Simon Game
How do I make an simon game?
What is a simon game? How do you make a simon game? This script and codes were developed by Neeilan Selvalingam on 13 September 2022, Tuesday.
Simon Game - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Simon Game</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <link href='https://fonts.googleapis.com/css?family=Montserrat:400,700' rel='stylesheet' type='text/css'>
<div class="container"> <div id="topCircle"> <button id="start" onclick="start()">Start</button><br> <span id="name">SIMON</span> <div id="score">1</div> <span id="steps">STEPS</span> <button id="reset" onclick="reset()">Reset</button><br> <button id="strict" onclick="strictToggle()">Strict</button></div> <button id="green" class="colors" onclick="enter(3)"></button> <button id="red" class="colors" onclick="enter(0)"></button> <br> <button id="yellow" class="colors" onclick="enter(2)"></button> <button id="blue" class="colors" onclick="enter(1)"></button>
</div> <script src="js/index.js"></script>
</body>
</html>
Simon Game - Script Codes CSS Codes
body{ background: #ff9e80;
}
.colors { width: 250px; height: 250px; border: solid 20px #212121;
}
button { background: #37474f; color: lightgray; border-radius: 10%; border-color:lightgray; font-size: 12px; height: 25px; width: 50px;
}
button:focus { outline: none;
}
#red { background: #dd2c00; border-radius: 0% 100% 0% 0%; margin-left: -4px;
}
#blue { background: blue; border-radius: 0% 0% 100% 0%; margin-left: -4px;
}
#yellow { background: #ffd600; border-radius: 0% 0% 0% 100%;
}
#green { background: green; border-radius: 100% 0% 0% 0%;
}
#topCircle { margin-top: -200px; position: absolute; width: 250px; height: 250px; z-index: 99; background: #424242; border: 15px solid #212121; border-radius: 50%; top: 390px; left: 110px; position: relative; text-align: center;
}
.container { width: 501px; /* border: solid 5px red; */ margin-left: auto; margin-right: auto;
}
#name { position: absolute; top: 50px; left: 40px; font-size: 3em; font-family: Montserrat, Arial; font-weight: bold; color: white;
}
#score { position: absolute; padding-left: 3px; left: 100px; top: 150px; border: 1px solid lightgray; width: 45px; overflow: hidden; background: rgb(41, 3, 5); font-family: Montserrat; color: red; font-weight: bold; font-size: 1.6em;
}
#steps { font-family: Montserrat, Arial; position: absolute; color: lightgray; top: 185px; left: 102px;
}
#reset { position: absolute; top: 115px; left: 160px;
}
#start { position: absolute; top: 115px; left: 40px;
}
#strict { position: absolute; top: 115px; left: 100px;
}
Simon Game - Script Codes JS Codes
var redSound = new Audio("https://s3.amazonaws.com/freecodecamp/simonSound1.mp3");
var blueSound = new Audio("https://s3.amazonaws.com/freecodecamp/simonSound2.mp3");
var yellowSound = new Audio("https://s3.amazonaws.com/freecodecamp/simonSound3.mp3");
var greenSound = new Audio("https://s3.amazonaws.com/freecodecamp/simonSound4.mp3");
var failure = new Audio("http://www.soundjay.com/misc/fail-buzzer-01.mp3");
var audio = [redSound, blueSound, yellowSound, greenSound];
var sequence = [];
var userSequence = [];
var score = 0;
var strict = false;
var winningScore = 20; //set score to win here
disableButtons(true);
document.getElementById("start").disabled = false;
function start() { document.getElementById("start").disabled = true; document.getElementById("strict").disabled = true; userSequence = []; disableButtons(true); init(); function init() { var number = Math.floor(Math.random() * 4); sequence.push(number); var i = 0; var count = setInterval(function() { activate(sequence[i]); i++; if (i >= sequence.length) { clearInterval(count); userTurn(); } }, 700); }
}
function userTurn() { setTimeout(function() { disableButtons(false); }, 500);
}
function arrayActivator(arr) { var i = 0; disableButtons(true); var count = setInterval(function() { activate(arr[i]); i++; if (i >= arr.length) { clearInterval(count); disableButtons(false); } }, 700);
}
function enter(input) { disableButtons(true); var roundCleared = true; userSequence.push(input); var j = userSequence.length - 1; activate(input); setTimeout(function() { disableButtons(false); }, 500); if (sequence[j] == input) { j++; } else { roundCleared = false; userSequence = []; failure.play(); if (strict) { reset(); } else { setTimeout(function() { arrayActivator(sequence) }, 1000); } } if (userSequence.length === sequence.length && roundCleared) { updateScore(); if (score === winningScore) { //score to win alert("YOU WIN!"); reset(); } else { setTimeout(function() { start(); }, 1000); } }
}
function activate(pos) { audio[pos].play(); switch (pos) { case 0: document.getElementById("red").style.background = "#ff1744 "; setTimeout(function() { document.getElementById("red").style.background = "#dd2c00"; }, 500); break; case 1: document.getElementById("blue").style.background = "#80d8ff"; setTimeout(function() { document.getElementById("blue").style.background = "blue"; }, 500); break; case 2: document.getElementById("yellow").style.background = "yellow"; setTimeout(function() { document.getElementById("yellow").style.background = "#ffd600"; }, 500); break; case 3: document.getElementById("green").style.background = "#76ff03 "; setTimeout(function() { document.getElementById("green").style.background = "green"; }, 500); break; }
}
function strictToggle() { if (strict) { strict = false; document.getElementById("strict").style.background = "#37474f"; } else { strict = true; document.getElementById("strict").style.background = "#dd2c00"; }
}
function updateScore() { score++; document.getElementById("score").innerHTML = (score + 1) + "";
}
function reset() { sequence = []; userSequence = []; score = -1; updateScore(); setTimeout(function() { start(); }, 1500);
}
function disableButtons(bool) { //true to disable, false to enable document.getElementById("reset").disabled = bool; document.getElementById("red").disabled = bool; document.getElementById("blue").disabled = bool; document.getElementById("yellow").disabled = bool; document.getElementById("green").disabled = bool;
}
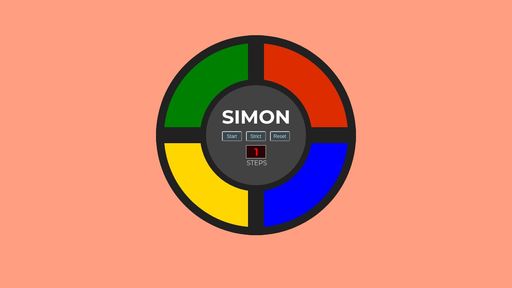
Developer | Neeilan Selvalingam |
Username | neeilan |
Uploaded | September 13, 2022 |
Rating | 3 |
Size | 3,313 Kb |
Views | 58,696 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Scribe | 3,525 Kb |
GameOfLife | 6,445 Kb |
RenderTest | 15,600 Kb |
NeeilWeather | 2,971 Kb |
UTSCMap | 3,292 Kb |
Parallaxtest | 1,152 Kb |
RecipeBox | 6,082 Kb |
Firebase-test | 2,374 Kb |
DecisionTree | 2,521 Kb |
Portfolio Website | 3,847 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
CSS3 Form Page Design | Rssatnam | 3,613 Kb |
Draggables in pure angular | Rlo206 | 5,167 Kb |
Css or Czz.. | Judag | 4,111 Kb |
Material design buttons | Fischaela | 4,381 Kb |
Use the Twitchtv JSON API | Roksanaop | 3,561 Kb |
Sinclair Research Computers | MattCowley | 3,068 Kb |
CSS3 Latte Art Logo | Esambino | 2,036 Kb |
Stylize Stories | Jvhti | 2,465 Kb |
SVG Animation | Thepheer | 4,793 Kb |
My Starter Kit For Codepen | Dkdesign | 2,012 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!