DeJong attractor
How do I make an dejong attractor?
A recreation of Jashkenas's de Jong attractor in ES6. Drag to regenerate, wait until it will settle up.. What is a dejong attractor? How do you make a dejong attractor? This script and codes were developed by Endre Simo on 28 November 2022, Monday.
DeJong attractor - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>DeJong attractor</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <button id="export" class="btn">Export to image</button> <script src="js/index.js"></script>
</body>
</html>
DeJong attractor - Script Codes CSS Codes
@import url(https://fonts.googleapis.com/css?family=Open+Sans:400,300italic,300,400italic,600);
body { margin: 0; padding: 0; overflow: hidden; background-color: #000;
}
canvas { position: absolute; top: 50%; left: 50%;
}
.btn { font-family: 'Open Sans', sans-serif; font-weight: 300; margin: 0 auto; padding: 0.3rem; cursor: pointer; background: none; border: 1px solid #fff; color: #fff; font-size: 18px; position: absolute; left: 45%; z-index: 999; bottom: 10px;
}
DeJong attractor - Script Codes JS Codes
'use strict';
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
var DeJongAttractor = function () { function DeJongAttractor(size, density, sensitivity, iterations) { var _this = this; _classCallCheck(this, DeJongAttractor); this.mouseX = 0; this.mouseY = 0; this.size = size || 800; this.canvas = document.createElement('canvas'); this.canvas.width = this.canvas.height = this.size; this.canvas.style.width = this.canvas.style.height = this.size + 'px'; this.canvas.style.marginTop = this.canvas.style.marginLeft = '-' + this.size / 2 + 'px'; document.body.appendChild(this.canvas); this.ctx = this.canvas.getContext('2d'); this.steps = 0; this.stopped = false; this.limit = 800; this.initDensity = density || 12; this.sensitivity = sensitivity || 0.02; this.iterations = iterations || 8000; this.reseed(); this.animate(); var button = document.getElementById('export'); document.addEventListener('mousedown', function (event) { return _this.pause(event); }, false); document.addEventListener('mousemove', function (event) { return _this.record(event); }, false); document.addEventListener('mouseup', function (event) { return _this.resume(event); }, false); button.addEventListener('click', function (event) { return _this.exportImage(event); }, false); } DeJongAttractor.prototype.reseed = function reseed() { this.clear(); this.seed(); this.plot(1); }; DeJongAttractor.prototype.animate = function animate() { var _this2 = this; this.interval = requestAnimationFrame(function () { return _this2.animate(); }); this.tick(); }; DeJongAttractor.prototype.stopLoop = function stopLoop() { clearInterval(this.interval); }; DeJongAttractor.prototype.tick = function tick() { if (this.stopped) { this.reseed(); } this.steps += 1; this.plot(5); if (this.steps > this.limit) { this.stopLoop(); } }; DeJongAttractor.prototype.pause = function pause(event) { event.preventDefault(); if (event.target.tagName.toLowerCase() == 'canvas') { this.stopped = true; if (!this.interval) { this.loop(); } } }; DeJongAttractor.prototype.record = function record(event) { if (this.stopped) { this.mouseX = event.pageX - this.canvas.offsetLeft; this.mouseY = event.pageY - this.canvas.offsetTop; } }; DeJongAttractor.prototype.resume = function resume(event) { this.stopped = false; this.steps = 0; }; DeJongAttractor.prototype.clear = function clear() { this.image = this.ctx.createImageData(this.size, this.size); var x = undefined, y = undefined; // create and populate a two dimensional array this.density = function (size) { var i = undefined, results = undefined; results = new Array(); for (x = 0; x < size; x++) { results.push(function () { var j = undefined, results1 = undefined; results1 = new Array(); for (y = 0; y < size; y++) { results1.push(0); } return results1; }()); } return results; }(this.size); this.maxDensity = 0; }; DeJongAttractor.prototype.seed = function seed() { this.xSeed = (this.mouseX * 2 / this.size - 1) * this.sensitivity; this.ySeed = (this.mouseY * 2 / this.size - 1) * this.sensitivity; this.x = this.size / 2; this.y = this.size / 2; }; DeJongAttractor.prototype.populate = function populate(samples) { var _this3 = this; var x, y, row; var iterations = this.iterations; for (var i = 0, len = samples * iterations; i < len; i++) { x = (Math.sin(this.xSeed * this.y) - Math.cos(this.ySeed * this.x)) * this.size * 0.2 + this.size / 2; y = (Math.sin(-this.xSeed * this.x) - Math.cos(-this.ySeed * this.y)) * this.size * 0.2 + this.size / 2; this.density[Math.round(x)][Math.round(y)] += this.initDensity; this.x = x; this.y = y; } this.maxDensity = Math.log(Math.max.apply(Math, function () { var row, results = new Array(); for (var i = 0, len = _this3.density.length; i < len; i++) { row = _this3.density[i]; results.push(Math.max.apply(Math, row)); } return results; }.call(this))); }; DeJongAttractor.prototype.plot = function plot(samples) { this.populate(samples); var data = this.image.data; var hexToRgb = function hexToRgb(hex) { var red = parseInt(hex) >> 16 & 0xff; var green = parseInt(hex) >> 8 & 0xff; var blue = parseInt(hex) & 0xff; return { r: red, g: green, b: blue }; }; for (var x = 0; x < this.size; x++) { for (var y = 0; y < this.size; y++) { var idx = (x * this.size + y) * 4; var dens = this.density[x][y]; data[idx + 3] = 255; if (dens <= 0) continue; var light = Math.log(dens) / this.maxDensity * 255; var current = data[idx]; var red = Math.min(255, hexToRgb(current).r + 255 / light); var green = Math.min(255, hexToRgb(current).g + 255 / light); var blue = Math.min(255, hexToRgb(current).b + 255 / light); var rgb = red << 16 & 0xff | green << 8 & 0xff | blue & 0xff; var color = this.illuminate(light, rgb); data[idx] = red / color; data[idx + 1] = color / green; data[idx + 2] = color; } } this.ctx.putImageData(this.image, 0, 0); }; DeJongAttractor.prototype.illuminate = function illuminate(a, b) { return (a * b >> 7) + (a * a >> 8) - (a * a * b >> 15); }; DeJongAttractor.prototype.exportImage = function exportImage(event) { event.stopPropagation(); this.ctx.fillStyle = "#333333"; this.ctx.font = 'bold 16px Helvetica, Arial, sans-serif'; this.ctx.textBaseline = "top"; var textSize = this.ctx.measureText("esimov.com"); //this.ctx.fillText("esimov.com", this.size - textSize.width - 10, 5); //retrieve canvas image as data URL: var dataURL = this.canvas.toDataURL("image/png"); //open a new window of appropriate size to hold the image: var imageWindow = window.open("", "DeJongAttractor", "left=0,top=0,width=" + this.size + ",height=" + this.size + ",toolbar=0,resizable=0"); //write some html into the new window, creating an empty image: imageWindow.document.write("<title>DeJong Attractor</title>"); imageWindow.document.write("<img id='exportImage'" + " alt=''" + " height='" + this.size + "'" + " width='" + this.size + "'" + " style='position:absolute;left:0;top:0'/>"); imageWindow.document.close(); //copy the image into the empty img in the newly opened window: var exportImage = imageWindow.document.getElementById("exportImage"); exportImage.src = dataURL; }; return DeJongAttractor;
}();
(function () { return new DeJongAttractor(800);
})(window);
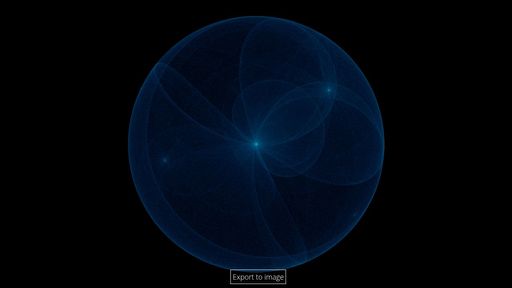
Developer | Endre Simo |
Username | esimov |
Uploaded | November 28, 2022 |
Rating | 4.5 |
Size | 6,155 Kb |
Views | 8,096 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
Worley noise cellular pattern | 8,070 Kb |
Perlin metaball | 7,396 Kb |
Flipping Clock | 3,804 Kb |
Navier Stoke Fluid Simulation | 8,584 Kb |
Landscape | 3,792 Kb |
Growing tree | 5,833 Kb |
Spirograph | 2,519 Kb |
Perlin Noise based Minecraft Tunnel | 9,853 Kb |
404 canvas page | 7,078 Kb |
Minecraft map generator | 7,723 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
DNA Double Helix | Hugo | 5,112 Kb |
Sencha Touch 2.3.1 Basic Grid Example | Trozdol | 2,770 Kb |
My Interests | Anshusaxenaarora | 2,015 Kb |
Matrix | Stathisnikolaidis | 1,922 Kb |
Simple Responsive Text | Fbrz | 2,282 Kb |
Toggle Time | Petebot | 5,345 Kb |
Video Player Custom Controls | EleftheriaBatsou | 3,665 Kb |
Bloomberg Style Link Hover | Gil-- | 1,609 Kb |
Animated skewed panes | NyX | 4,462 Kb |
Underlined form fields | Mitchdot | 2,323 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!