D3 Force-directed Graph Project
How do I make an d3 force-directed graph project?
Built for freeCodeCamp using D3 and jQuery. Modeled after https://bl.ocks.org/mbostock/4062045, http://bl.ocks.org/sathomas/11550728, and http://www.d3noob.org/2013/03/d3js-force-directed-graph-example-basic.html.. What is a d3 force-directed graph project? How do you make a d3 force-directed graph project? This script and codes were developed by Zac Clemans on 14 January 2023, Saturday.
D3 Force-directed Graph Project - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>D3 Force-directed Graph Project</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="container"></div> <script src='http://cdnjs.cloudflare.com/ajax/libs/d3/3.5.16/d3.min.js'></script>
<script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.2/jquery.min.js'></script> <script src="js/index.js"></script>
</body>
</html>
D3 Force-directed Graph Project - Script Codes CSS Codes
.container { width: 800px; margin: 20px auto 0 auto; background: rgb(70, 70, 130); border-radius: 10px;
}
.node { stroke: #fff; stroke-width: 1px;
}
.link { stroke: #999; stroke-opacity: .6;
}
.tooltip { display: none; position: absolute; padding: 2px; color: white; background: rgb(52, 52, 54); border-radius: 5px;
}
D3 Force-directed Graph Project - Script Codes JS Codes
var api = "https://www.freecodecamp.com/news/hot", width = 800, height = 800;
var svg = d3.select('.container').append('svg') .attr('width', width) .attr('height', height);
$.ajax({ url: api, dataType: 'json', success: function(d) { var nodes = [], links = []; // Separate out relevant information from the JSON file // and store in the links variable d.forEach(function(item) { var link = { source: item.author.username, target: item.link.match(/([^:\/\n]+\.[a-z]+(?=\/))/)[0], value: 10, img: item.author.picture }; links.push(link) }); // Separate out each author and domain as a node with no duplicates links.forEach(function(link) { // If author or domain already exists in the nodes variable, // Increase it's weight by 2 (for a bigger node) if (nodes[link.source]) { nodes[link.source].value += 2; } if (nodes[link.target]) { nodes[link.target].value += 2; } // Check if author is already present in nodes. If not, // create a new node for that author link.source = nodes[link.source] || (nodes[link.source] = { name: link.source, type: 'author', value: 5, img: link.img }); // Same as above for domains link.target = nodes[link.target] || (nodes[link.target] = { name: link.target, type: 'domain', value: 5 }); }); var force = d3.layout.force() .nodes(d3.values(nodes)) .links(links) .size([width, height]) .linkDistance(80) .charge(-200) .start(); // Create a defs section and store a pattern for each // author's avatar. var defs = svg.append('defs') var images = defs.selectAll('pattern') .data(force.nodes()) .enter() .append('pattern') .attr('id', function(d) { return d.name; }) .attr('x', 0) .attr('y', 0) .attr('patternUnits', 'objectBoundingBox') // use objectBoundingBox to be bounded by circle .attr('width', function(d) { // diameter of the circle is d.value * 2 // fits the image within the bounding circle return d.value * 2; }) .attr('height', function(d) { return d.value * 2; }) .append('image') .attr('x', 0) .attr('y', 0) .attr('width', function(d) { // must be the same as the width (and height) of the pattern return d.value * 2; }) .attr('height', function(d) { return d.value * 2; }) .attr('xlink:href', function(d) { return d.img; }) var tooltip = d3.select('body').selectAll('.tooltip') .data(force.nodes()) .enter() .append('div') .attr('class', 'tooltip'); var link = svg.selectAll('.link') .data(force.links()) .enter() .append('line') .attr('class', 'link'); var node = svg.selectAll('.node') .data(force.nodes()) .enter() .append('circle') .attr('class', 'node') .attr('r', function(d) { return d.value; }) // fill with the author avatar pattern unless domain .attr('fill', function(d) { return d.type == 'author' ? 'url(#' + d.name + ')' : 'rgb(60, 60, 60)' }) .call(force.drag) .on('mouseover', function(d) { tooltip .html(function() { return d.type == 'author' ? d.name + "<br/>has posted " + ((d.value - 5) / 2 == 0 ? 1: ((d.value - 5) / 2) + 1) + " time(s)" : d.name + "<br/>has been posted " + ((d.value - 5) / 2 == 0 ? 1: ((d.value - 5) / 2) + 1) + " time(s)"; }) .style('display', 'inline') .style('cursor', 'pointer') .style('top', (d3.event.pageY - 45) + 'px') .style('left', (d3.event.pageX + 5) + 'px'); d3.select(this).style('opacity', 0.5) }) .on('mouseout', function() { tooltip.style('display', 'none'); d3.select(this).style('opacity', 1); }) force.on('tick', function() { link.attr('x1', function(d) { return d.source.x; }) .attr('y1', function(d) { return d.source.y; }) .attr('x2', function(d) { return d.target.x; }) .attr('y2', function(d) { return d.target.y; }); node.attr('cx', function(d) { return d.x; }) .attr('cy', function(d) { return d.y; }) }) }
});
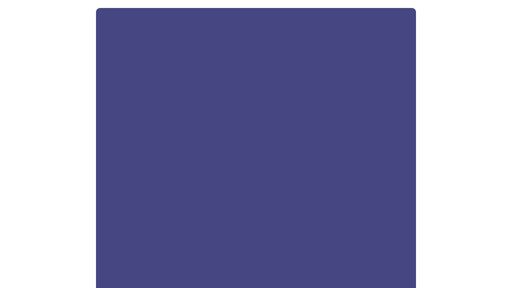
Developer | Zac Clemans |
Username | thalpha |
Uploaded | January 14, 2023 |
Rating | 3 |
Size | 3,348 Kb |
Views | 8,096 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Tic-Tac-Toe | 5,278 Kb |
Personal Portfolio | 3,289 Kb |
Twitch Streamer Status | 3,948 Kb |
D3 Heatmap Project | 3,647 Kb |
Markdown Previewer | 3,771 Kb |
FCC Camper Leaderboard | 6,801 Kb |
Recipe Box Redux Refactor | 11,597 Kb |
Recipe Box | 7,954 Kb |
Hero arrow test | 1,482 Kb |
Random Chuck Norris Joke Generator | 2,586 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
A Pen by Malith Hettiarachchi | MalZiiirA | 5,531 Kb |
Hamburger Menu Animation | Salmanraza | 2,580 Kb |
Eunice A | Ejbronze | 2,203 Kb |
Very Simple Slider | Doodlemarks | 2,682 Kb |
Headroom.js demo | WickyNilliams | 3,982 Kb |
Working around OS X Dynamic Scrollbars | Jrjenk | 2,279 Kb |
Bootstrap 3 Price Table | Honglio | 2,655 Kb |
Fullscreen audio play button | 72 | 2,148 Kb |
Border image | JohnRiordan | 2,120 Kb |
Horizontal Navigation with Floats | Gymratpacks | 5,403 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!