NeeilCalc
How do I make an neeilcalc?
What is a neeilcalc? How do you make a neeilcalc? This script and codes were developed by Neeilan Selvalingam on 13 September 2022, Tuesday.
NeeilCalc - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>neeilCalc</title> <link rel="stylesheet" href="css/style.css">
</head>
<body> <head>
<link href='https://fonts.googleapis.com/css?family=Share+Tech+Mono' rel='stylesheet' type='text/css'></head>
<div id="calculator">
<table> <tr> <td colspan="3" id="display"></td> </tr> <tr> <td><button class="btn" onclick="input(1)">1</buttton></td> <td><button class="btn" onclick="input(2)">2</buttton></td> <td><button class="btn" onclick="input(3)">3</buttton></td> </tr> <tr> <td><button class="btn" onclick="input(4)">4</buttton></td> <td><button class="btn" onclick="input(5)">5</buttton></td> <td><button class="btn" onclick="input(6)">6</buttton></td> </tr> <tr> <td><button class="btn" onclick="input(7)">7</buttton></td> <td><button class="btn" onclick="input(8)">8</buttton></td> <td><button class="btn" onclick="input(9)">9</buttton></td> </tr> <tr> <td><button class="btn op" onclick="input('+')">+</buttton></td> <td><button class="btn" onclick="input(0)">0</buttton></td> <td><button class="btn op" onclick="input('-')">-</buttton></td> </tr> <tr> <td><button class="btn op" onclick="input('*')">*</buttton></td> <td><button class="btn op" onclick="input('/')">/</buttton></td> <td><button class="btn op" onclick="input('.')">.</buttton></td> </tr> <tr> <td><button class="btn op" onclick="input('NEG')"> ±</buttton></td> <td><button class="btn op" onclick="input('C')">CE</buttton></td> <td><button class="btn calc" onclick="input('=')">=</buttton></td> </tr> <tr></tr>
</table>
</div> <script src="js/index.js"></script>
</body>
</html>
NeeilCalc - Script Codes CSS Codes
html,body { height:100%; background: rgb(1,15,22); font-family: Helvetica, Arial;
}
#display { width: 300px; background: rgb(35,182,79); overflow:hidden; color: rgb(22,118,15); padding-right: 5%; font-size: 2.1em; font-family: 'Share Tech Mono', 'Arial'; font-weight: bold; text-align: right; margin-left: -5; border: solid rgb(221,221,221); border-width:15px 25px 20px 25px;
}
table { height: 600px; width: 430px; margin: 5% auto 0% auto; background: rgb(230,230,230); border-radius:5px; box-shadow: 0px 0px 50px 0px rgb(38,57,83);
}
.btn { background: #AEC6CF; width: 45%; height: 75%; border-radius: 70%; color: white; border-style: none; font-size: 1em;
}
.btn:active { width: 44%; height: 74%;
}
.op { background:rgb(103,148,165) ;
}
.calc{ background:rgb(227,128,128) ;
}
.btn:hover { background: #de6868; font-weight: bold;
}
.btn:focus { outline:none;
}
td{ height: 14%; text-align: center; width:30%;
}
table:hover { box-shadow: 0px 0px 120px 0px rgb(48,71,103);
}
table:hover #display { background-color: rgb(52,216,101); color: rgb(34,179,78);
}
NeeilCalc - Script Codes JS Codes
var display="";
var values= [];
function input (value){ if (value==="C"){ clear(); } else if (value==="="){ display = process(); //process() returns final answer } else if (value==="NEG"){ values.push("NEG"); display+="-"; } else{ values.push(value); display+=""+value; } updateDisplay();
}
function process() { joinNums(); joinDecimals(); //***BUG with decimals longer than 1*** FIXED console.log(values); neg(); repeatCatcher(); //if consecutive operations are stringed together, return value of last operation rather than NaN/error multiplyVals(); addVals(); //console.log(values); //max of 8 decimal places return Math.round(values[0]*100000000)/100000000;
}
function neg(){ //call after joining nums and decimals for (var i=0; i<values.length-1; i++){ if (values[i]==="NEG"){ values[i+1]=values[i+1]*(-1); values[i]="$"; } } values = values.filter(filter$);
}
function joinNums(){ //Joins consecutive digits into numbers for (var i=0; i<values.length-1; i++){ if (typeof(values[i])==="number" && typeof(values[i+1])==="number") { values[i+1]=Number(values[i]+""+values[i+1]); values[i]="$"; } } values = values.filter(filter$);
}
function joinDecimals() { //Call after joinNums var divisionFactor=10; for (var i=0; i<values.length-1; i++){ if (values[i]==="."){ var decimalLength = (""+values[i+1]).length; divisionFactor= Math.pow(10,decimalLength); //to account for decimal portions of varying length ex- 0.5 vs 0.55 values[i+1]=values[i-1]+values[i+1]/divisionFactor; values[i]="$"; values[i-1]="$"; } } values = values.filter(filter$);
}
var filter$ = function(val){ return (val!=="$");
}
function multiplyVals() { //Only call after joinNums + joinDecimals for (var i=0; i<values.length-1; i++){ if(values[i]==="/"){ //division values[i]="*"; values[i+1]=(1/values[i+1]); } if (values[i]==="*"){ values[i+1]=values[i+1]*values[i-1]; values[i]="$"; values[i-1]="$"; } // if(values[i]==="/"){ //division // not needde if adjusted before multiplying // values[i+1]=values[i-1]/values[i+1]; // values[i]="$"; // values[i-1]="$"; // } } values = values.filter(filter$);
}
function addVals() { //Call after multiplyVals for (var i=0; i<values.length-1; i++){ if(values[i]==="-"){ //subtraction values[i]="+"; values[i+1]=(-1*values[i+1]); } if (values[i]==="+"){ values[i+1]=values[i+1]+values[i-1]; values[i]="$"; values[i-1]="$"; } } values = values.filter(filter$);
}
function repeatCatcher() { for (var i=0; i<values.length-1; i++){ if (typeof(values[i])!=="number" && typeof(values[i+1])!=="number") { values[i]="$"; } }
}
function clear(){ display=""; values=[];
}
function updateDisplay() { if (display.length>14){ display="..."+display.substring(display.length-15,display.length); } document.getElementById("display").innerHTML=display;
}
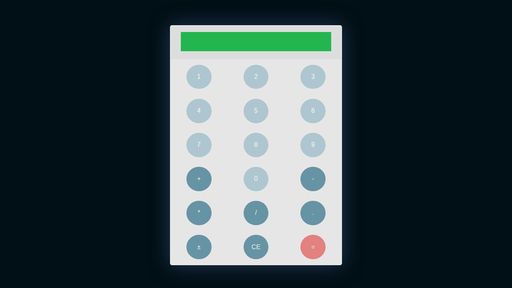
Developer | Neeilan Selvalingam |
Username | neeilan |
Uploaded | September 13, 2022 |
Rating | 3 |
Size | 3,209 Kb |
Views | 34,408 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
MarkdownPreviewer | 3,147 Kb |
NeeilWiki | 2,824 Kb |
Portfolio Website | 3,847 Kb |
Campleaderboard | 4,119 Kb |
GameOfLife | 6,445 Kb |
ResourcesSearch | 2,779 Kb |
Scribe | 3,525 Kb |
UTSCMap | 3,292 Kb |
A Pen by Neeilan Selvalingam | 9,533 Kb |
RenderTest | 15,600 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
Multiple jCarousel | Pafnuty | 2,461 Kb |
Jstam.com Home Page | Jstam | 10,558 Kb |
Mega Menu by Joni | Asakasinsky | 3,171 Kb |
Animated skewed panes | NyX | 4,462 Kb |
CSS only simple parallax scroll | Stanssongs | 3,708 Kb |
Collapsing Widget | Er40 | 4,279 Kb |
Canvas Fireworks | Jackrugile | 6,200 Kb |
Image grid with captions | Mchernin34 | 2,222 Kb |
Amazing CSS Menu with Notification Badges | Faizanasad | 2,549 Kb |
Electric worm | Jeffibacache | 2,377 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!