Rails consolidation part2 - Model
How do I make an rails consolidation part2 - model?
What is a rails consolidation part2 - model? How do you make a rails consolidation part2 - model? This script and codes were developed by DAWEI DAI on 29 September 2022, Thursday.
Rails consolidation part2 - Model - Script Codes HTML Codes
<!DOCTYPE html>
<html >
<head> <meta charset="UTF-8"> <title>Rails consolidation part2 - Model</title> <link rel='stylesheet prefetch' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css'> <link rel="stylesheet" href="css/style.css">
</head>
<body> <div class="container-fluid"> <div class="jumbotron"> <div class="row"> <div class="col-xs-12"> <h1 class="text-center">Rails part2</h1> <h2 class="text-center"><em>Active Record Model</em></h2> <!--div class="thumbnail"><image src="https://c2.staticflickr.com/4/3689/10613180113_fdf7bcd316_b.jpg"> <div class="caption text-center">Dr. Norman Borlaug, second from left, trains biologists in Mexico on how to increase wheat yields - part of his life-long war on hunger.</div> </div--> <div class="col-xs-12 col-sm-10 col-sm-offset-1 col-md-12 col-md-offset-0"> <h3 class="text-center text-primary">Lesson 1</h3> <pre>
also you may see change method
the same
then the roll back migration will be inferred from the change method
If this table needs to have a model on top of it, then primary key (id) is required, othewise, not, :id=> false
object to object, id to id (when linking associatiation)
post.user_id = user.id
post.user = user
def change add_column(:posts, :user_id, :integer)
end
On windows, If you find this error when rails s, solution is here:
http://stackoverflow.com/questions/17643897/cannot-load-such-file-sqlite3-sqlite3-native-loaderror-on-ruby-on-rails
your file to be modified is here C:\RailsInstaller\Ruby2.0.0\lib\ruby\gems\2.0.0\specifications\sqlite3-1.3.7-x86-mingw32.gemspec
Also check https://www.gotealeaf.com/lessons/bfbb8c25/home/search_discussion?term=sqlite3
Search this post:
David Sebesta
Heads up for Windows users
maybe a good time to switch to mac, this is one of the quacky issues on Windows. I have ruby 2.0.0, which solved other's people's problem, but not mine
Using vagrant you can have a vm running in a matter of minutes
Assignment: Post model
Setting up a model requires an associated table in the database. By convention in Rails, the table name should be the lowercase plural of the model name.
Example: Post model should have a table in the database called posts.
Here's how to set up the model:
1 First, create a migration (create_posts) to modify the database. In this migration, we want to create the table. Rails migrations are the only place where we want to use generators. Issue this command from the terminal within your project directory: rails generate migration create_posts
2 Open the newly created migration file in your code editor, and take a look at the migration file. Use the create_table method to create the necessary table and columns. We want: a url and title, which should both be strings and a description which should be text. t.string :url,:title t.text :description t.timestamps
3 From the terminal again, issue this command: rake db:migrate (you may need to do rake db:create first, if you're using mysql or postgres, for sqlite, no need). If you're getting a rake error, you can try adding bundle exec before the rake command.
4 Then take a look at your database and see that you have a posts table, with three columns: url, title and description.
5 Create a Post model file: under app/models directory, create a post.rb file put class Post < ActiveRecord::Base; end
6 Open rails console, and create your first Post object: Post.create(title: "My first post", description: "I sure hope this works!", url: "www.yahoo.com"). Verify by looking at your database that this worked.
rake db:migration will run all the un-run migrations for you
Assignment: 1:M between User and Post
It's a two step process:
First, we need to modify the database to create a new foreign key column on the posts table to support the one to many association. Remember, the foreign key column always goes on the 'many' side. Hint: generate a migration file, then use Rails migration syntax to create a new column.
def change add_column(:posts, :user_id, :integer) -- fk should always be integer
end
Now, we can declare the associations in the models. has_many :xxxs belongs_to :xxx
quick test to see if model is wired up with table Post
should see something the schema view
trick: In rails console, type "Post".tableize
=> "posts"
create users table
make surey your migration runs clearly end to end (consider your historic migration, no contraditary to each other migration)
Add timestamps to posts table
def change add_column :posts, :created_at, :datetime (column name is important, rails can maintain it) add_column :posts, :updated_at, :datetime
end
create User model
in user has_many :posts
in post belongs_to :user
user = User.create(username: 'bob')
user.posts shows []
post = Post.create(title:"myfirstpost", url:"example.com") # even if you use Post.new, will be still saved after the next << command
user.posts << post (post's user_id will be set, will hit the database immediately)
user.posts
user.posts.size
user.posts.each {|post| puts post.title}
post.user
post.user.username
Notice if you say post.user = user
The user_id on post won't be set to db
until you say post.save
(Notice always changing the right hand side)
I know if it's the left side of =, then yes, you need to run save
if it's on the right side of =, no need to run save
test??
# Subject and Page has one-to-one relation - subject has_one :page page belongs_to :subject
subject = Subject.find(1)
subject.page # returns nil
first_page = Page.new(name:"First Page", permalink:'first', position:1) # create a row, still in memory, not in DB
first_page.subject # returns nil
subject.page = first_page # the row of Page is stored to db, and returns that row object
# that row's subject_id is 1
If you deviate from convention, you have to specifiy
class Post < ActiveRecord::Base belongs_to :creator, foreign_key: 'user_id', class_name: "User"
end
then you can use .creator
post = Post.find 1
post.user error
post.creator works
post.creator.username
create_comments migration, two foreign key
create Comment model class Comment < ActiveRecord::Base belongs_to :creator, foreign_key: 'user_id', class_name: 'User' belongs_to :post end user has_many :comments post has_many :comments
object to object, id to id
comment = Comment.create(body: "my first comment", creator: User.first, post:Post.first)
or
comment = Comment.create(body: "my first comment", user_id: User.first.id, post_id:Post.first.id)
comment.creator
comment.user_id
comment.post
comment.post_id
</pre> <h3 class="text-center text-primary">Lesson 2</h3> <pre>rake db:rollback will rollback the last run migration and remove the 4th record from table that tracks migration (schema_migrations table), but will not remove the migration file
as a general rule of thumb, don't modify migration, always add new migration, this is when you work in a team and have distributed to your migration
say you rollback and change the last migration to change a table name. If other people pull it and down and run migration, nothing will happen because that migration has already been run (because they already downloaded the old version and ran migration against that)
Tha'ts why never rollback when you work in a team(after sharing the migration file with everybody already)
If you haven't pushed it, then you can rollback. No problem
Other people woudn't rollback and rerun your migration unless you email it. It's embarrasing
when you do rake db:migrate, it's only gonna run migration that hasn't been run yet
rake db:drop
rake db:create
these two commands will start your db from scratch even for sqlite
FK is always integer!!!!!!!!!!!!!!
if you use reference keyward , create index automatically
https://d3qk2ku91l34sz.cloudfront.net/blog/2015/04/13/beginners-guide-to-understanding-rails.html
in rails console,
type reload!
will reload the console
u.posts << Post.create
u.posts << Post.new
both will store to DB
= association has to run save afterwards???? strongly doubt that, need to check. That's right, you need!
comment.post = Post.first
comment.save (then the post_id on the comment will be changed)
MM Associations from GTL
# 1. has_and_belongs_to_many
# no join model
# implicit join table at db layer, still a join at table level, not much performance improvement, no need to have id because no model on top of it
# table1_name_table2_name ex: groups_users
# Problem: cannot put additional attribute(columns) on association
# 2. has_many: through
# has a join model
# can put additional attribute on association
# Problem: need a join model
# recommend always using has_many :through
=begin
in Post
has_many :post_categories
has_many :categories, through: :post_categories
has to be these two lines
order has to be like this, due to dependency
likewise in Category
has_many :post_categories
has_many :posts, through: :post_categories
so when you say
cat = Category.create(name: "Rubyists")
post.categories << cat
the join table will have the right fk updated
Create categories table
Create Category model
create post_categories table
"PostCategory".tableize
create post_category.rb
create PostCategory class
belongs_to :post
belongs_to :category
in Post
has_many :post_categories
has_many :categories, through: :post_categories
has to be these two lines
order has to be like this, due to dependency
likewise in Category
has_many :post_categories
has_many :posts, through: :post_categories
so when you say
post = Post.find 1
cat = Category.create(name: "Rubyists") or even Category.new(name: "Rubyists")
post.categories << cat
two things will happen:
1.the join table will have the right fk updated
2.the record wil be added to categories table, notice above the new and create both hit the db, because of << and on the right hand side
pc = PostCategory.create
pc.post = post
pc.save
post.reload.post_categories
pc.post
category = Category.create(name: "News")
pc = PostCategory.first
category.post_categories << pc
category.post_categories
pc.category
In Rails 4
Person.find(1) # returns the object for ID = 1
Person.find("1") # returns the object for ID = 1
@pin = current_user.pins.find_by(id: params[:id])
will set it to nil if not finding anything
@pin = current_user.pins.find(params[:id])
will throw error if not find anything
Study this documentation, and take inventory of the methods that the associations gives you. http://api.rubyonrails.org/classes/ActiveRecord/Associations/ClassMethods.html
Note: there is a lot here, and this is a difficult documentation to digest. The goal here isn't to memorize everything. The goal is to get you familiar with looking at Rails documentation. This is similar to what we did in the Ruby course, where we asked you to study the documentation for common Ruby classes, like Array and Hash.
The associations documentation shows what methods are automatically given to you when you set up a 1:M or M:M association in Rails. You may only use a few now, but over time, you'll start to get a feel for which ones are useful, and which ones you prefer to use.</pre>
<p>migration review</p>
<pre>
also talked abour rake db:rollback to rollback the last run migration and remove the version number from schema_migration table
you don't pass your data around, you pass schema around using migration
in gitignore, you see it ignores all the sqlite3 files
rake -T | grep db
rake db:drop
rake db:create (not needed for sqlith, but still can be used)
rake db:migrate
vast majority of time, change method is ok. rollback knows how to reversese it, unless you do customized things
if you need to do your own extra things, use up/down, rollback will run the down method
otherwise use change, good for 99%
don't modify your existing migration, always add new migration for 2 reasons
1: may cause non-sync between you and teammates (if you have already pushed it to github)
2: otherwise even when you are not push to git yet
you may drop some table in your local database when you rollback (like droptable migration method)
rename_column :users, :old_name, :new_name
some migration methods are destructive, causing loss of data, check DOC
lots of other ways to set up schema, migration is just one of them:
you can create the table manually(using DDL)
Antoehr way: (especially when your mgiration is not run from end to end)
Use schema.rb -< automatically generated by your migration, never modify it. schema.rb is pushed to GIT.
you can look at it and know the schema of the db
schema.rb is the master record of what the schema should look like!
rake db:drop
rake db:create
rake db:schema:load -> it will load from the schema.rb maintainied by rails
migration has more advantages, tracking historical, and also has access to your Rails codebase, like adding data, most frowned upon, in schema.rb, you cannot do that
generally don't put data manipulation code in migration
if you have data change along with schema change
use rake task for data change, cuz sometimes you have to load from schema, then u won't be able to have those data change if you put data change in migration
don't use seed for that, seed is for setting up brand new app
</pre>
<pre>
rails generate migration add_user_id_to_pins user_id:integer:index (no space)
it will generate, pretty cool
def change add_column :pins, :user_id, :integer add_index :pinx, :user_id
end
foregin key is always integer
recommend adding index on foreign key, to boost performance, but not necessary
or
rails generate migration AddNameToUsers name:string
</pre> <!------------------------------------> </div> </div> </div> </div> <footer class="text-center"> <hr> <p>Written and coded by <a href="https://www.freecodecamp.com/quincylarson" target="_blank">Dawei Dai</a>.</p> </footer>
</div>
</body>
</html>
Rails consolidation part2 - Model - Script Codes CSS Codes
body { margin-top: 60px
}
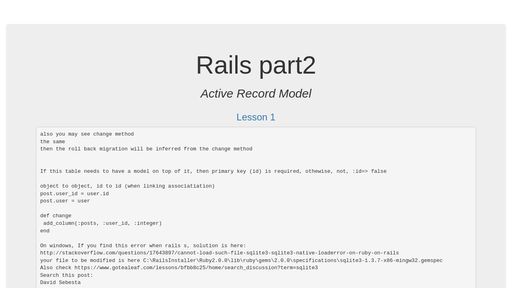
Developer | DAWEI DAI |
Username | shachopin |
Uploaded | September 29, 2022 |
Rating | 3 |
Size | 6,785 Kb |
Views | 22,264 |
Find the perfect freelance services for your business! Fiverr's mission is to change how the world works together. Fiverr connects businesses with freelancers offering digital services in 500+ categories. Find Developer!
Name | Size |
RequireJS consolidation part1 | 6,672 Kb |
Oracle JET Experiment 3 - Offcanvas | 4,424 Kb |
Rails consolidation part13 - Twitter App Clone | 6,213 Kb |
JSON and Ajax demo part1 | 3,828 Kb |
Angular JS 1.X Experiment 4 - Routing | 4,467 Kb |
Angular JS 1.X Experiment 2 - Custom Directives and templateUrl | 5,474 Kb |
Oracle JET Experiment 1 - ojMenu and delayed Ajax and launch Popup | 5,635 Kb |
Mobile Dev Part1 - Basics | 16,159 Kb |
Mobile Dev Part2 - More | 6,685 Kb |
Oracle JET Experiment 6 - Tooltip popup | 3,633 Kb |
Jasper is the AI Content Generator that helps you and your team break through creative blocks to create amazing, original content 10X faster. Discover all the ways the Jasper AI Content Platform can help streamline your creative workflows. Start For Free!
Name | Username | Size |
A Pen by panstable | Panstable | 2,940 Kb |
Kut D3 | Jellevrswk | 3,687 Kb |
Hovers with popups | Zacharyolson | 2,380 Kb |
TheCalendar.js | The-teacher | 6,330 Kb |
RAQuote | Naderk007 | 4,412 Kb |
Simple Responsive Text | Fbrz | 2,282 Kb |
V.35 The Monolith Update - Hero Release Notes | Jordan | 12,045 Kb |
Lazy Load for Background Images | The_ruther4d | 2,977 Kb |
Simple Buttons | Haydenmills | 1,750 Kb |
Slide In Panel | Vikvarg | 2,811 Kb |
Surf anonymously, prevent hackers from acquiring your IP address, send anonymous email, and encrypt your Internet connection. High speed, ultra secure, and easy to use. Instant setup. Hide Your IP Now!