RecipeBox
How do I make an recipebox?
What is a recipebox? How do you make a recipebox? This script and codes were developed by Neeilan Selvalingam on 13 September 2022, Tuesday.
RecipeBox - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>recipeBox</title> <link rel='stylesheet prefetch' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class='container'> <h1>Recipe Box</h1><hr/> <div id = 'RecipeBox'/>
</div> <script src='https://fb.me/react-15.1.0.min.js'></script>
<script src='https://fb.me/react-dom-15.1.0.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
RecipeBox - Script Codes CSS Codes
.container { margin-top: 50px;
}
input { padding: 10px; width: 100%; margin: 2px;
}
h1 { text-align: center;
}
.small { color: grey;
}
RecipeBox - Script Codes JS Codes
'use strict';
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var RecipeBox = function (_React$Component) { _inherits(RecipeBox, _React$Component); function RecipeBox(props) { _classCallCheck(this, RecipeBox); var _this = _possibleConstructorReturn(this, _React$Component.call(this, props)); if (window.sessionStorage.getItem('_neeilan_recipes')) { var stateString = window.sessionStorage.getItem('_neeilan_recipes'); _this.state = JSON.parse(stateString); } else { _this.state = { recipes: [{ id: 'f4323j3', name: 'Cake', ingredients: ['Flour', 'Butter', 'Eggs', 'Sugar'] }, { id: 'g5s0l3b', name: 'Beef kabobs', ingredients: ['Beef', 'Salt', 'Pepper'] }] }; } _this.onRecipeAdded = _this.onRecipeAdded.bind(_this); _this.removeRecipe = _this.removeRecipe.bind(_this); _this.editRecipe = _this.editRecipe.bind(_this); _this.editCallback = _this.editCallback.bind(_this); return _this; } RecipeBox.prototype.componentWillUpdate = function componentWillUpdate(nextProps, nextState) { window.sessionStorage.setItem("_neeilan_recipes", JSON.stringify(nextState)); }; RecipeBox.prototype.render = function render() { var _this2 = this; var recipes = this.state.recipes.map(function (r) { return React.createElement(Recipe, { key: r.id, id: r.id, name: r.name, ingredients: r.ingredients, remove: _this2.removeRecipe, edit: _this2.editRecipe }); }); if (this.state.recipeToEdit) { return React.createElement(RecipeEditor, { recipe: this.state.recipeToEdit, saveCallback: this.editCallback }); } return React.createElement( 'div', { className: 'col-sm-6 col-sm-offset-3' }, React.createElement(RecipeForm, { onRecipeAdded: this.onRecipeAdded }), recipes ); }; RecipeBox.prototype.onRecipeAdded = function onRecipeAdded(recipe) { this.setState({ recipes: this.state.recipes.concat([recipe]) }); }; RecipeBox.prototype.removeRecipe = function removeRecipe(id) { var arrayWithRecipeRemoved = this.state.recipes.filter(function (recipe) { return recipe.id !== id; }); this.setState({ recipes: arrayWithRecipeRemoved }); }; RecipeBox.prototype.editRecipe = function editRecipe(id) { var recipeToEdit = this.state.recipes.filter(function (recipe) { return recipe.id == id; })[0]; this.setState({ recipeToEdit: recipeToEdit }); }; RecipeBox.prototype.editCallback = function editCallback(recipe) { var arrayWithOldRecipeRemoved = this.state.recipes.map(function (r) { if (r.id == recipe.oldId) { return recipe; } else { return r; } }); this.setState({ recipeToEdit: '', recipes: arrayWithOldRecipeRemoved }); }; return RecipeBox;
}(React.Component);
var RecipeForm = function (_React$Component2) { _inherits(RecipeForm, _React$Component2); function RecipeForm(props) { _classCallCheck(this, RecipeForm); var _this3 = _possibleConstructorReturn(this, _React$Component2.call(this, props)); _this3.state = { name: '', ingredients: '', recipes: [] }; _this3.updateName = _this3.updateName.bind(_this3); _this3.updateIngredients = _this3.updateIngredients.bind(_this3); _this3.addRecipe = _this3.addRecipe.bind(_this3); return _this3; } RecipeForm.prototype.render = function render() { var style = { addButtonStyle: { marginTop: 10, marginBottom: 30, padding: 10 } }; return React.createElement( 'div', null, React.createElement('input', { name: 'name', value: this.state.name, onChange: this.updateName, placeholder: 'Name of recipe' }), React.createElement('br', null), React.createElement('input', { name: 'ingredients', value: this.state.ingredients, onChange: this.updateIngredients, placeholder: 'Ingredients (comma separated)' }), React.createElement( 'button', { style: style.addButtonStyle, className: 'btn btn-primary col-sm-12', onClick: this.addRecipe }, 'Add' ) ); }; RecipeForm.prototype.addRecipe = function addRecipe() { if (!this.state.name) { if (this.state.ingredients) alert('Recipe name cannot be empty'); return; } var recipe = { id: encodeURIComponent(this.state.name + this.state.ingredients), name: this.state.name, ingredients: this.state.ingredients.split(",") }; this.setState({ name: '', ingredients: '' }); this.props.onRecipeAdded(recipe); }; RecipeForm.prototype.updateName = function updateName(e) { this.setState({ name: e.target.value }); }; RecipeForm.prototype.updateIngredients = function updateIngredients(e) { e.preventDefault(); this.setState({ ingredients: e.target.value }); }; return RecipeForm;
}(React.Component);
var RecipeEditor = function (_React$Component3) { _inherits(RecipeEditor, _React$Component3); function RecipeEditor(props) { _classCallCheck(this, RecipeEditor); var _this4 = _possibleConstructorReturn(this, _React$Component3.call(this, props)); _this4.state = _this4.props.recipe; _this4.state.ingredients = _this4.props.recipe.ingredients.join(); _this4.updateIngredients = _this4.updateIngredients.bind(_this4); _this4.updateName = _this4.updateName.bind(_this4); _this4.save = _this4.save.bind(_this4); return _this4; } RecipeEditor.prototype.render = function render() { return React.createElement( 'div', { className: 'col-sm-6 col-sm-offset-3' }, React.createElement('input', { name: 'name', value: this.state.name, onChange: this.updateName, placeholder: 'Name of recipe' }), React.createElement('br', null), React.createElement('input', { name: 'ingredients', value: this.state.ingredients, onChange: this.updateIngredients, placeholder: 'Ingredients (comma separated)' }), React.createElement('br', null), React.createElement( 'button', { style: { marginTop: 10, padding: 10 }, className: 'btn btn-primary', onClick: this.save, className: 'btn btn-primary col-sm-12' }, 'Save' ) ); }; RecipeEditor.prototype.save = function save() { var newIngredients = this.state.ingredients.split(","); var recipe = { oldId: this.state.id, id: encodeURIComponent(this.state.name + this.state.ingredients), name: this.state.name, ingredients: newIngredients }; if (recipe.name) { this.props.saveCallback(recipe); } else { alert('Recipe name cannot be empty'); } }; RecipeEditor.prototype.updateName = function updateName(e) { this.setState({ name: e.target.value }); }; RecipeEditor.prototype.updateIngredients = function updateIngredients(e) { this.setState({ ingredients: e.target.value }); }; return RecipeEditor;
}(React.Component);
var Recipe = function (_React$Component4) { _inherits(Recipe, _React$Component4); function Recipe(props) { _classCallCheck(this, Recipe); var _this5 = _possibleConstructorReturn(this, _React$Component4.call(this, props)); _this5.state = { showIngredients: false }; _this5.removeRecipe = _this5.removeRecipe.bind(_this5); _this5.editRecipe = _this5.editRecipe.bind(_this5); _this5.toggleIngredientsVisibility = _this5.toggleIngredientsVisibility.bind(_this5); return _this5; } Recipe.prototype.render = function render() { var ingredients = this.state.showIngredients ? this.props.ingredients[0] ? this.props.ingredients.map(function (i) { return React.createElement( 'h4', null, i, React.createElement('br', null) ); }) : React.createElement( 'i', null, 'No ingredients to show', React.createElement('br', null) ) : ''; return React.createElement( 'div', null, React.createElement('br', null), React.createElement( 'div', { className: 'row col-sm-12' }, React.createElement( 'span', { onClick: this.toggleIngredientsVisibility }, React.createElement( 'span', { className: 'lead col-sm-6' }, this.props.name ), React.createElement( 'span', { className: 'small col-sm-4 col-sm-offset-1', onClick: this.editRecipe }, 'EDIT' ), React.createElement( 'button', { onClick: this.removeRecipe, type: 'button', className: 'close' }, React.createElement( 'span', { 'aria-hidden': 'true' }, '×' ) ) ) ), React.createElement( 'div', { className: 'row' }, React.createElement( 'div', { className: 'small col-sm-8 col-sm-offset-1 col-xs-8 col-xs-offset-1' }, ingredients ) ), React.createElement('hr', null) ); }; Recipe.prototype.toggleIngredientsVisibility = function toggleIngredientsVisibility() { this.setState({ showIngredients: !this.state.showIngredients }); }; Recipe.prototype.removeRecipe = function removeRecipe() { this.props.remove(this.props.id); }; Recipe.prototype.editRecipe = function editRecipe() { this.props.edit(this.props.id); }; return Recipe;
}(React.Component);
ReactDOM.render(React.createElement(RecipeBox, null), document.getElementById('RecipeBox'));
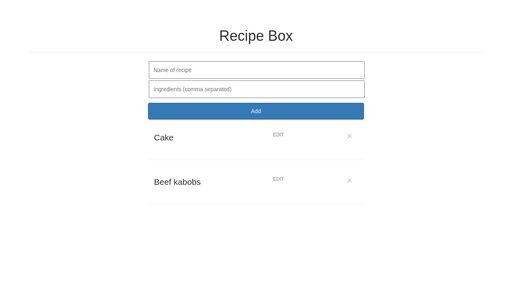
Developer | Neeilan Selvalingam |
Username | neeilan |
Uploaded | September 13, 2022 |
Rating | 3 |
Size | 6,082 Kb |
Views | 28,336 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
MarkdownPreviewer | 3,147 Kb |
Simon Game | 3,313 Kb |
Campleaderboard | 4,119 Kb |
Firebase-test | 2,374 Kb |
Milestones | 6,285 Kb |
Scribe | 3,525 Kb |
DecisionTree | 2,521 Kb |
Fctest | 14,173 Kb |
ResourcesSearch | 2,779 Kb |
NeeilWeather | 2,971 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Nice responsive team page | Infomiho | 3,139 Kb |
SVG Circle Progress | JMChristensen | 3,368 Kb |
CSS Piano | Dannystyle | 5,138 Kb |
IPhone5S SVG Space Grey | Onlinechris | 75,035 Kb |
Elephants Full screen site | Orrinward | 3,981 Kb |
404 Page | Saransh | 2,666 Kb |
SVG hamburger menu button | Elifitch | 2,602 Kb |
Drop Cap | Gsaiki | 1,571 Kb |
Christ the Redeemer | Prashantsani | 2,208 Kb |
Simple Linear Regression with Editable Table | Melatonind | 3,264 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!