handleGameRestarting(game); }); });
}
function handleModeSwitching(game) { $("#button-mode").on('click', function() { $("#selector-switch").toggleClass("switched"); if($("#selector-switch").hasClass("switched")) { $("#mode-name").html("Strict mode"); $("#selector-switch").animate({right: '-=1.1em'}, 300); game.setMode("strict"); } else { $("#mode-name").html("Regular mode"); $("#selector-switch").animate({right: '+=1.1em'}, 300); game.setMode("regular"); } });
}
function handleGameRestarting(game) { $("#restart").on('click', function() { game.restart("New Game!"); });
}
function main() { handleStartButton();
}
function NumberGenerator(start, end) { var min = start; var max = end; var sequence = []; this.clearSequence = function() { sequence = []; }; this.appendToSequence = function() { var number = Math.floor(Math.random() * (max - min) + min); sequence.push(number); console.log("sequence: ", sequence); }; this.getSequence = function() { return sequence; };
};
function Speaker(samples) { var sounds = []; for(var i=0; i<samples.length; i++) { sounds.push(new Audio(samples[i])); } this.play = function(i) { if(i < sounds.length) { sounds[i].play(); } }
}
function SimonGame(samplesLinks) { var score = 0; var mode = 'regular'; var userSequence = []; var sequenceGenerator = new NumberGenerator(0, samplesLinks.length); var speaker = new Speaker(samplesLinks); var playSequence = function(callback) { var soundNumbers = sequenceGenerator.getSequence(); var soundCount = 0; var interval; (function() { var fn = function() { $(".control").removeClass("control-active"); if(soundCount === score + 1) { clearInterval(interval); callback(); } else { speaker.play(soundNumbers[soundCount]); var str = "#btn-" + soundNumbers[soundCount]; $(str).addClass("control-active"); soundCount++; } }; interval = setInterval(fn, 1000); })(); }; var registerClicks = function() { userSequence = []; console.log("registering clicks", userSequence); $(".control").off('click').on('click', function() { var id = $(this).attr('id'); var idNumber = parseInt(id.slice(-1)); userSequence.push(idNumber); console.log("clicked", userSequence); speaker.play(idNumber); if(userSequence.length === score + 1) { checkUsersAnswer(); } }); }; this.playRound = function() { sequenceGenerator.appendToSequence(); playSequence(registerClicks); }; var checkUsersAnswer = function() { console.log("checking...", mode); console.log("user: ", userSequence); console.log("game: ", sequenceGenerator.getSequence()); var generatedSequence = sequenceGenerator.getSequence(); var areEqual = true; var alert = ""; for(var i=0; i<userSequence.length; i++) { if(userSequence[i] !== generatedSequence[i]) { areEqual = false; break; } } if(areEqual) { score++; console.log("correct!"); if(score === 20) { alert = "Congratulations! You win."; score = 0; sequenceGenerator.clearSequence(); sequenceGenerator.appendToSequence(); playSequence(registerClicks); } else { sequenceGenerator.appendToSequence(); playSequence(registerClicks); } } else { if(mode === "regular") { alert = "Wrong! Try again..."; playSequence(registerClicks); } else { alert = "Wrong! Game over."; score = 0; sequenceGenerator.clearSequence(); sequenceGenerator.appendToSequence(); playSequence(registerClicks); } } $("#alert").text(alert); $("#count").text(score); }; this.restart = function(alert) { score = 0; sequenceGenerator.clearSequence(); sequenceGenerator.appendToSequence(); playSequence(registerClicks); $("#count").text(score); $("#alert").text(alert); }; this.setMode = function(newMode) { console.log("Setting mode: ", newMode); mode = newMode; };
};
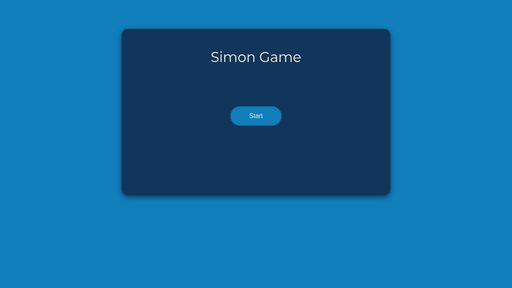
Developer | Tomasz |
Username | tomaszgil |
Uploaded | August 10, 2022 |
Rating | 3 |
Size | 5,103 Kb |
Views | 40,480 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Wiki Search Box | 4,020 Kb |
Twitch App | 4,824 Kb |
Random Quote Generator | 3,694 Kb |
Personal Portfolio | 5,159 Kb |
JavaScript Calculator | 3,518 Kb |
TicTacToe Game | 4,158 Kb |
Quick Weather App | 4,600 Kb |
Pomodoro Clock | 4,180 Kb |
A Pen by Tomasz | 1,884 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Flower expansion | Sreucherand | 3,425 Kb |
Svg animation draw | SzymonDziewonski | 5,545 Kb |
Responsive Advert | James_zedd | 2,354 Kb |
Vue Transition | Chenming142 | 4,561 Kb |
Resizable SASS Icons | Marianarlt | 7,611 Kb |
CSS Link Icons with jQuery Titles | Nicwinn | 2,312 Kb |
Mini Profile | Frytyler | 3,828 Kb |
Nice responsive team page | Infomiho | 3,139 Kb |
CSS Social Media Icon | TychoBlender | 3,871 Kb |
-2 base | Kozanecki_p | 1,641 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!