Twitch Zipline
How do I make an twitch zipline?
This is for the freecodecamp zipline: Use the Twitchtv JSON API.. What is a twitch zipline? How do you make a twitch zipline? This script and codes were developed by Devin on 23 November 2022, Wednesday.
Twitch Zipline - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Twitch Zipline</title> <link rel='stylesheet prefetch' href='http://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css'>
<link rel='stylesheet prefetch' href='http://cdnjs.cloudflare.com/ajax/libs/animate.css/3.2.3/animate.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.4.0/css/font-awesome.min.css">
<body class="center-block"> <div class="data-container"> <div class="tabs text-center"> <li class="tab all-tab" style="border-radius: 5px 0px 0px 0px;">All</li ><li class="tab online-tab">Online</li ><li class="tab offline-tab" style="border-radius: 0px 5px 0px 0px;">Offline</li> </div> <div class="data"> <div class="search"> <button class="search-button btn"><i class="fa fa-search"></i></button> <input class="text" id="search-input"></input> </div> <hr> </div> </div>
</body>
<div class="random"></div> <script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
Twitch Zipline - Script Codes CSS Codes
*:focus { outline: none;
}
.center-block{ background-color:#beaaf8;
}
.data-container{ margin: 0 auto;
}
.data{ background-color:white; border-radius:0px 0px 5px 5px; margin: 0 auto; width:300px; border:solid 1px #6d44ea; padding-bottom:12px;
}
.link{ color:black;
}
.link:hover{ color:black;
}
#storbeck{ color:red;
}
.data li{ list-style:none; font-size:20px; padding:10px; padding-left:20px; background-color:#f9f9f9; margin-top:12px;
}
.data li i{ position:relative; float:right; padding-top:13px;
}
.tabs{ width:300px; height:60px; margin: 0 auto;
}
.tabs li{ display: inline-block;
}
.tab{ width:100px; height:60px; padding-top:20px; background-color:#8963f2; font-weight:800; color:white; text-shadow:1px 1px 10px black;
}
.tab:hover{ background-color:#9476ed; border: solid 1px white; box-shadow:1px 1px 10px black; padding-top:19px; cursor:pointer;
}
.search{ padding:5px; height:20px; width:300px;
}
.search-expand{ animation-name: expand; animation-duration:2s; animation-fill-mode:forwards;
}
.search-button{ border-radius:50px 0px 0px 50px; background-color:white; width:10px; color:#8963f2;
}
.text{ border:solid 1px; border-radius:5px 5px 5px 5px; height:25px; width:0px;
}
.profile-image{ width:50px; border-radius:50px;
}
.status-text{ //display:none; font-size:11px;
}
/* offline users */
.offline-user{
}
.display-none{ display:none;
}
@keyframes expand{ from {width: 0px; background-color:#6d44ea;} to {width: 255px;}
}
Twitch Zipline - Script Codes JS Codes
$(document).ready(function(){ $(".text").addClass("search-expand");
});
//where the live users display names will be pushed to make sure they arn't appended twice.
var streaming = [];
//users
var users = [ ["freecodecamp"],["storbeck"],["terakilobyte"],["habathcx"],["RobotCaleb"],["thomasballinger"],["noobs2ninjas"],["beohoff"], ["medrybw"]
];
//when clicking the search icon pop out search bar.
$(".search-button").click(function(){ $(".text").toggleClass("search-expand"); $(".text").focus(); $(".offline-user").fadeOut(); $(".online-user").fadeOut();
});
$(".text").click(function(){ $(".offline-user").fadeOut(); $(".online-user").fadeOut();
});
//when clicking online, show online users.
$(".online-tab").click(function(){ console.log("hi"); $(".offline-user").fadeOut() $(".data").fadeOut(); $(".data").fadeIn(); $(".online-user").fadeIn()
});
//When clicking on offline, show offline users.
$(".offline-tab").click(function(){ console.log("hi"); $(".offline-user").fadeIn() $(".data").fadeOut(); $(".data").fadeIn(); $(".online-user").fadeOut()
});
$(".all-tab").click(function(){ console.log("hi"); $(".data").fadeOut(); $(".data").fadeIn(); $(".offline-user").fadeIn() $(".online-user").fadeIn() console.log(input.value); $(".found-now").fadeOut();
});
//search functionality
var input = document.getElementById('search-input');
var input_expression = new RegExp("ReGeX" + input.value + "ReGeX");
$("#search-input").on("keyup", function(){ for(x = 0; x < users.length; x++){ console.log(input.value); //compare only the full input.value with the same length of letters from each user. if(users[x].join().substr(0, input.value.length).toLowerCase() == input.value.toLowerCase()){ console.log(users[x]); //loop through the elements, pull the element with the username that passes the above if statment. for(i = 0; i < $(".offline-user span").length - 1; i++){ if($(".offline-user span")[i].innerHTML.toLowerCase() == users[x].toString().toLowerCase()){ console.log("WEEE") $(".offline-user")[i].style.display = "block"; } else{ $(".offline-user")[i].style.display = "none"; } } //Do the same for online users. for(i = 0; i < $(".online-user span").length + 1; i++){ if($(".online-user span")[i].innerHTML.toLowerCase() == users[x].toString().toLowerCase()){ console.log("WEEE") $(".online-user")[i].style.display = "block"; } } } }
});
$(".fa-search").click(function(){ console.log(input.value);
});
//for each online user, grab the specific data and put it in a list tag.
for(x = 0; x < users.length;x++){
$.getJSON("https://api.twitch.tv/kraken/streams/" + users[x][0], function(json){ //grab the specific data if(json.display_name !== null){ //if the user is online, grab the channel info from streams json request. var display_name = json.stream.channel.display_name; streaming.push(display_name); var profile_image = json.stream.channel.logo; var url = json.stream.channel.url; var status = json.stream.channel.status; var live = "<i style='color:green;' class='fa fa-check'></i>"; //if no logo, use default. if(profile_image == null){ profile_image = "http://www.rudebaguette.com/assets/default_profile.gif"; } if(status.length > 30){ status = status.substring(0, 30) + "..."; } } $(".data").append("<a style='text-decoration: none;' class='link' href='" + url + "'>" + "<li class='online-user'>" + "<img class='profile-image' src='" + profile_image + "'>" + " " + "<span>" + display_name + "</span>" + " " + live + "<br><small class='status-text'><b>" + status + "</b></small>" + "</li>" + "</a>"); //on success, capture users that are not online. //this loop will pick up all the channel data if the user is not online. The streams json request will pick up all channel data for those users online. success: for(x = 0; x < users.length;x++){ $.getJSON("https://api.twitch.tv/kraken/channels/" + users[x][0], function(json){ //grab the specific data if(streaming.indexOf(json.display_name) != -1){ console.log("Has allready been appended as live.") } else{ var display_name = json.display_name; var profile_image = json.logo; var url = json.url; var status = json.status; var live = "<i style='color:red;' class='fa fa-times'></i>" //if there is no profile image, use the default. if(profile_image == null){ profile_image = "http://www.rudebaguette.com/assets/default_profile.gif"; } if(status == null){ status = ""; } if(status.length > 30){ status = status.substring(0, 30) + "..."; } //putting each users data into a list item $(".data").append("<a style='text-decoration: none;' class='link' href='" + url + "'>" + "<li class='offline-user'>" + "<img class='profile-image' src='" + profile_image + "'>" + " " + "<span>" + display_name + "</span>" + live +"<br><small class='status-text'><b>" + status + "</b></small>" + "</li>" + "</a>"); } }); } });
}
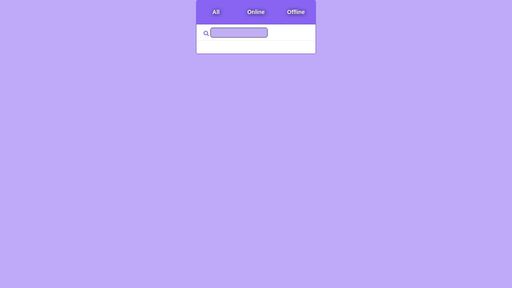
Developer | Devin |
Username | edwin0258 |
Uploaded | November 23, 2022 |
Rating | 3 |
Size | 4,084 Kb |
Views | 12,144 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Portfolio Zipline | 5,335 Kb |
Calculator | 4,368 Kb |
React Composer Widget | 6,528 Kb |
Pomodoro Clock | 5,289 Kb |
Artist Card Widget | 7,159 Kb |
Simple Svg Loading Bar | 2,693 Kb |
Descriptive Tags | 1,953 Kb |
Sonar Svg | 2,495 Kb |
Mondrian Generator | 21,771 Kb |
A Pen by Devin | 1,521 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Velocity.js custom stagger | Tommiehansen | 4,805 Kb |
Haml Calendar | Katydecorah | 5,643 Kb |
Birthday Party Starter | Aussieyang | 1,629 Kb |
CSSOff 2013 Submission | Codewunder | 14,766 Kb |
Layout 11 | Altynai | 1,690 Kb |
Obligatory CSS3 UI Nav | Romandiaz | 9,017 Kb |
Pure CSS Animated Photo Stack | Depthdev | 2,486 Kb |
A Pen by Markku Lehmonen | SharpDal | 7,804 Kb |
A Pen by Patrick Cox | Pcridesagain | 2,899 Kb |
Electric worm | Jeffibacache | 2,377 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!