React Carousel POC
How do I make an react carousel poc?
What is a react carousel poc? How do you make a react carousel poc? This script and codes were developed by Cory on 13 December 2022, Tuesday.
React Carousel POC - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>React Carousel POC</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <body> <style> html, body { box-sizing: border-box; } *, *::before, *::after { box-sizing: inherit; } figure { margin: 0; } .mySlide { width: 100%; margin: 0; display: flex; flex-flow: column; justify-content: space-between; } .mySlide img { width: 100%; } .mySlide figcaption { font-family: "Helvetica", sans-serif; font-weight: 100; text-align: center; padding: 1em; } .track { border: 1em solid transparent; flex: 1 1 100%; } .slider { display: flex; flex-flow: row nowrap; align-items: stretch; justify-content: space-between; width: 100vw; } .slider button { border: none; background: hsla(0, 0%, 0%, .05); padding: .5em; font-size: 2em; } </style> <main> </main> </body>
</html> <script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.3.1/react.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.3.1/react-dom.js'></script> <script src="js/index.js"></script>
</body>
</html>
React Carousel POC - Script Codes CSS Codes
html, body { box-sizing: border-box;
}
*,
*::before,
*::after { box-sizing: inherit;
}
figure { margin: 0;
}
.carousel { background-color: rgb(102, 51, 153); display: -webkit-box; display: -ms-flexbox; display: flex; padding: 1em; box-sizing: border-box; position: relative;
}
.carousel * { box-sizing: inherit;
}
.track { display: -webkit-box; display: -ms-flexbox; display: flex; -ms-flex-flow: row nowrap; flex-flow: row nowrap; -webkit-box-pack: justify; -ms-flex-pack: justify; justify-content: space-between; overflow-x: auto; position: relative; /* makes .track an offset parent */
}
.my-slide img { width: 100%; background: #bada55; /**/ padding: 1em;
}
.control-left,
.control-right { background-color: hsla(0, 100%, 100%, .2); border: none; color: white; cursor: pointer; font-size: 10vh; outline: none;
}
.control-left:hover, .control-right:hover { background-color: hsla(0, 100%, 100%, .4);
}
React Carousel POC - Script Codes JS Codes
'use strict';
var _class, _temp, _class2, _temp2;
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
var _React = React;
var Component = _React.Component;
var Children = _React.Children;
var PropTypes = _React.PropTypes;
var _ReactDOM = ReactDOM;
var findDOMNode = _ReactDOM.findDOMNode;
var render = _ReactDOM.render;
var array = PropTypes.array;
var bool = PropTypes.bool;
var func = PropTypes.func;
var number = PropTypes.number;
var object = PropTypes.object;
var node = PropTypes.node;
var oneOf = PropTypes.oneOf;
var oneOfType = PropTypes.oneOfType;
var string = PropTypes.string;
var Slide = function Slide(_ref) { var _ref$basis = _ref.basis; var basis = _ref$basis === undefined ? '100%' : _ref$basis; var _ref$gutter = _ref.gutter; var gutter = _ref$gutter === undefined ? '1em' : _ref$gutter; var _ref$className = _ref.className; var className = _ref$className === undefined ? '' : _ref$className; var children = _ref.children; var props = _objectWithoutProperties(_ref, ['basis', 'gutter', 'className', 'children']); return React.createElement( 'div', _extends({ className: className, style: { flex: '0 0 auto', width: basis, marginLeft: gutter } }, props), children );
};
Slide.propTypes = { basis: string, gutter: string, children: node, className: oneOf([string, array, object])
};
var easingFn = function easingFn(t) { return --t * Math.pow(t, 2) + 1;
};
var values = Object.values || function (obj) { return Object.keys(obj).map(function (key) { return obj[key]; });
};
var compose = function compose() { for (var _len = arguments.length, fns = Array(_len), _key = 0; _key < _len; _key++) { fns[_key] = arguments[_key]; } return function (val) { return fns.reduceRight(function (currVal, fn) { return fn(currVal); }, val); };
};
var minMap = function minMap() { for (var _len2 = arguments.length, vals = Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { vals[_key2] = arguments[_key2]; } return function (val) { var _Math; return (_Math = Math).min.apply(_Math, vals.concat([val])); };
};
var maxMap = function maxMap() { for (var _len3 = arguments.length, vals = Array(_len3), _key3 = 0; _key3 < _len3; _key3++) { vals[_key3] = arguments[_key3]; } return function (val) { var _Math2; return (_Math2 = Math).max.apply(_Math2, vals.concat([val])); };
};
var on = function on(evt) { var opts = arguments.length <= 1 || arguments[1] === undefined ? false : arguments[1]; return function (cb) { return function (el) { return el.addEventListener(evt, cb, opts); }; };
};
var onWindowScroll = function onWindowScroll(cb) { return on('scroll', true)(cb)(window);
};
var onScroll = function onScroll(cb) { var _ref2 = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1]; var _ref2$target = _ref2.target; var target = _ref2$target === undefined ? window : _ref2$target; return onWindowScroll(function (e) { return (target === window || target === e.target) && cb(e); });
};
var onScrollEnd = function onScrollEnd(cb) { var _ref3 = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1]; var _ref3$wait = _ref3.wait; var wait = _ref3$wait === undefined ? 200 : _ref3$wait; var _ref3$target = _ref3.target; var target = _ref3$target === undefined ? window : _ref3$target; return function (timeoutID) { return onScroll(function (evt) { clearTimeout(timeoutID); timeoutID = setTimeout(function () { return evt.target === target ? cb() : undefined; }, wait); }); }(0);
};
var onScrollStart = function onScrollStart(cb) { var _ref4 = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1]; var _ref4$target = _ref4.target; var target = _ref4$target === undefined ? window : _ref4$target; var started = false; onScrollEnd(function () { return started = false; }, { target: target }); onScroll(function (e) { if (!started) { started = true; cb(e); } }, { target: target });
};
var trackTouchesForElement = function trackTouchesForElement(el) { var touchIds = []; on('touchstart')(function (_ref5) { var changedTouches = _ref5.changedTouches; var changedIds = [].slice.call(changedTouches).map(function (_ref6) { var identifier = _ref6.identifier; return identifier; }); touchIds = [].concat(touchIds, changedIds); })(el); on('touchend')(function (_ref7) { var changedTouches = _ref7.changedTouches; var changedIds = [].slice.call(changedTouches).map(function (_ref8) { var identifier = _ref8.identifier; return identifier; }); touchIds = touchIds.filter(function (touchId) { return !changedIds.includes(touchId); }); })(el); return function () { return touchIds.length; };
};
var animate = function animate(el) { var _ref9 = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1]; var _ref9$delta = _ref9.delta; var delta = _ref9$delta === undefined ? 0 : _ref9$delta; var _ref9$immediate = _ref9.immediate; var immediate = _ref9$immediate === undefined ? false : _ref9$immediate; var _ref9$duration = _ref9.duration; var duration = _ref9$duration === undefined ? immediate ? 0 : 500 : _ref9$duration; var _ref9$easing = _ref9.easing; var easing = _ref9$easing === undefined ? easingFn : _ref9$easing; var _ref9$prop = _ref9.prop; var prop = _ref9$prop === undefined ? 'scrollTop' : _ref9$prop; return new Promise(function (res, rej) { var initialVal = el[prop]; var overFlowStyle = el.style.overflow || 'auto'; var startTime = null; var step = function step(timestamp) { if (!startTime) startTime = timestamp; var progressTime = timestamp - startTime; var progressRatio = easing(progressTime / duration); el[prop] = initialVal + delta * progressRatio; if (progressTime < duration) { window.requestAnimationFrame(step); } else { el[prop] = initialVal + delta; // paranoia check. jump to the end when animation time is complete. // Give scroll control back to the user once animation is done. // el.style.overflow = overFlowStyle // MS Edge doesn't like the above apparently. el.setAttribute('style', el.getAttribute('style').replace(/(overflow:\s?)\w*/, '$1' + overFlowStyle)); res(); } }; // We are going to temporarily prevent the user from being able to scroll during the animation. // This will prevent a janky fight between user scroll and animation which is just bad user experience. el.style.overflow = 'hidden'; window.requestAnimationFrame(step); });
};
var normalizeIndex = function normalizeIndex(idx, len) { return (idx % len + len) % len;
};
var Track = (_temp = _class = function (_Component) { _inherits(Track, _Component); function Track(props) { _classCallCheck(this, Track); // We can't do arrow function properties for these since // we are passing them to the consuming component and we // require the proper context var _this = _possibleConstructorReturn(this, _Component.call(this, props)); _this.state = { activeIndex: 0 }; _this.handleKeyUp = function (nextKeys, prevKeys) { return function (_ref10) { var key = _ref10.key; var isNext = nextKeys.includes(key); var isPrev = prevKeys.includes(key); if (isNext) _this.next(); if (isPrev) _this.prev(); }; }(['ArrowRight'], ['ArrowLeft']); _this.getNearestSlideIndex = function () { var _Math3; var _this$track = _this.track; var children = _this$track.children; var scrollLeft = _this$track.scrollLeft; var offsets = [].slice.call(children).map(function (_ref11) { var offsetLeft = _ref11.offsetLeft; return Math.abs(offsetLeft - scrollLeft); }); return offsets.indexOf((_Math3 = Math).min.apply(_Math3, offsets)); }; _this.reorderLastVisibleSet = function (_ref12) { var _slice; var _ref12$reset = _ref12.reset; var reset = _ref12$reset === undefined ? true : _ref12$reset; var _ref12$prev = _ref12.prev; var prev = _ref12$prev === undefined ? false : _ref12$prev; var _ref12$next = _ref12.next; var next = _ref12$next === undefined ? false : _ref12$next; var visibleSlides = _this.props.visibleSlides; var resetOrder = function resetOrder(el) { el.style.order = 'initial'; }; var orderForPrev = function orderForPrev(el) { el.style.order = 1; }; var orderForNext = function orderForNext(el) { el.style.order = -1; }; (_slice = [].slice).call.apply(_slice, [_this.track.children].concat(prev ? [0, visibleSlides] : next ? [-visibleSlides] : [])).forEach(prev ? orderForPrev : next ? orderForNext : resetOrder); }; _this.setRef = function (name) { return function (ref) { _this[name] = ref; }; }; _this.next = _this.next.bind(_this); _this.prev = _this.prev.bind(_this); _this.infiniteNext = _this.infiniteNext.bind(_this); _this.infinitePrev = _this.infinitePrev.bind(_this); _this.slideTo = _this.slideTo.bind(_this); return _this; } Track.prototype.componentDidMount = function componentDidMount() { var _this2 = this; this.DOMNode = findDOMNode(this.track); // These are not a part of component state since we don't want // incure the overhead of calling setState. They are either cached // values or state only the onScrollEnd callback cares about and // are not important to the rendering of the component. this.childCount = this.track.children.length; var isAnimating = false; var isScrolling = false; var getOngoingTouchCount = trackTouchesForElement(this.DOMNode); var shouldSelfCorrect = function shouldSelfCorrect() { return !_this2.props.preventSnapping && !isAnimating && !isScrolling && !getOngoingTouchCount(); }; onScrollStart(function () { isScrolling = true; }); onScrollEnd(function () { isScrolling = false; isAnimating = false; if (shouldSelfCorrect()) { isAnimating = true; _this2.slideTo(_this2.getNearestSlideIndex()); } }, { target: this.DOMNode }); on('touchend')(function () { if (shouldSelfCorrect()) { isAnimating = true; _this2.slideTo(_this2.getNearestSlideIndex()); } })(this.track); this.slideTo(this.props.startAt, { immediate: true }); }; Track.prototype.componentDidUpdate = function componentDidUpdate() { this.childCount = this.track.children.length; }; // We don't want to update if only state changed. // Sate is not important to the rendering of this component Track.prototype.shouldComponentUpdate = function shouldComponentUpdate(nextProps) { var propValues = values(this.props); var nextPropValues = values(nextProps); return !nextPropValues.every(function (val, i) { return val === propValues[i]; }); }; Track.prototype.next = function next() { return this.slideTo(this.state.activeIndex + this.props.visibleSlides); }; Track.prototype.infiniteNext = function infiniteNext() { var _this3 = this; if (this.state.activeIndex >= this.childCount - this.props.visibleSlides) { this.reorderLastVisibleSet({ next: true }); this.track.scrollLeft = 0; return this.next().then(function () { _this3.reorderLastVisibleSet({ reset: true }); _this3.track.scrollLeft = 0; }); } else { return this.next(); } }; Track.prototype.prev = function prev() { return this.slideTo(this.state.activeIndex - this.props.visibleSlides); }; Track.prototype.infinitePrev = function infinitePrev() { var _this4 = this; if (this.state.activeIndex < this.props.visibleSlides) { this.reorderLastVisibleSet({ prev: true }); this.track.scrollLeft = this.track.scrollWidth; return this.prev().then(function () { _this4.reorderLastVisibleSet({ reset: true }); _this4.track.scrollLeft = _this4.track.scrollWidth; }); } else { return this.prev(); } }; Track.prototype.slideTo = function slideTo(index) { var _this5 = this; var _ref13 = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1]; var _ref13$immediate = _ref13.immediate; var immediate = _ref13$immediate === undefined ? false : _ref13$immediate; var afterSlide = this.props.afterSlide; var _track = this.track; var children = _track.children; var scrollLeft = _track.scrollLeft; var slideIndex = normalizeIndex(index, this.childCount); var delta = children[slideIndex].offsetLeft - scrollLeft; return animate(this.track, { prop: 'scrollLeft', delta: delta, immediate: immediate }).then(function () { if (_this5.state.activeIndex !== slideIndex) { _this5.setState({ activeIndex: slideIndex }); afterSlide(slideIndex); } }); }; Track.prototype.render = function render() { var _props = this.props; var children = _props.children; var className = _props.className; var gutter = _props.gutter; var preventScroll = _props.preventScroll; var slideClass = _props.slideClass; var visibleSlides = _props.visibleSlides; var preventScrolling = preventScroll ? 'hidden' : 'auto'; var styles = { display: 'flex', flexFlow: 'row nowrap', justifyContent: 'space-between', overflowX: preventScrolling, msOverflowStyle: '-ms-autohiding-scrollbar', /* chrome like scrollbar experience for IE/Edge */ position: 'relative', /* makes .track an offset parent */ transition: 'all .25s ease-in-quint', outline: 'none' }; return React.createElement( 'div', { className: className, style: styles, ref: this.setRef('track'), tabIndex: '0', onKeyUp: this.handleKeyUp }, Children.map(children.apply(undefined, this.props.infinite ? [this.infiniteNext, this.infinitePrev] : [this.next, this.prev]), function (child, i) { return React.createElement( Slide, { className: slideClass, key: 'slide-' + i, basis: 'calc((100% - (' + gutter + ' * ' + (visibleSlides - 1) + ')) / ' + visibleSlides + ')', gutter: gutter, onClick: child.props.onClick }, child ); }) ); }; return Track;
}(Component), _class.propTypes = { afterSlide: func, children: func, className: oneOfType([array, string, object]), gutter: string, infinite: bool, preventScroll: bool, slideClass: oneOfType([array, string, object]), preventSnapping: bool, startAt: number, visibleSlides: number
}, _class.defaultProps = { afterSlide: function afterSlide() {}, gutter: '1em', infinite: false, preventScroll: false, startAt: 0, visibleSlides: 1
}, _temp);
var Track2 = (_temp2 = _class2 = function (_Component2) { _inherits(Track2, _Component2); function Track2(props) { _classCallCheck(this, Track2); var _this6 = _possibleConstructorReturn(this, _Component2.call(this, props)); _this6.handleKeyUp = function (nextKeys, prevKeys) { return function (_ref14) { var key = _ref14.key; var isNext = nextKeys.includes(key); var isPrev = prevKeys.includes(key); if (isNext) _this6.next(); if (isPrev) _this6.prev(); }; }(['ArrowRight'], ['ArrowLeft']); _this6.getNearestSlideIndex = function () { var _Math4; var _this6$track = _this6.track; var children = _this6$track.children; var scrollLeft = _this6$track.scrollLeft; var offsets = [].slice.call(children).map(function (_ref15) { var offsetLeft = _ref15.offsetLeft; return Math.abs(offsetLeft - scrollLeft); }); return offsets.indexOf((_Math4 = Math).min.apply(_Math4, offsets)); }; _this6.setRef = function (name) { return function (ref) { _this6[name] = ref; }; }; _this6.state = { activeIndex: 0 }; // We can't do arrow function properties for these since // we are passing them to the consuming component and we // require the proper context _this6.next = _this6.next.bind(_this6); _this6.prev = _this6.prev.bind(_this6); _this6.slideTo = _this6.slideTo.bind(_this6); return _this6; } Track2.prototype.componentDidMount = function componentDidMount() { var _this7 = this; this.DOMNode = findDOMNode(this.track); // These are not a part of component state since we don't want // incure the overhead of calling setState. They are either cached // values or state only the onScrollEnd callback cares about and // are not important to the rendering of the component. this.childCount = this.track.children.length; var isAnimating = false; var isScrolling = false; var getOngoingTouchCount = trackTouchesForElement(this.DOMNode); var shouldSelfCorrect = function shouldSelfCorrect() { return !_this7.props.preventSnapping && !isAnimating && !isScrolling && !getOngoingTouchCount(); }; onScrollStart(function () { isScrolling = true; }); onScrollEnd(function () { isScrolling = false; isAnimating = false; if (shouldSelfCorrect()) { isAnimating = true; _this7.slideTo(_this7.getNearestSlideIndex()); } }, { target: this.DOMNode }); on('touchend')(function () { if (shouldSelfCorrect()) { isAnimating = true; _this7.slideTo(_this7.getNearestSlideIndex()); } })(this.track); this.slideTo(this.props.startAt, { immediate: true }); }; Track2.prototype.componentDidUpdate = function componentDidUpdate() { this.childCount = this.track.children.length; }; // We don't want to update if only state changed. // Sate is not important to the rendering of this component Track2.prototype.shouldComponentUpdate = function shouldComponentUpdate(nextProps) { var propValues = values(this.props); var nextPropValues = values(nextProps); return !nextPropValues.every(function (val, i) { return val === propValues[i]; }); }; Track2.prototype.next = function next() { var childCount = this.childCount; var props = this.props; var state = this.state; var activeIndex = state.activeIndex; var visibleSlides = props.visibleSlides; var firstIndex = 0; var lastIndex = childCount - visibleSlides; var nextActive = activeIndex + visibleSlides; return this.slideTo(activeIndex === lastIndex ? firstIndex : nextActive < lastIndex ? nextActive : lastIndex); }; Track2.prototype.prev = function prev() { var activeIndex = this.state.activeIndex; var visibleSlides = this.props.visibleSlides; var firstIndex = 0; var nextActive = activeIndex - visibleSlides; return this.slideTo(activeIndex === firstIndex || nextActive > firstIndex ? nextActive : firstIndex); }; Track2.prototype.slideTo = function slideTo(index) { var _this8 = this; var _ref16 = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1]; var _ref16$immediate = _ref16.immediate; var immediate = _ref16$immediate === undefined ? false : _ref16$immediate; var afterSlide = this.props.afterSlide; var _track2 = this.track; var children = _track2.children; var scrollLeft = _track2.scrollLeft; var slideIndex = normalizeIndex(index, this.childCount); var delta = children[slideIndex].offsetLeft - scrollLeft; return animate(this.track, { prop: 'scrollLeft', delta: delta, immediate: immediate }).then(function () { if (_this8.state.activeIndex !== slideIndex) { _this8.setState({ activeIndex: slideIndex }); afterSlide(slideIndex); } }); }; Track2.prototype.render = function render() { var _props2 = this.props; var children = _props2.children; var className = _props2.className; var gutter = _props2.gutter; var preventScroll = _props2.preventScroll; var slideClass = _props2.slideClass; var visibleSlides = _props2.visibleSlides; var preventScrolling = preventScroll ? 'hidden' : 'auto'; var styles = { display: 'flex', flexFlow: 'row nowrap', justifyContent: 'space-between', overflowX: preventScrolling, msOverflowStyle: '-ms-autohiding-scrollbar', /* chrome like scrollbar experience for IE/Edge */ position: 'relative', /* makes .track an offset parent */ transition: 'all .25s ease-in-quint', outline: 'none' }; return React.createElement( 'div', { className: className, style: styles, ref: this.setRef('track'), tabIndex: '0', onKeyUp: this.handleKeyUp }, Children.map(children(this.next, this.prev), function (child, i) { return React.createElement( Slide, { className: slideClass, key: 'slide-' + i, basis: 'calc((100% - (' + gutter + ' * ' + (visibleSlides - 1) + ')) / ' + visibleSlides + ')', gutter: gutter, onClick: child.props.onClick }, child ); }) ); }; return Track2;
}(Component), _class2.propTypes = { afterSlide: func, children: func, className: oneOfType([array, string, object]), gutter: string, preventScroll: bool, slideClass: oneOfType([array, string, object]), preventSnapping: bool, startAt: number, visibleSlides: number
}, _class2.defaultProps = { afterSlide: function afterSlide() {}, gutter: '1em', preventScroll: false, startAt: 0, visibleSlides: 1
}, _temp2);
var Slider = function Slider(_ref17) { var ux = _ref17.ux; var children = _ref17.children; var infinite = _ref17.infinite; var onNext = undefined; var onPrev = undefined; var next = function next() { return onNext(); }; var prev = function prev() { return onPrev(); }; return React.createElement( 'div', { className: 'slider' }, React.createElement( 'button', { onClick: prev }, '<' ), ux ? React.createElement( Track2, { visibleSlides: 3, className: 'track', infinite: infinite }, function (_next, _prev) { onNext = _next; onPrev = _prev; return children; } ) : React.createElement( Track, { visibleSlides: 3, className: 'track', infinite: infinite }, function (_next, _prev) { onNext = _next; onPrev = _prev; return children; } ), React.createElement( 'button', { onClick: next }, '>' ) );
};
Slider.propTypes = { children: array
};
render(React.createElement( 'div', null, React.createElement( 'h1', null, 'Carousel appears to go on infinitely in both directions' ), React.createElement( Slider, { infinite: true }, React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?1' }), React.createElement( 'figcaption', null, 'Beard Index 0' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?2' }), React.createElement( 'figcaption', null, 'Beard Index 1' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?3' }), React.createElement( 'figcaption', null, 'Beard Index 2' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?4' }), React.createElement( 'figcaption', null, 'Beard Index 3' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?6' }), React.createElement( 'figcaption', null, 'Beard Index 4' ) ) ), React.createElement('hr', null), React.createElement( 'h1', null, 'Carousel controls always work, but user is visually returned to the beginning when the end is reached' ), React.createElement( Slider, { ux: true }, React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?1' }), React.createElement( 'figcaption', null, 'Beard Index 0' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?2' }), React.createElement( 'figcaption', null, 'Beard Index 1' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?3' }), React.createElement( 'figcaption', null, 'Beard Index 2' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?4' }), React.createElement( 'figcaption', null, 'Beard Index 3' ) ), React.createElement( 'figure', { className: 'mySlide' }, React.createElement('img', { alt: 'Place Beard', src: 'http://placebeard.it/300/300?5' }), React.createElement( 'figcaption', null, 'Beard Index 4' ) ) )
), document.querySelector('main'));
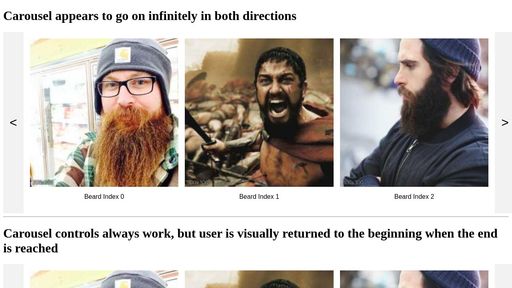
Developer | Cory |
Username | uniqname |
Uploaded | December 13, 2022 |
Rating | 3 |
Size | 12,622 Kb |
Views | 34,408 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Randomly shuffle colors | 2,806 Kb |
Audio Player | 7,940 Kb |
Memory Leak Demo | 2,074 Kb |
Javascript Digital Clock Example | 1,797 Kb |
A Pen by Cory | 3,306 Kb |
CSA-React POC | 5,660 Kb |
PictureTime | 3,565 Kb |
Floating Labels | 2,238 Kb |
JS REPL | 5,216 Kb |
Paper like dialog compoent | 4,701 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Layout 11 | Altynai | 1,690 Kb |
Part 19 Bootstrap split button dropdown | Venkatesha | 1,601 Kb |
Social.svg.min | Larsenwork | 13,849 Kb |
Review test | Otro_user_gil | 4,054 Kb |
Another brick in the wall | Fivera | 1,955 Kb |
IbrahimJabbari-Effect14 | Ibrahimjabbari | 1,919 Kb |
Css Rotating 3d cubes different speed | Dghez | 2,364 Kb |
Prism | Pyrografix | 2,843 Kb |
A Pen by Matt Popovich | Mpopv | 3,349 Kb |
CSS Tooltips | Darylldoyle | 2,599 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!