React TransitionGroup - Fancy
How do I make an react transitiongroup - fancy?
A visually fancier version of my ReactTransitionGroup exercise. What is a react transitiongroup - fancy? How do you make a react transitiongroup - fancy? This script and codes were developed by Pedro Tavares on 01 December 2022, Thursday.
React TransitionGroup - Fancy - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>React TransitionGroup - Fancy</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div id="root"></div> <script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.3.1/react-with-addons.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/react/15.3.1/react-dom.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/gsap/1.19.0/TweenMax.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
React TransitionGroup - Fancy - Script Codes CSS Codes
html, body { width: 100%; height: 100%;
}
div, span { box-sizing: border-box;
}
div { padding: 0; margin: 0; text-align: center; position: relative; display:inline-block;
}
span { display: inline-block; position: relative; left:0; top:0; margin:0; padding:0; font-size: 10vh; text-align: center; color: rgba(230,230,235,0.5); text-shadow: 0 0 2px rgba(255,255,255,0.5);
}
p { font-size: 0.8rem; line-height: 1.2rem;
}
#root { position: relative; width: 100%; height: 100%; background: -webkit-linear-gradient(top, #eeeeee 0%,#cccccc 100%); background: linear-gradient(to bottom, #eeeeee 0%,#cccccc 100%);
}
.holder { position: absolute; left: 50%; top: 45%; -webkit-transform: translate(-50%, -50%); transform: translate(-50%, -50%);
}
.clock { overflow: hidden; border-radius: 16px; border: 3px solid black; border-top-color: rgb(240,240,240); border-left-color: rgb(240,240,240); border-right-color: rgb(230,230,230); border-bottom-color: rgb(230,230,230); background: rgb(220,220,220); box-shadow: inset 0 0 18px rgba(50,50,50,0.7), 5px 5px 20px hsl(0, 0%, 50%);
}
.unitContainer { margin: 0; padding: 18px;
}
.unitHolder { border: 3px solid rgb(100,100,100); border-right-color: rgb(200,200,200); border-bottom-color: rgb(240,240,240); background: -webkit-linear-gradient(top, #4c4c4c 0%, #595959 12%, #666666 25%, #474747 39%, #2c2c2c 50%, #000000 51%, #111111 60%, #2b2b2b 76%, #1c1c1c 91%, #131313 100%); background: linear-gradient(to bottom, #4c4c4c 0%, #595959 12%, #666666 25%, #474747 39%, #2c2c2c 50%, #000000 51%, #111111 60%, #2b2b2b 76%, #1c1c1c 91%, #131313 100%); border-radius: 8px; position: relative; overflow: hidden;
}
.unit { width: 100%; height: 100%;
}
React TransitionGroup - Fancy - Script Codes JS Codes
"use strict";
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
// Import the TransitionGroup from React's add-ons
var TransitionGroup = React.addons.TransitionGroup;
// Create a React component to hold the a generic unit of time. Hour, minute, second.
// It is here that we will control the unit's animation
var Unit = function (_React$Component) { _inherits(Unit, _React$Component); function Unit() { _classCallCheck(this, Unit); return _possibleConstructorReturn(this, _React$Component.apply(this, arguments)); } // Let's create a single simple method to handle the animation Unit.prototype.animate = function animate(el, callback, out) { // Some constants to reduce repetition var dur = 0.5; var amount = 100; var ease = "Power1.easeInOut"; // Check if it is the in animation or the out animation if (out) { TweenMax.set(el, { position: "absolute" }); TweenMax.to(el, dur, { yPercent: -amount, ease: ease, clearProps: "all", onComplete: callback }); } else { TweenMax.from(this.el, dur, { yPercent: amount, ease: ease, clearProps: "all", onComplete: callback }); } }; // This method gets called every time the component is added to the DOM except on the first load Unit.prototype.componentWillEnter = function componentWillEnter(callback) { this.animate(this.el, callback); }; // This method gets called every time the component is about to be removed from the DOM Unit.prototype.componentWillLeave = function componentWillLeave(callback) { this.animate(this.el, callback, true); }; Unit.prototype.render = function render() { var _this2 = this; return React.createElement( "span", { className: "unit", ref: function ref(el) { _this2.el = el; } }, this.props.number ); }; return Unit;
}(React.Component);
// The clock component will be the one responsible for counting the time and tell each of the units of time what time they should display
var Clock = function (_React$Component2) { _inherits(Clock, _React$Component2); function Clock(props) { _classCallCheck(this, Clock); var _this3 = _possibleConstructorReturn(this, _React$Component2.call(this, props)); var date = new Date(); _this3.visChange = _this3.visChange.bind(_this3); _this3.state = { hidden: "", hours: ("0" + date.getHours()).slice(-2), minutes: ("0" + date.getMinutes()).slice(-2), seconds: ("0" + date.getSeconds()).slice(-2) }; return _this3; } Clock.prototype.visChange = function visChange(e) { if (document[this.state.hidden]) { this.stopCount(); } else { this.startCount(); } }; Clock.prototype.startCount = function startCount() { var _this4 = this; this.timerID = setInterval(function () { return _this4.tick(); }, 1000); }; Clock.prototype.stopCount = function stopCount() { clearInterval(this.timerID); }; Clock.prototype.componentDidMount = function componentDidMount() { // from: https://developer.mozilla.org/en-US/docs/Web/API/Page_Visibility_API // Set the name of the hidden property and the change event for visibility var visibilityChange = undefined; if (typeof document.hidden !== "undefined") { // Opera 12.10 and Firefox 18 and later support this.setState({ hidden: "hidden" }); visibilityChange = "visibilitychange"; } else if (typeof document.msHidden !== "undefined") { this.setState({ hidden: "msHidden" }); visibilityChange = "msvisibilitychange"; } else if (typeof document.webkitHidden !== "undefined") { this.setState({ hidden: "webkitHidden" }); visibilityChange = "webkitvisibilitychange"; } this.startCount(); document.addEventListener(visibilityChange, this.visChange); }; Clock.prototype.componentWillUnmount = function componentWillUnmount() { this.stopCount(); }; Clock.prototype.tick = function tick() { var date = new Date(); var hours = ("0" + date.getHours()).slice(-2); var minutes = ("0" + date.getMinutes()).slice(-2); var seconds = ("0" + date.getSeconds()).slice(-2); this.setState({ hours: hours, minutes: minutes, seconds: seconds }); }; Clock.prototype.render = function render() { // Here's the gotcha that took me a long while to understand. // It is not enough to simply update the 'number' props of each <Unit /> component. We need to create a new component at each render and use it to replace the old one. // We give each unit a unique key based on the time and add a string to differenciate between the siblings so that React can correctly identify which component is which. var hours = React.createElement(Unit, { key: "h" + this.state.hours, number: this.state.hours }); var mins = React.createElement(Unit, { key: "m" + this.state.minutes, number: this.state.minutes }); var secs = React.createElement(Unit, { key: "s" + this.state.seconds, number: this.state.seconds }); // React requires us to return one single root element. And because of the animation effect we are trying to achieve here, we have to encapsulate each of our <Unit /> components on a <TransitionGroup /> return React.createElement( "div", { className: "holder" }, React.createElement( "h1", null, "React Clock" ), React.createElement( "div", { className: "clock" }, React.createElement( "div", { className: "unitContainer" }, React.createElement( TransitionGroup, { component: "div", className: "unitHolder" }, hours ) ), React.createElement( "div", { className: "unitContainer" }, React.createElement( TransitionGroup, { component: "div", className: "unitHolder" }, mins ) ), React.createElement( "div", { className: "unitContainer" }, React.createElement( TransitionGroup, { component: "div", className: "unitHolder" }, secs ) ) ), React.createElement( "p", { style: { textDecoration: 'line-through' } }, "There is a tiny wobble when viewing in chrome. If you know why that happens, let me know. I haven't managed to squash that bug." ), React.createElement( "p", { style: { textDecoration: 'line-through' } }, "Erm... By just adding this text, the wobble in Chrome goes away. Go figure." ), React.createElement( "p", null, "Nope. Adding the extra line brought the wobble back but sideways. Now with this paragraph. Wobble is back to up and down." ), React.createElement( "p", null, "While in safari, the colour of the number changes when animating. One day, I'll investigate the reason why..." ) ); }; return Clock;
}(React.Component);
ReactDOM.render(React.createElement(Clock, null), document.getElementById('root'));
/* Other Notes: This would not work because of the ':' present inside the transition group <TransitionGroup> {hours}:{mins}:{secs} </TransitionGroup> However, this would <TransitionGroup> {hours}{mins}{secs} </TransitionGroup> This would also work <TransitionGroup> {hours}<span>:</span>{mins}<span>:</span>{secs} </TransitionGroup> This would not work because the encompassing <div> is not a React component and therefore does not communicates with the <TransitionGroup> <TransitionGroup> <div>{hours}<span>:</span>{mins}<span>:</span>{secs}</div> </TransitionGroup> Here, we are only updating values, we are not adding or removing the components, which would trigger the lifecycle hooks <TransitionGroup> <Unit key="hours" number={this.state.hours} /> <Unit key="minutes" number={this.state.minutes} /> <Unit key="seconds" number={this.state.seconds} /> </TransitionGroup>
*/
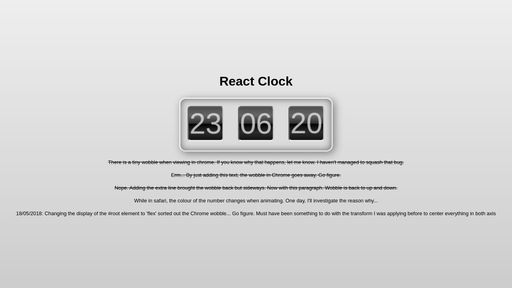
Developer | Pedro Tavares |
Username | dipscom |
Uploaded | December 01, 2022 |
Rating | 3 |
Size | 7,407 Kb |
Views | 10,120 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
MOMO BROS | 5,043 Kb |
SVG Grid Mask - With Color effect | 3,666 Kb |
Responsive Animated Moving Things | 8,646 Kb |
GSAP - Fun with Cycle and Bezier 2 | 4,195 Kb |
GSAP - Responsive Corner Navigation | 3,210 Kb |
Using Functions to Build a timeline - Broken | 1,831 Kb |
Shooting Hoops | 6,731 Kb |
Falling tomatoes | 2,772 Kb |
Animated SVG Blur | 2,288 Kb |
Mask Within Mask | 3,843 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
JavaScript Animation | Rcmeisty | 4,581 Kb |
CSS only simple parallax scroll | Stanssongs | 3,708 Kb |
Large canvas mousemove experiment | Jibbon | 2,885 Kb |
Wavy Road with Dashes | Jonobr1 | 2,679 Kb |
Sign Up Form | Sicontis | 5,272 Kb |
CSS3 Form | Tusharbandal | 1,836 Kb |
NgEasyModal | Lorenzodianni | 4,159 Kb |
Electric worm | Jeffibacache | 2,377 Kb |
Spiralator 9000 | AdmiralPotato | 4,671 Kb |
Project_one | MOHIM | 9,592 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!